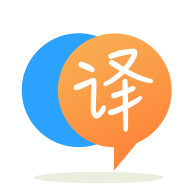
[英]How to filter array by comparing two arrays of objects with different elements in their objects?
[英]Filter and delete items by comparing two arrays of objects with different elements
我有兩個數組
arr1 = [{path: "path1"}, {path: "path2"}];
arr2 = [{path: "path1"}];
首先,我想通過比較兩個數組來查找從arr1中刪除的元素(在本例中為其path1對象),並操縱第一個數組或通過刪除該項覆蓋它。 在這種情況下,預期的數組是
expArray = [{path: "path2"}]
我試過使用array.filter
方法。
var filteredElements = arr1 .filter(function(obj) {
return !arr2 .some(function(obj2) {
return obj.Path === obj2.Path;
});
});
但是它僅提供了不同的元素列表,但不支持從數組中刪除該元素。 有沒有辦法使用下划線或傳統方式做到這一點?
您有Path
vs path
的錯字。 該代碼適用於此更改。
var arr1 = [{ path: "path1" }, { path: "path2" }], arr2 = [{ path: "path1" }], filteredElements = arr1.filter(function(obj) { return !arr2.some(function(obj2) { return obj.path === obj2.path; // ^ ^ }); }); console.log(filteredElements);
如果您想對arr1
進行突變,則可以拼接不需要的元素,並且由於這會改變長度,因此可以從末尾進行迭代。
var arr1 = [{ path: "path1" }, { path: "path2" }], arr2 = [{ path: "path1" }], i = arr1.length; while (i--) { if (arr2.some(({ path }) => arr1[i].path === path)) { arr1.splice(i, 1); } } console.log(arr1);
您可以使用Array#splice
從現有陣列中刪除元素。
let arr1 = [{ path: "path1" }, { path: "path2"}]; let arr2 = [{ path: "path1" }]; // get length of array for iteration let l = arr1.length; // iterate from end to start to avoid skipping index after removing an element while (l--) { // check elemnt present in the array if (arr2.some(obj => obj.path === arr1[l].path)) // remove the element arr1.splice(l, 1); } console.log(arr1);
我相信您只是將path
屬性鍵入為Path
並且它返回一個空數組。
工作的代碼:
var arr1 = [{path: "path1"}, {path: "path2"}];
var arr2 = [{path: "path1"}];
var filteredElements = arr1.filter(function(obj) {
return !arr2.some(function(obj2) {
return obj.path === obj2.path;
});
});
如果要覆蓋第一個數組,只需用arr1
替換filteredElements
。
你可以用地圖
let arr1 = [{ path: "path1" }, { path: "path2"}]; const arr2 = [{ path: "path1" }]; const itemsToRemove = arr2.map(a => a.path); arr1 = arr1.filter(a => !itemsToRemove.includes(a.path)); console.log(arr1);
過濾並重新分配數組值。
處理此問題的另一種方法是,從篩選數據的過程中分離出用於測試兩項是否相等的邏輯。 在這里, keep
是一個非常簡單的高階函數。 我們傳遞給它一個函數,該函數報告兩個對象是否具有相同的path
屬性,並在兩個數組上返回一個函數。
然后,我們簡單地使用該功能。
const keep = (eq) => (xs, ys) => xs.filter ( x => ys .some ( y => eq(x, y) ) ) const keepByPath = keep ( (x, y) => x.path == y.path ) const arr1 = [{ path: "path1", foo: 1 }, { path: "path2", foo: 2 }] const arr2 = [{ path: "path1", bar: 1 }] console.log ( keepByPath (arr1, arr2) //~> [{ path: "path1", foo: 1 }] )
請注意,我添加了一些屬性。 這些證明我們只保留第一個數組中的項目。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.