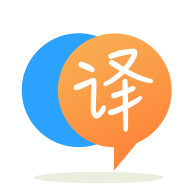
[英]How can I check whether or not Motion & Fitness is enabled on an iOS device?
[英]How can I check whether dark mode is enabled in iOS/iPadOS?
從 iOS/iPadOS 13 開始,可以使用深色用戶界面樣式,類似於 macOS Mojave 中引入的深色模式。 如何檢查用戶是否啟用了系統范圍的暗模式?
對於iOS 13 ,您可以使用此屬性檢查當前樣式是否為暗模式:
if #available(iOS 13.0, *) {
if UITraitCollection.current.userInterfaceStyle == .dark {
print("Dark mode")
}
else {
print("Light mode")
}
}
您應該檢查UITraitCollection
的userInterfaceStyle
變量,與 tvOS 和 macOS 相同。
switch traitCollection.userInterfaceStyle {
case .light: //light mode
case .dark: //dark mode
case .unspecified: //the user interface style is not specified
}
您應該使用UIView
/ UIViewController
的traitCollectionDidChange(_ previousTraitCollection: UITraitCollection?)
函數來檢測界面環境的變化(包括用戶界面風格的變化)。
來自Apple 開發者文檔:
當iOS界面環境發生變化時,系統會調用該方法。 根據您的應用程序的需要,在視圖控制器和視圖中實現此方法以響應此類更改。 例如,當 iPhone 從縱向旋轉到橫向時,您可能會調整視圖控制器子視圖的布局。 此方法的默認實現為空。
系統默認 UI 元素(例如UITabBar
或UISearchBar
)會自動適應新的用戶界面樣式。
正如 daveextreme 所提到的,當您使用overrideUserInterfaceStyle
屬性時,檢查當前視圖用戶界面樣式並不總是返回系統樣式。 在這種情況下,最好使用以下代碼:
switch UIScreen.main.traitCollection.userInterfaceStyle {
case .light: //light mode
case .dark: //dark mode
case .unspecified: //the user interface style is not specified
}
在objective-c中,你想做:
if( self.traitCollection.userInterfaceStyle == UIUserInterfaceStyleDark ){
//is dark
}else{
//is light
}
1/ 對於 UIView/UIViewController:
self.traitCollection.userInterfaceStyle == .dark
2/ 對於靜態或其他:
UITraitCollection.current.userInterfaceStyle == .dark
但:
//Never use this! You will get wrong value in app extensions (ex. ToDay widget)
UIScreen.main.traitCollection.userInterfaceStyle == .dark //WRONG!
對於斯威夫特:
if #available(iOS 12.0, *) {
switch UIScreen.main.traitCollection.userInterfaceStyle {
case .dark: // put your dark mode code here
case .light:
case .unspecified:
}
}
對於目標 C:
if (@available(iOS 12.0, *)) {
switch (UIScreen.mainScreen.traitCollection.userInterfaceStyle) {
case UIUserInterfaceStyleDark:
// put your dark mode code here
break;
case UIUserInterfaceStyleLight:
case UIUserInterfaceStyleUnspecified:
break;
default:
break;
}
}
有關更多信息,請觀看此 WWDC2019視頻
使用Environment
變量的\\.colorScheme
鍵:
struct ContentView: View {
@Environment(\.colorScheme) var colorScheme
var body: some View {
Text(colorScheme == .dark ? "In dark mode" : "In light mode")
}
}
此外,它會根據環境配色方案的變化自動更新。
要檢查當前,所有符合UITraitEnvironment
協議的對象,包括所有UIView
子類和所有UIViewConttroller
子類都可以訪問當前樣式:
myUIView.traitCollection.userInterfaceStyle == .dark
myUIViewController.traitCollection.userInterfaceStyle == .dark
要檢測樣式的實時變化,這里是完整的詳細答案
目標 C
要通過控制中心檢測何時啟用或禁用暗模式,請使用“appDidBecomeActive”通知,該通知將在您返回應用程序時觸發。
//----------------------------------------------------------------------------
// viewWillAppear
//----------------------------------------------------------------------------
- (void)viewWillAppear {
[super viewWillAppear];
[[NSNotificationCenter defaultCenter]addObserver:self
selector:@selector(appDidBecomeActive:)
name:UIApplicationDidBecomeActiveNotification
object:nil];
}
完成后不要忘記將其刪除:
//------------------------------------------------------------------------------------
// viewWillDisappear
//------------------------------------------------------------------------------------
- (void)viewWillDisappear:(BOOL)animated
{
[super viewWillDisappear:animated];
[[NSNotificationCenter defaultCenter] removeObserver:self
name:UIApplicationDidBecomeActiveNotification
object:nil];
}
在暗模式更改時執行您需要的操作:
//----------------------------------------------------------------------------
// appDidBecomeActive
//----------------------------------------------------------------------------
-(void)appDidBecomeActive:(NSNotification*)note {
if (@available(iOS 13.0, *)) {
if( self.traitCollection.userInterfaceStyle == UIUserInterfaceStyleDark ){
//dark mode
}
else {
//not dark mode
}
}
else {
//fall back for older versions
}
}
為 write 方法創建一個類函數 1 次並在任何你想要的地方使用
func isDarkMode() -> Bool{
if #available(iOS 12.0, *) {
if UIScreen.main.traitCollection.userInterfaceStyle == .dark {
return true
} else {
return false
}
} else {
return false
}
}
下面的 Helper 方法適用於任何 iOS 版本:
var isDarkMode: Bool {
guard #available(iOS 12.0, *) else {
return false
}
return UIScreen.main.traitCollection.userInterfaceStyle == .dark
}
用法:
view.backgroundColor = isDarkMode ? .black : .white
檢測變化的最佳點是 UIView/UIViewController 的 traitCollectionDidChange(_ previousTraitCollection: UITraitCollection?) 函數。
override func traitCollectionDidChange(_ previousTraitCollection: UITraitCollection?) {
super.traitCollectionDidChange(previousTraitCollection)
let userInterfaceStyle = traitCollection.userInterfaceStyle // Either .unspecified, .light, or .dark
// Update your user interface based on the appearance
}
通過覆蓋視圖控制器上的 traitCollectionDidChange 來檢測外觀變化是微不足道的。 然后,只需訪問視圖控制器的 traitCollection.userInterfaceStyle。
但是,重要的是要記住 traitCollectionDidChange 可能會被其他特征更改調用,例如設備旋轉。 您可以使用這種新方法檢查當前外觀是否不同:
override func traitCollectionDidChange(_ previousTraitCollection: UITraitCollection?) {
super.traitCollectionDidChange(previousTraitCollection)
let hasUserInterfaceStyleChanged = previousTraitCollection.hasDifferentColorAppearance(comparedTo: traitCollection) // Bool
// Update your user interface based on the appearance
}
你可以使用這個擴展:
import UIKit
extension UIApplication {
@available(iOS 13.0, *)
var userInterfaceStyle: UIUserInterfaceStyle? {
return self.keyWindow?.traitCollection.userInterfaceStyle
}
}
@available(iOS 13.0, *)
func setSystemTheme() {
switch UIApplication.shared.userInterfaceStyle {
case .dark?:
currentTheme = .dark
case .light?:
currentTheme = .light
default:
break
}
}
var isDarkMode: Bool {
guard #available(iOS 12.0, *) else {
return false
}
let window = (UIApplication.shared.delegate as? AppDelegate)?.window
return window?.traitCollection.userInterfaceStyle == .dark
}
如果您沒有在 AppDelegate 中使用 window,請從 SceneDelegate 調用 window
它類似於上面的大多數答案,但是當我們使用
window?.overrideUserInterfaceStyle = .dark
可以稱為
isDarkMode ? .black : .white
也許一些不錯的擴展?
public extension UIViewController {
@available(iOS 12.0, *)
public var isDarkMode: Bool { traitCollection.userInterfaceStyle == .dark }
}
您可以使用此方法 Swift 5 輕松檢測暗模式或亮模式
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
if traitCollection.userInterfaceStyle == .light {
print("Light mode")
} else {
print("Dark mode")
}}
您可以使用以下代碼檢查項目中的亮模式或暗模式:
func viewDidLoad() {
super.viewDidLoad()
switch traitCollection.userInterfaceStyle {
case .light, .unspecified:
// light mode detected
case .dark:
// dark mode detected
}
}
您還可以檢查界面風格的變化:
override func traitCollectionDidChange(_ previousTraitCollection: UITraitCollection?) {
super.traitCollectionDidChange(previousTraitCollection)
let userInterfaceStyle = traitCollection.userInterfaceStyle // Either .unspecified, .light, or .dark
// Update your user interface based on the appearance
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.