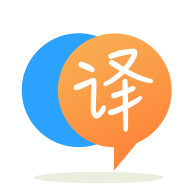
[英]Using Jasmine to spy on document.createElement throws error from angular mock
[英]Spy on or mock document.createElement using Jest
我需要測試一些在文檔上創建一個元素的代碼,該元素分配了一個 URL,然后單擊。
我正在使用 Jest。
const link = document.createElement('a')
我放棄了模擬文檔的嘗試,因為我看不到一種簡單的方法來做到這一點,盡管模擬點擊會很好。
我需要知道createElement
發生了,所以我決定創建一個間諜:
jest.spyOn(document, 'createElement')
出於某種原因,間諜破壞了測試,我得到了與嘗試模擬文檔時相同的錯誤:
Error: TypeError: Cannot set property 'href' of undefined
document.createElement
下面的代碼是:
link.href = url
有任何想法嗎?
這是解決方案,我使用 node.js 運行時環境進行演示:
class Link {
private name: string;
private _href: string = '';
constructor(name) {
this.name = name;
}
get href() {
return this._href;
}
set href(url) {
this._href = url;
}
}
const document = {
createElement(name) {
return new Link(name);
}
};
function createLink(url) {
const link = document.createElement('a');
link.href = url;
return link;
}
export { createLink, document, Link };
單元測試:
import { createLink, document, Link } from './';
describe('createLink', () => {
it('t1', () => {
const url = 'https://github.com/mrdulin';
jest.spyOn(document, 'createElement').mockReturnValueOnce(new Link('mock link'));
const link = createLink(url);
expect(link).toBeInstanceOf(Link);
expect(link.href).toBe(url);
});
});
PASS src/stackoverflow/57088724/index.spec.ts
createLink
✓ t1 (6ms)
Test Suites: 1 passed, 1 total
Tests: 1 passed, 1 total
Snapshots: 0 total
Time: 2.451s, estimated 3s
在從事我最新的開源項目 ( TabMerger ) 時,我想出了一個更簡單的解決方案,並認為它更直觀,可以幫助正在苦苦掙扎的人。 此外,它可以很容易地根據您的需要進行調整。
// function to test
function exportJSON() {
chrome.storage.local.get("groups", (local) => {
var group_blocks = local.groups;
chrome.storage.sync.get("settings", (sync) => {
group_blocks["settings"] = sync.settings;
var dataStr = "data:text/json;charset=utf-8," + encodeURIComponent(JSON.stringify(group_blocks, null, 2));
// want to test all of below also!!
var anchor = document.createElement("a");
anchor.setAttribute("href", dataStr);
anchor.setAttribute("download", AppHelper.outputFileName() + ".json");
anchor.click();
anchor.remove();
});
});
}
為了測試,我簡單地模擬了document.createElement
函數的返回值以返回一個可以被監視的錨對象:
describe("exportJSON", () => {
it("correctly exports a JSON file of the current configuration", () => {
// ARRANGE
// creates the anchor tag as an object
function makeAnchor(target) {
return {
target,
setAttribute: jest.fn((key, value) => (target[key] = value)),
click: jest.fn(),
remove: jest.fn(),
};
}
// spy on the document.createElement and mock its return
// also spy on each anchor function you want to test
var anchor = makeAnchor({ href: "#", download: "" });
var createElementMock = jest.spyOn(document, "createElement").mockReturnValue(anchor);
var setAttributeSpy = jest.spyOn(anchor, "setAttribute");
var clickSpy = jest.spyOn(anchor, "click");
var removeSpy = jest.spyOn(anchor, "remove");
// create expectations
var group_blocks = JSON.parse(localStorage.getItem("groups"));
group_blocks["settings"] = JSON.parse(sessionStorage.getItem("settings"));
const dataStr = "data:text/json;charset=utf-8," + encodeURIComponent(JSON.stringify(group_blocks, null, 2));
jest.clearAllMocks(); // start with clean mocks/spies before call is made
// ACT
AppFunc.exportJSON();
// ASSERT
// chromeLocalGetSpy, chromeSyncGetSpy defined elsewhere as
// jest.spyOn(chrome.storage.local, "get") & jest.spyOn(chrome.storage.sync, "get"), respectively.
expect(chromeLocalGetSpy).toHaveBeenCalledTimes(1);
expect(chromeLocalGetSpy).toHaveBeenCalledWith("groups", anything);
expect(chromeSyncGetSpy).toHaveBeenCalledTimes(1);
expect(chromeSyncGetSpy).toHaveBeenCalledWith("settings", anything);
expect(document.createElement).toHaveBeenCalledTimes(1);
expect(document.createElement).toHaveBeenCalledWith("a");
expect(setAttributeSpy).toHaveBeenCalledTimes(2);
expect(setAttributeSpy).toHaveBeenNthCalledWith(1, "href", dataStr);
expect(setAttributeSpy).toHaveBeenNthCalledWith(2, "download", AppHelper.outputFileName() + ".json");
expect(clickSpy).toHaveBeenCalledTimes(1);
expect(removeSpy).toHaveBeenCalledTimes(1);
createElementMock.mockRestore(); // restore document.createElement so that it is unchanged in other tests
});
});
exportJSON
√ correctly exports a JSON file of the current configuration (48 ms)
完整代碼可在我的GitHub 上找到
在<rootDir>/tests/__mocks__/chromeMock.js
文件夾中,您可以看到localStorage
和sessionStorage
分別用於模擬chrome.storage.local
和chrome.storage.sync
API。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.