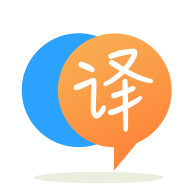
[英]React useEffect dependencies for subscribe/unsubscribe with eslint exhaustive-deps
[英]Warning for 'exhaustive-deps' keeps asking for the full 'props' object instead of allowing single 'props' methods as dependencies
這個問題與eslint-plugin-react-hooks
當我在 CodeSanbox 中使用 React Sandbox 時,我可以使用 props 對象的單個屬性作為 useEffect 鈎子的依賴項:
示例 1:
useEffect(()=>{
console.log('Running useEffect...');
console.log(typeof(props.myProp)); // USING ONLY 'myProp' PROPERTY
},[ ]); // EMPTY ARRAY
示例 1 在 CodeSandbox 環境中給了我以下警告:
React Hook useEffect 缺少依賴項:'props.myProp'。 包括它或刪除依賴項數組。 (react-hooks/exhaustive-deps) eslint
如果我將[props.myProp]
添加到數組中,警告就會消失。
但是在我的 VSCode 本地環境中的相同示例 1,我收到以下警告:
React Hook useEffect 缺少一個依賴項:'props'。 包括它或刪除依賴項數組。 然而,'props' 會在任何 props 改變時改變,所以首選的解決方法是在 useEffect 調用之外解構 'props' 對象,並在 useEffect.eslint(react-hooks/exhaustive-deps) 中引用那些特定的 props
是問我缺少完整的props
對象。 如果我將[props.myProp]
添加到數組中,警告不會消失。 盡管代碼按預期工作。
我希望我會在 VSCode 的本地環境中獲得與在 CodeSandbox 上獲得的行為相同的行為。
會發生什么? 我可以在eslint-plugin-react-hooks
更改任何配置嗎?
套餐
開發:
"eslint": "^5.10.0",
"eslint-plugin-react": "^7.11.1",
"eslint-plugin-react-hooks": "^1.6.1",
常規的
"react": "^16.8.6",
"react-dom": "^16.8.6",
.eslintrc.json
{
"root" :true,
"env": {
"browser": true,
"commonjs": true,
"es6": true,
"node": true
},
"extends": [
"eslint:recommended",
"plugin:react/recommended",
"plugin:import/errors"
],
"parser":"babel-eslint",
"parserOptions": {
"ecmaVersion": 8,
"sourceType": "module",
"ecmaFeatures": {
"jsx":true
}
},
"plugins":[
"react",
"react-hooks"
],
"rules": {
"react/prop-types": 0,
"semi": "error",
"no-console": 0,
"react-hooks/rules-of-hooks": "error",
"react-hooks/exhaustive-deps": "warn"
},
"settings": {
"import/resolver" : {
"alias" : {
"map" : [
["@components","./src/components"],
["@constants","./src/constants"],
["@helpers","./src/helpers"]
],
"extensions": [".js"]
}
}
}
}
這個問題的答案在這里有些回答: https : //github.com/facebook/react/issues/16265#issuecomment-517518539
該插件之所以看到它不同是因為這樣做
props.whatever()
你實際上是通過props
本身作為this
值whatever
。 所以從技術上講,它會看到陳舊的道具。通過在調用之前讀取函數,您可以避免這個問題。
雖然答案說解構就是答案,但有一個小小的警告。 除了解決警告(99% 的情況)或破壞您的代碼之外,解構或重新分配將沒有任何效果。 如果您解構或重新分配,該函數將沒有this
,如果這不是問題,則警告毫無意義。 這是一個人為案例的演示,其中任何一個都很重要。
function bark() {
console.log(`${this.name} barked.`);
}
const mac = {
name: "mac",
bark
};
const cheese = {
name: "cheese",
bark
};
const DogBark = props => {
useEffect(() => props.dog.bark(), [props.dog.bark]);
return null;
};
const Dog = () => {
const [dog, setDog] = React.useState(mac);
useEffect(() => setDog(cheese), []);
// will cheese bark?
return (
<DogBark dog={dog} />
);
};
奶酪不會吠叫。 當您開始使用useCallback
時,問題變得更糟:
const DogBarkByCallback = props => {
const bark = React.useCallback(() => props.dog.bark(), [props.dog.bark]);
// every dog should bark, and if the definition of
// bark changes even though the dog is the same, it should bark again
useEffect(() => bark(), [props.dog, bark]);
return null;
};
在這種情況下,mac 吠叫兩次,因為回調沒有改變。 因此,解決方案是確保[props.dog]
位於您的依賴項數組中,正如警告所建議的那樣,或者知道您不需要this
並解構或重新分配值以規避警告。
在控制台中演示: https : //codesandbox.io/s/exhaustive-deps-fn-mrdld
您是否考慮過破壞財產?
const { myProp } = props
useEffect(()=>{
console.log('Running useEffect...');
console.log(typeof(myProp)); // USING ONLY 'myProp' PROPERTY
},[myProp]);
我了解發生了什么事。 這不是一個錯誤(我認為)。
這段代碼:
useEffect(()=>{
function someFunction() {
props.whatever(); // CALLING A FUNCTION FROM PROPS
}
},[ ]);
結果如下:
React Hook useEffect缺少依賴項:“ props”。 包括它或刪除依賴項數組。 但是,“ props”將在任何 prop發生更改時發生更改,因此首選解決方法是在useEffect調用之外對“ props”對象進行解構,並引用useEffect內部的那些特定prop。 (反應鈎/窮舉)
這段代碼:
useEffect(()=>{
function someFunction() {
props.whatever; // ACCESSING A PROPERTY FROM PROPS
}
},[]);
結果如下:
React Hook useEffect缺少依賴項:“ props.whatever”。 包括它或刪除依賴項數組。 (反應鈎/窮舉)
我不確定為什么,但是當您從props
調用方法或從props
property
時,插件對它的看法有所不同。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.