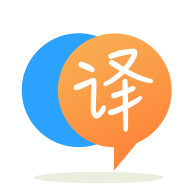
[英]How do I safely read a variable from one thread and modify it from another?
[英]How do I prevent another thread to modify a state flag?
我有一堂課
class Device
{
enum State {eStopped, eRunning}
State flag = eStopped;
public:
void run(){
if(flag==eRunning){return;}
/*some codes to start this device*/
flag = eRunning;
}
void stop(){
if(flag==eStopped){return;}
/*some codes to stop this device*/
flag = eStopped;
}
void doMaintenance(){
if(flag==eRunning){return;} // We can't do maintenance when the device is running
/*Here, the flag may be modified to eRunning in other threads*/
}
}
在doMaintenance()
函數, flag
將被其他線程之后改變(flag==eRunning)
檢查。 我如何優雅地防止這種情況發生?
還有其他問題要解決。 例如,假設設備處於停止狀態。 然后,在兩個線程中,執行run()
。 然后,兩個線程開始執行相同的啟動順序。 我們也應該注意這一點。
因此,最簡單的解決方案是不允許run()
, stop()
和doMaintenance()
任何一個同時運行。 這可以通過互斥鎖輕松解決:
class Device
{
enum State {eStopped, eRunning}
State flag = eStopped;
std::mutex m_mutex;
public:
void run(){
std::scoped_lock lock(m_mutex);
if(flag==eRunning){return;}
/*some codes to start this device*/
flag = eRunning;
}
void stop(){
std::scoped_lock lock(m_mutex);
if(flag==eStopped){return;}
/*some codes to stop this device*/
flag = eStopped;
}
void doMaintenance(){
std::scoped_lock lock(m_mutex);
if(flag==eRunning){return;} // We can't do maintenance when the device is running
/*Here, the flag may be modified to eRunning in other threads*/
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.