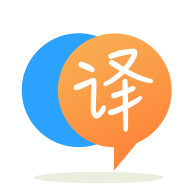
[英]How to multiply every third element of a list together if the first elements are the same?
[英]Multiply every third element of a list by two
輸入數據: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
輸出數據: [1, 2, 6, 4, 5, 12, 7, 8, 18, 10]
我讀了很多答案,建議在其中使用包含第一個元素的切片符號。 但就我而言,我應該將列表的每個第3個元素都包含在內。
有可能提高我的認識嗎?
for index in range(len(data)):
if (index + 1) % 3 == 0:
data[index] = data[index] * 2
是的,您可以使用切片符號來實現:
data[2::3] = [x*2 for x in data[2::3]]
data[2::3]
表示從元素索引2(即第三個元素)開始的每個第三個元素。 您也可以重新分配給切片,以使用這種非常簡潔的語法。
range
迭代器上有一個“ step”參數:
for index in range(2, len(data), 3):
data[index] *= 2
那會做嗎?
您的解決方案還不錯。 可以通過直接直接遍歷所需索引而不是遍歷所有索引並跳過不想要的索引來改進它:
for index in range(2, len(data), 3):
data[index] *= 2
這將產生所需的結果:
[1, 2, 6, 4, 5, 12, 7, 8, 18, 10]
您可以使用itertools和zip中的cycle將項目與其乘數配對:
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
from itertools import cycle
result = [n*m for n,m in zip(data,cycle([1,1,2]))]
# [1, 2, 6, 4, 5, 12, 7, 8, 18, 10]
或者,您可以使用enumerate()
result = [ n*max(1,i%3) for i,n in enumerate(data) ]
您可以通過將第三個參數傳遞給range()函數來對其進行組織,如下所示:
for index in range(2, len(data), 3): data[index] = data[index]*2
... 最終獲勝者是:
Calculation time in seconds and results validation test.
1.150 question_by_akrapovich
0.331 answer_by_Tom_Karzes_and_Prune
0.333 answer_2_by_Manuel_Montoya
0.373 answer_by_Blorgbeard
0.816 answer_1_by_Alain_T
2.850 answer_2_by_Alain_T
用於時間測試和結果驗證的組合代碼:
import time
def question_by_akrapovich(data):
for index in range(len(data)):
if (index + 1) % 3 == 0:
data[index] = data[index] * 2
return data
def answer_by_Tom_Karzes_and_Prune(data):
for index in range(2, len(data), 3):
data[index] *= 2
return data
def answer_by_Blorgbeard(data):
data[2::3] = [x*2 for x in data[2::3]]
return data
def answer_1_by_Alain_T(data):
from itertools import cycle
return [n * m for n, m in zip(data, cycle([1, 1, 2]))]
def answer_2_by_Alain_T(data):
return [ n*max(1,i%3) for i,n in enumerate(data) ]
def answer_2_by_Manuel_Montoya(data):
for index in range(2, len(data), 3):
data[index] = data[index]*2
return data
def test(f):
n = 10_000_000
data = [i + 1 for i in range(n)]
start_time = time.perf_counter()
data = f(data)
run_time = time.perf_counter() - start_time
if n != len(data):
print('error in list length', n, len(data))
exit(1)
for i in range(n):
j = i + 1
m = j * 2 if j % 3 == 0 else j
if data[i] != m:
print('error in data', i, m, data[i])
exit(1)
print('%.3f %s' % (run_time, f.__name__))
print('Calculation time in seconds and results validation test.')
for f in [question_by_akrapovich, answer_by_Tom_Karzes_and_Prune,
answer_2_by_Manuel_Montoya, answer_by_Blorgbeard,
answer_1_by_Alain_T, answer_2_by_Alain_T]:
test(f)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.