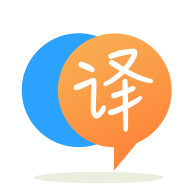
[英]Exception in thread “main” java.lang.StackOverflowError
[英]Exception in thread "main" java.lang.StackOverflowError after running Dijkstra's algorithm in an adjacency matrix
在加權鄰接矩陣中運行 Dijkstra 算法后,我試圖打印最短路徑。 嘗試打印路徑時出現計算器溢出錯誤。
我已經嘗試將起始節點更改為 long 和 BigInteger 類型,如該平台上以前的答案所建議的那樣,我也知道我正在使用遞歸方法,從而導致問題所在。
import java.util.*;
public class djikstra {
private static final int invalid = -1;
public djikstra(int matrix[][],int start) {
int numVertices = matrix[0].length;
int [] distances = new int [numVertices];
boolean [] isAdded = new boolean[numVertices];
for (int i=0;i<numVertices;i++) {
distances[i]= Integer.MAX_VALUE;
isAdded[i] = false;
}
distances[(int) start]=0;
int [] parents = new int [numVertices];
parents[start] = invalid;
for(int i=1;i<numVertices;i++) {
int closestNeighbour = -1;
int shortDist = Integer.MAX_VALUE;
for(int j=0; j <numVertices;j++) {
if(!isAdded[j] && distances[j]<shortDist) {
closestNeighbour = j;
shortDist = distances[j];
}
}
isAdded[closestNeighbour]=true;
for(int j = 0; j <numVertices;j++) {
int edgeDist = matrix[closestNeighbour][j];
if(edgeDist > 0 && ((closestNeighbour+edgeDist)<distances[j])) {
parents[j] = closestNeighbour;
distances[j] = shortDist + edgeDist;
}
}
}
printSol(start,distances,parents);
}
private static void printSol(int start,int[] distances,int[] parents) {
int numVertices=distances.length;
for(int i=0;i<numVertices;i++) {
if(i !=start) {
path(i,parents);
}
}
}
private static void path(int curr,int[]parents) {
if(curr== -1) {
return;
}
path(parents[curr],parents);
}
}
public static void Main(String args[]){
int matrix2[][]= {{0, 0, 0, 4, 12, 14, 0, 0, 0, 0, 0 ,0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 12, 0, 8, 0, 18, 15, 7},
{0, 0, 0, 11, 3, 3, 0, 0, 0, 0, 0, 13, 8, 10},
{4, 0, 0, 0, 10, 12, 0, 0, 0, 0, 10, 10, 13, 0},
{0, 0, 3, 10, 0, 2, 0, 0, 0, 0, 0, 10, 5, 11},
{0, 0, 3, 12, 2, 0, 0, 0, 0, 0, 0, 10, 5, 9 },
{20, 0, 0, 0, 0, 0, 0, 14, 10, 20, 16, 22, 0, 0},
{0, 12, 0, 0, 0 ,0 ,14, 0, 4, 6, 12, 0,0, 0 },
{0, 16, 0, 0, 0, 0, 10, 4, 0, 10, 14, 20, 0, 0},
{0, 8, 0, 0, 0, 0, 0, 6, 10, 0, 18, 0, 0, 15 },
{0, 0, 0, 10, 0, 0, 0, 12, 0, 0, 0, 6, 11, 11},
{0, 0, 0, 10, 10, 10, 0, 0, 0, 0, 6, 0, 5, 11},
{0, 0, 8, 0, 5, 5, 0, 0, 0, 0, 11, 5, 0, 8},
{0, 7, 10, 0, 11, 9, 0, 0, 0, 0, 0, 11, 8, 0}};
djikstra doDjikstra = new djikstra(matrix2,0);
}
Expected results:
0 4 13 1
Actual results:
Exception in thread "main" java.lang.StackOverflowError
at helperclasses.djikstra.path(djikstra.java:61)
StackOverflowError
是由path
方法中的無限遞歸觸發的。 parents[curr]
永遠不會持有 -1(基本情況),因此遞歸永遠不會停止。 您將需要確保最終使用 -1 為curr
調用path
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.