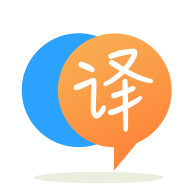
[英]What is the way to set default values on keys in lists when unmarshalling Yaml in Go?
[英]What's a better way to create go structs for unmarshalling the following yaml file?
我正在解組 yaml 文件snmp.yml 。 我想知道我是否可以獲得關於創建更好結構的建議。 這就是我現在所擁有的,但我猜我為 Metric 創建的結構很好,但 SNMPyaml 需要更好的重組才能完全能夠正確使用未編組的數據。
非常感謝這里的任何建議/反饋。 先感謝您!
package system
import (
"fmt"
"io/ioutil"
"log"
"path/filepath"
y "gopkg.in/yaml.v2"
)
//SNMPyaml struct
type SNMPyaml struct {
Metrics Metric `yaml:"metrics"`
}
//Metric exportable
type Metric struct {
Name string `yaml:"name,omitempty"`
Oid string `yaml:"oid"`
Type string `yaml:"type"`
Help string `yaml:"help,omitempty"`
}
// Yamlparser
func Yamlparser() {
// Read the snmp.yml file
absPath, _ := filepath.Abs("./app/snmp.yml")
yamlFile, yamlerror := ioutil.ReadFile(absPath)
if yamlerror != nil {
log.Fatalf("ioutil err: %v", yamlerror)
}
//Unmarshall
var c SNMPyaml
err := y.Unmarshal(yamlFile, &c)
if err != nil {
log.Fatal(err)
}
fmt.Print(c)
}
metrics:
- name: sysStatClientCurConns
oid: 1.3.6.1.4.1.3375.2.1.1.2.1.8
type: gauge
indexes:
- labelname: sysStatClientCurConns
type: gauge
- name: sysClientsslStatCurConns
oid: 1.3.6.1.4.1.3375.2.1.1.2.9.2
type: gauge
indexes:
- labelname: sysClientsslStatCurConns
type: gauge
- name: sysClientSslStatTotNativeConns
oid: 1.3.6.1.4.1.3375.2.1.1.2.9.6
type: gauge
indexes:
- labelname: sysClientSslStatTotNativeConns
type: gauge
我為此得到的錯誤是:
2019/07/31 23:25:58 yaml: line 25: mapping values are not allowed in this context
exit status 1
在您的輸入metrics
是一個序列(列表),因此您不能將其編組為單個Metric
。 使用切片: []Metric
:
type SNMPyaml struct {
Metrics []Metric `yaml:"metrics"`
}
還有一個indexes
字段,另一個序列,您的Metric
結構中沒有相應的字段,並且您有一個不必要的Help
(至少在您提供的輸入中沒有這樣的字段):
type Metric struct {
Name string `yaml:"name,omitempty"`
Oid string `yaml:"oid"`
Type string `yaml:"type"`
Indexes []Index `yaml:"indexes"`
}
可以使用此結構對索引進行建模的位置:
type Index struct {
LabelName string `yaml:"labelname"`
Type string `yaml:"type"`
}
運行這些更改后,在Go Playground上嘗試,並產生以下輸出:
{[{sysStatClientCurConns 1.3.6.1.4.1.3375.2.1.1.2.1.8 儀表 [{sysStatClientCurConns Gauge}]} {sysClientsslStatCurConns 1.3.6.1.4.1.3375.2.1.1.2.9.2 儀表{sysClientslStatCurConns 1.3375.2.1.1.2.9.2 儀表}{sysClientStatConnslstslstslstslstat}{syslstslstnslstslstslstats 1.3.6.1.4.1.3375.2.1.1.2.9.6規[{sysClientSslStatTotNativeConns規}]}]}
另請注意,有一個在線 YAML-to-Go 轉換器,您可以在其中輸入 YAML 源,它會生成對您的輸入進行建模的 Go 數據結構: https : //mengzhuo.github.io/yaml-to-go/
生成的代碼使用未命名的結構(如果您必須為其創建值,這會很痛苦),但這是一個很好的起點,您可以輕松地將它們重構為命名類型。 它從您的 YAML 輸入生成以下模型:
type AutoGenerated struct {
Metrics []struct {
Name string `yaml:"name"`
Oid string `yaml:"oid"`
Type string `yaml:"type"`
Indexes []struct {
Labelname string `yaml:"labelname"`
Type string `yaml:"type"`
} `yaml:"indexes"`
} `yaml:"metrics"`
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.