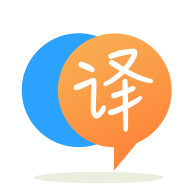
[英]How to convert this curl request to Http post request for file upload in java?
[英]How to transform a curl command to HTTP POST request using java
我想在java
使用HTTP
POST
請求運行這個特定的 curl 命令
curl --location --request POST "http://106.51.58.118:5000/compare_faces?face_det=1" \
--header "user_id: myid" \
--header "user_key: thekey" \
--form "img_1=https://cdn.dnaindia.com/sites/default/files/styles/full/public/2018/03/08/658858-577200-katrina-kaif-052217.jpg" \
--form "img_2=https://cdn.somethinghaute.com/wp-content/uploads/2018/07/katrina-kaif.jpg"
我只知道如何通過傳遞JSON
對象來發出簡單的POST
請求,但我從未嘗試過基於上述curl
命令進行POST
。
這是我基於此curl
命令制作的POST
示例:
curl -X POST TheUrl/sendEmail
-H 'Accept: application/json' -H 'Content-Type: application/json'
-d '{"emailFrom": "smth@domain.com", "emailTo":
["smth@gmail.com"], "emailSubject": "Test email", "emailBody":
"708568", "generateQRcode": true}' -k
這是我如何使用java
做到的
public void sendEmail(String url) {
try {
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
//add reuqest header
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json; utf-8");
con.setRequestProperty("Accept", "application/json");
con.setDoOutput(true);
// Send post request
JSONObject test = new JSONObject();
test.put("emailFrom", emailFrom);
test.put("emailTo", emailTo);
test.put("emailSubject", emailSubject);
test.put("emailBody", emailBody);
test.put("generateQRcode", generateQRcode);
String jsonInputString = test.toString();
System.out.println(jsonInputString);
System.out.println("Email Response:" + returnResponse(con, jsonInputString));
} catch (Exception e) {
System.out.println(e);
}
System.out.println("Mail sent");
}
public String returnResponse(HttpURLConnection con, String jsonInputString) {
try (OutputStream os = con.getOutputStream()) {
byte[] input = jsonInputString.getBytes("utf-8");
os.write(input, 0, input.length);
} catch (Exception e) {
System.out.println(e);
}
try (BufferedReader br = new BufferedReader(new InputStreamReader(con.getInputStream(), "utf-8"))) {
StringBuilder response = new StringBuilder();
String responseLine = null;
while ((responseLine = br.readLine()) != null) {
response.append(responseLine.trim());
}
return response.toString();
} catch (Exception e) {
System.out.println("Couldnt read response from URL");
System.out.println(e);
return null;
}
}
我找到了這個有用的鏈接,但我無法真正理解如何在我的示例中使用它。
和我的例子有什么不同嗎? 如果是我怎樣才能POST
以下數據?
注意:所需數據
HEADERS:
user_id myid
user_key mykey
PARAMS:
face_det 1
boxes 120,150,200,250 (this is optional)
BODY:
img_1
multipart/base64 encoded image or remote url of image
img_2
multipart/base64 encoded image or remote url of image
這是API的完整文檔
您的 HttpURLConnection 需要四件事:
application/x-www-form-urlencoded
(或multipart/form-data
)作為其正文的內容類型。 這是通過使用setRequestProperty
方法設置Content-Type
標頭來完成的,就像其他標頭一樣。這四個步驟看起來像這樣:
String img1 = "https://cdn.dnaindia.com/sites/default/files/styles/full/public/2018/03/08/658858-577200-katrina-kaif-052217.jpg";
String img2 = "https://cdn.somethinghaute.com/wp-content/uploads/2018/07/katrina-kaif.jpg";
con.setRequestMethod("POST");
con.setDoOutput(true);
con.setRequestProperty("user_id", myid);
con.setRequestProperty("user_key", thekey);
con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
String body =
"img_1=" + URLEncoder.encode(img1, "UTF-8") + "&" +
"img_2=" + URLEncoder.encode(img2, "UTF-8");
try (OutputStream os = con.getOutputStream()) {
byte[] input = body.getBytes(StandardCharsets.UTF_8);
os.write(input);
}
您應該從代碼中刪除所有catch
塊,並修改您的方法簽名以包含throws IOException
。 你不希望你的應用程序的用戶認為操作成功了,如果實際上它失敗了,對吧?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.