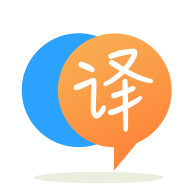
[英]How can i combine an array of objects into another array of objects while also combining the common keys
[英]How can I filter through an array of objects while also comparing another object?
我正在嘗試搜索汽車列表(包含品牌,型號,價格和其他值)並將其與我在表單上搜索的汽車進行比較。
我知道怎么做才能完全比較兩輛車,但我不知道如何過濾它,例如,我只想通過make搜索並顯示所有車輛的相同品牌(但不用擔心)其他值因為沒有其他選擇)。
我嘗試過使用lowdash ._isEqual()
,如果這兩個對象完全相同的話就可以使用。 但是,如果我只是想通過某個品牌,或者某個年份,或類似的東西來搜索,我不知道該怎么做。
app.component.ts
export class AppComponent {
cars:Car[];
title = 'car-dealership';
constructor(private carsService:CarsService) {}
searchCars(car:Car) {
this.carsService.getAllCars().subscribe(resp => {
this.cars = resp;
this.cars.filter(c => {
//do something
})
})
}
}
輸入form.component.ts
export class InputFormComponent implements OnInit {
@Output() searchCars: EventEmitter<any> = new EventEmitter();
make:string;
year:number;
color:string;
sunRoof = false;
fourWheel = false;
lowMiles = false;
powerWindows = false;
navigation = false;
heatedSeats = false;
price:number;
constructor() { }
ngOnInit() {
}
onSubmit() {
const selectedCar = {
color: this.color,
hasHeatedSeats: this.heatedSeats,
hasLowMiles: this.lowMiles,
hasNavigation: this.navigation,
hasPowerWindows: this.powerWindows,
hasSunroof: this.sunRoof,
isFourWheelDrive: this.fourWheel,
make: this.make,
price: Number(this.price),
year: Number(this.year)
}
console.log('form submitted with:', selectedCar);
this.searchCars.emit(selectedCar);
}
}
cars.service.ts
export class CarsService {
constructor() {}
getAllCars() {
return of(Cars);
}
}
如果要包含該項,則只需要返回filter
方法 - 如果它返回true
(或任何其他“truthy”值),則包含該項,否則排除。
searchCars(car:Car) {
this.carsService.getAllCars().subscribe(resp => {
this.cars = resp.filter(c => {
return c.make === this.make; // Or whatever complicated logic you want to use
});
});
}
根據您的評論,我了解您需要檢查兩件事:1)是否應使用此標准; 2)項目是否符合條件。
那么,讓我們編碼吧!
searchCars(car:Car) {
this.carsService.getAllCars().subscribe(resp => {
this.cars = resp.filter(c => {
// First, check if the criteria should be used
if (this.make && this.make.length > 0) {
return c.make === this.make;
}
// Return a default value if the criteria isn't being used
return true;
});
});
}
太棒了。 但如果需要匹配零,一或兩個條件呢?
好吧,我們將使用一種稱為“早期回歸”的“技巧”或“模式”。 我們將檢查它是否與EACH條件不匹配並返回false,而不是檢查它是否匹配所有條件並返回true。
searchCars(car:Car) {
this.carsService.getAllCars().subscribe(resp => {
this.cars = resp.filter(c => {
// First, check if the criteria should be used
if (this.make && this.make.length > 0) {
// Then, check if it doesn't match
if (c.make !== this.make) {
// Early return, it doesn't matter what the other checks say
// we know this shouldn't be included.
return false;
}
}
// First, check if the criteria should be used
if (this.color && this.color.length > 0) {
// Then, check if it doesn't match
if (c.color !== this.color) {
// Early return, it doesn't matter what the other checks say
// we know this shouldn't be included.
return false;
}
}
// Repeat for all conditions you need to look at
// Return a default value. If the code gets here it means either:
// 1) no conditions are applied
// 2) it matches all relevant conditions
return true;
});
});
}
試試下面的內容
this.cars.filter(c => {
return c.make == this.make // You can have multiple conditions here
})
Filter方法返回滿足條件的新數組中的項。
您只需告訴過濾器函數要在數組中檢查哪種條件,它將返回條件變為true的每個元素,例如,如果要按make過濾:
this.cars.filter(c => {
c.make === 'SOME_MAKE'
});
您還可以添加多個過濾器:
this.cars.filter(c => {
c.make === 'SOME_MAKE' && c.year === 2015 // Filtering by make and year
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.