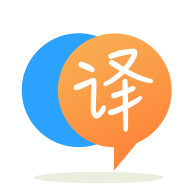
[英]How to scan characters from an ASCII image file(which is a text file that shows an image using ASCII characters) and print the image inverted using C?
[英]How to print characters from text file, using arguments, in C?
我需要使用參數-n
設置整數n
,該參數將設置為從給定.txt
文件末尾要打印的字符數。 這需要在沒有<stdio.h>
庫的情況下完成,因為它是有關系統調用的家庭作業。
我有一個能夠接受參數-n
並輸出用戶指定字符數的程序。 但是,在所需的輸出之后,它將打印出不可讀的字符和NULLS
列表,並導致我的終端出現故障。
#include <sys/types.h>
#include <fcntl.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char* argv[])
{
//declaration of variables
char buf[1000];
int fd;
int n;
//initialising n to zero
n = 0;
//checking if the program run call is greater than one argument
if(argc > 1){
//if the second argument is equal to '-n' then take the 3rd argument (the int) and put it into n using stroll (string to long)
if(!strncmp(argv[1], "-n", 2)){
n = atoi(argv[2]);
}
//if n has no set value from -n, set it to 200
if(n == 0){
n = 200;}
// open the file for read only
fd = open("logfile.txt", O_RDONLY);
//Check if it can open and subsequent error handling
if(fd == -1){
char err[] = "Could not open the file";
write(STDERR_FILENO, err, sizeof(err)-1);
exit(1);
}
//use lseek to place pointer n characters from the end of file and then use read to write it to the buffer
lseek(fd, (n-(2*n)), SEEK_END);
read(fd, buf, n);
//write out to the standard output
write(STDOUT_FILENO, buf, sizeof(buf)-1);
//close the file fd and exit normally with code 0
close(fd);
exit(0);
return(0);
}
我需要使用參數-n設置整數n 。
假設命令行看起來類似於prog.exe -n 4
,則main(int argc, char* argv[])
將填充為:
argc == 3
argv[] == {"programName.exe", "-n", "4"}
可以通過將-n
和4
組合在一起來將argc
減少為2: -n4
,或者完全消除-n
,然后在代碼中認識到argv [1](第二個參數)是所需值的字符串表示形式,並將其轉換為int
類型。
例:
programName.exe 3
然后像這樣編碼:
int main(int argc, char *argv[])
{
char *dummy;
int val;
if(argc != 2)
{
printf("Usage: prog.exe <n> where <n> is a positive integer value.\nProgram will now exit");
return 0;
}
// Resolve value of 2nd argument:
val = strtol(argv[1], &dummy, 10);
if (dummy == argv[1] || ((val == LONG_MIN || val == LONG_MAX) && errno == ERANGE))
{
//handle error
}
//use val to read desired content from file
...
return 0;
}
其他一些一般建議:
通常,用戶輸入必須盡可能保持可預測性。 在要求用戶輸入可接受的內容時要明確,並拒絕不符合要求的內容。 例如,如果程序期望一個數位的參數,則將其強制為正整數,並拒絕其他所有參數。 給定不符合要求的用戶輸入:
程序3 4 5
在代碼中檢測:
int main(int argc, char *argv[])
{
int val = 0;
char *dummy = NULL;
if(argc != 2)
{
printf("Usage: prog.exe <n> - Where <n> is an integer with value > 0\n Program will now exit.");
return 0;
}
val = strtol(argv[1], &dummy, 10);
if (dummy == argv[1] || ((val == LONG_MIN || val == LONG_MAX) && errno == ERANGE))
{
printf("Usage: prog.exe <n> - Where <n> is an integer with value > 0\n Program will now exit.");
return 0;
}
...
使用可移植函數(用fseek
代替lseek
,並用fopen
等open
)(閱讀示例以了解的原因 ),這里是代碼的改編,可以完成您描述的工作,包括使用prog.exe -n <n>
參數( argv == 3
)。
int main(int argc, char *argv[])
{
char *dummy;
char buf[1000];
int n;
//initialising n to zero
n = 0;
//checking if the program run call is greater than one argument
if(argc != 3)
{
printf("Usage: prog.exe -n <n> - Where <n> is an integer with value > 0\n Program will now exit.");
return 0;
}
n = strtol(argv[2], &dummy, 10);
if (dummy == argv[2] || ((n == LONG_MIN || n == LONG_MAX) && errno == ERANGE))
{
printf("Usage: prog.exe <n> - Where <n> is an integer with value > 0\n Program will now exit.");
return 0;
}
// open the file for read only
FILE *fd = fopen(".\\data.txt", "r");
//Check if it can open and subsequent error handling
if(!fd)
{
char err[] = "Could not open the file";
fputs(err, stdout);
return 0;
}
//use lseek to place pointer n characters from the end of file and then use read to write it to the buffer
fseek(fd, (n-(2*n)), SEEK_END);
if(fgets(buf, n, fd))
{
//write out to the standard output
fwrite(buf, 1, n, stdout);
}
else
{
;//handle error
}
//close the file fd and exit normally with code 0
fclose(fd);
return(0);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.