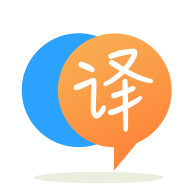
[英]Vue&TypeScript: how to avoid error TS2345 when import implemented in TypeScript component outside of project directory?
[英]Vue & Typescript: How to avoid TS error when accessing child component's methods
我創建了一個自定義的基於類的 vue 組件,我正在嘗試從父組件訪問它的方法和/或計算屬性。 Vue Docs 中有一個示例解釋了我正在嘗試做的事情( https://v2.vuejs.org/v2/guide/components-edge-cases.html#Accessing-Child-Component-Instances-amp-子元素)。 所以基本上就是這樣
class ParentComponent extends Vue {
someMethod() {
(this.$refs.myChildRef as ChildComponent).focus()
}
}
class ChildComponent extends Vue {
focus() {
// do something
}
}
現在,這會導致 TS 錯誤:“TS2339:屬性 'focus' 在類型 'Vue' 上不存在”
顯然,打字稿沒有看到我的 ChildComponent 有其他方法。
該代碼在運行時仍然有效,所以它似乎只是一個打字稿問題。
有誰知道如何解決這個問題?
選項1:忽略它
//@ts-ignore
選項 2:鍵入任何
const child: any = this.$refs.myChildRef;
child.focus();
選項 3:接口,如@LLai 所述
interface ComponentInterface {
focus: () => void
}
選項 4:合並類型
正如@LaLai 所說的那樣
(this.$refs.myChildRef as ChildComponent & { focus: () => void }).focus()
或者如果您更頻繁地需要它
Class ParentComponent extends Vue {
$refs: Vue["$refs"] & {
myChildRef: { focus: () => void }
};
}
選項 5:來自vue-property-decorator
@Ref()
裝飾器
Class ParentComponent extends Vue {
@Ref()
childComponent: ChildComponent
}
一種解決方案是在您的子組件上實現一個接口。
interface ComponentInterface {
focus: () => void
}
class ChildComponent extends Vue implements ComponentInterface {
focus () {
// do something
}
}
另一種選擇是合並類型
class ParentComponent extends Vue {
someMethod() {
(this.$refs.myChildRef as ChildComponent & { focus: () => void }).focus()
}
}
但是,如果您在子組件之外大量調用焦點,這可能會變得重復。 我更喜歡選項1。
我們使用 typescript 構建的組件也有類似的問題。 我們現在使用的解決方案只是在每個問題之前添加一個 ts-ignore 注釋。 這不是最好的方法,但它有效。
//@ts-ignore
試試看
我不想兩次聲明方法名稱,所以我使用這種方式:
在 ChildComponent.ts 中
import Vue from 'vue'
export default class ChildComponent extends Vue.extend({
methods: {
focus() {}
}
}){}
在 ChildComponent.vue 中
<template>...</template>
<script lang="ts">
import ChildComponent from './ChildComponent'
export default ChildComponent;
</script>
在父組件中
import Vue from 'vue'
import ChildComponent from './ChildComponent.vue'
import ChildComponentClass from './ChildComponent'
export default class ParentComponent extends Vue.extend({
components: {ChildComponent},
methods: {
someMethod() {
(<ChildComponentsClass>this.$refs.child).focus();
}
}
}){}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.