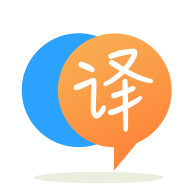
[英]Mock call to method from another method within same controller in Laravel
[英]Call method of another controller in laravel
我必須調用另一個控制器的方法。
我使用以下代碼進行呼叫。
app('App\Http\Controllers\ApiUserController')->getList();
一切正常。
但是我想嘗試使用use函數,這樣我就不必重復所有行
use App\Http\Controllers\ApiUserController;
class MyMethods
{
public function index()
{
app('ApiUserController')->getList()
我在這里弄錯了嗎?
無需使用app
功能,您將需要像這樣通過OOP進行操作:
use App\Http\Controllers\ApiUserController;
class MyMethods
{
public function index()
{
$apiUserController = new ApiUserController();
$apiUserController->getList();
但是,正如許多人在這里提到的那樣,從另一個控制器中調用一個控制器的方法實際上並不是最佳實踐。
因此,如果我在您的位置,我將創建一個幫助器,在config
注冊其別名,然后使用該幫助器在兩個位置獲取列表。
希望對您有所幫助
從其他控制器或其他對象調用控制器不是一個好習慣。 這是一篇很好的文章,解釋原因。 同樣, “胖”控制器比“瘦”控制器更不受歡迎。
您應該使用通用邏輯定義服務層對象並使用它。 創建服務對象並向服務提供者之一注冊。
namespace App\Providers;
use Illuminate\Support\ServiceProvider;
use App\Services\YourUserService;
class AppServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->singleton(YourUserService::class);
}
}
之后,您可以使用DI風格的服務。
use App\Services\YourUserService;
class MyMethods
{
protected $userService;
public function __construct(YourUserService $userService)
{
$this->userService = $userService;
}
public function index()
{
$this->userService->foo();
}
}
我同意學習者上面給出的答案,但是,就代碼組織和可測試性而言,我不建議這樣做。
查看代碼,我可以看到您需要獲取用戶列表,這就是為什么必須從另一個控制器調用api用戶控制器的原因。 但是,您可以輕松地將邏輯提取到服務甚至特征中。
如果您要使用特質,則可以執行以下操作,
trait ApiUser {
public function getList()
{
// get the list for users from api
}
}
//Then you can simply use this trait any where you want,
class SomeController
{
// correct namespace for ApiUser trait
use ApiUser;
}
另一種方式,我喜歡根據情況反復使用; 堅持編碼的原則是接口不要實現。 那將是一些類似的事情。
interface ApiUserInterface
{
public function getList();
}
class ApiUser implements ApiUserInterface
{
public function getList()
{
// logic to get users from api
}
}
確保當應用程序需要接口時,它知道在哪里可以找到其實現。 如果使用Laravel,則可以將接口注冊到AppServiceProvider中的類
完成后,您可以在需要合同的任何地方使用此服務。
class OneController
{
protected $apiUserContract;
public function __construct(ApiUserInterface $apiUserContract)
{
$this->apiUserContract = $apiUserContract;
}
public function index()
{
// You can retrieve the list of the contract
$this->apiUserContract->getList();
}
}
// you could also just typehint the contact in method without requiring
// it in constructor and it will get resolved out of IOC i.e. container
class AnotherController
{
public function index(ApiUserInterface $apiUserContract)
{
// You can retrieve the list of the contract
$apiUserContract->getList();
}
}
讓我知道您是否需要進一步的解釋,希望對您有所幫助
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.