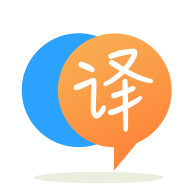
[英]Where Should i keep my jar files so that All projects commonly used them?
[英]Where should I be instantiating my “Ticket” to prevent them from all becoming the same price?
我有一個銷售活動門票的程序,尤其是“戲劇”。 門票以 10.0 的基值開始,並乘以 priceFactor。 我的價格因素工作正常,但門票最終都取走了最終門票價格的價值。
我已經嘗試在事件 class 和 Play 類的 addTicket 方法 (stackoverflowerror) 和 Ticket 構造函數中實例化 Ticket object (Ticket tick = new Ticket(this);...因為它需要一個事件的參數),但沒有成功. 我注意到的是,在不添加任何實例的情況下,我的票證對象 serialNumbers 仍然會增加,所以我不確定為什么如果*它正在創建一個新票證 object,我的所有票證最終都以相同的價格結束。
public class Play extends Event {
/**
* Creates a Play object with the description and price factor.
*
* @param description the description of the play
* @param priceFactor the price factor for the play
*/
public Play(String description, double priceFactor) {
super(description, priceFactor);
}
/**
* Creates a play with the given description and a price factor of 1.0.
*
* @param description the description of the play
*/
public Play(String description) {
this(description, 1.0);
}
/**
* Adds a ticket to the list of tickets sold for this Play object. It also
* adjusts the price factor.
*
* @param ticket the Ticket object to be added
* @return true iff the Ticket object could be added.
* @throws NoSpaceException
* @throws UnsupportedOperationException
*/
@Override
public boolean addTicket(Ticket ticket) throws UnsupportedOperationException, NoSpaceException {
double i = this.getPriceFactor();
if (this.getTickets().size() < 3) {
super.addTicket(ticket);
}
else if (this.getTickets().size() == 3) {
super.setPriceFactor(i * 1.2);
i = super.getPriceFactor();
super.addTicket(ticket);
}
else if (this.getTickets().size() == 4) {
super.setPriceFactor(i * 1.2);
super.addTicket(ticket);
}
return true;
}
/**
* Returns a String representation.
*
*/
@Override
public String toString() {
return "Play" + " " + super.getEventId() + " " + super.getDescription() + " " + super.getPriceFactor();
}
}
-----------------------------------------------------------------------------
public abstract class Event {
private String description;
protected int ticketsSold;
private int eventId;
private double priceFactor;
private static int counter = 1;
private static final int CAPACITY = 5;
private ObservableList<Ticket> tickets = FXCollections.observableArrayList();
/**
* Stores the description and price factor and assigns a unique id to the event.
* The constructor also allocates the array tickets.
*
* @param description a description of this Play
* @param priceFactor the price factor for this Play
*
*/
public Event(String description, double priceFactor) {
this.description = description;
this.priceFactor = priceFactor;
this.eventId = computeSerialNumber();
}
/**
* Receives the description and stores that and a price factor of 1.0. Besides,
* it assigns a unique id to the event. The constructor also allocates the array
* tickets.
*
* @param description a description of this Play
*
*/
public Event(String description) {
this(description, 1.0);
}
/**
* Returns the unique id of the play
*
* @return id of the play
*
*/
public int getEventId() {
return eventId;
}
/**
* Returns the tickets list
*
* @return the tickets list
*/
public ObservableList<Ticket> getTickets() {
return tickets;
}
/**
* Sets the price factor for the event.
*
* @param priceFactor the new price factor
*/
public void setPriceFactor(double priceFactor) {
this.priceFactor = priceFactor;
}
/**
* Computes and returns the total proceeds for this event.
*
* @return total proceeds
*/
public double getProceeds() {
double sum = 0;
for (Ticket t : tickets) {
sum += t.getPrice();
}
return sum;
}
/**
* Compares this Play with object. Follows the semantics of the equals method in
* Object.
*
*/
@Override
public boolean equals(Object object) {
if (this == object)
return true;
else
return false;
}
public int hashcode() {
return this.eventId;
}
/**
* Returns the description of the Play object
*
* @return description
*/
public String getDescription() {
return description;
}
/**
* Returns the price factor
*
* @return price factor
*/
public double getPriceFactor() {
return priceFactor;
}
/**
* Setter for description
*
* @param description the new description
*/
public void setDescription(String description) {
this.description = description;
}
/**
* Returns a unique serial number. This is a helper method.
*
* @return serial number
*/
private int computeSerialNumber() {
int i = counter;
counter++;
return i;
}
/**
* Adds a ticket to the list of tickets sold for this Play object.
*
* @param ticket the Ticket object to be added
* @return true iff the Ticket object could be added.
* @throws NoSpaceException
* @throws UnsupportedOperationException
*/
public boolean addTicket(Ticket ticket) throws UnsupportedOperationException, NoSpaceException {
if (tickets.size() == CAPACITY)
return false;
else
tickets.add(ticket);
ticketsSold++;
return true;
}
/**
* Returns a String representation of this Event object
*/
@Override
public String toString() {
return description + " " + eventId;
}
}
--------------------------------------------------------------------------------
public class Ticket {
private static int counter = 1;
private int serialNumber;
private double price;
private static double PRICE = 10.0;
private Event event;
/**
* Creates a ticket for an event. An exception is thrown if there is no space.
*
* @param event the event for which the ticket is being created.
* @throws NoSpaceException
*/
public Ticket(Event event) throws NoSpaceException, UnsupportedOperationException {
this.event = event;
event.addTicket(this);
this.serialNumber = computeSerialNumber();
}
/**
* Returns the price of the ticket
*
* @return ticket price
*/
public double getPrice() {
price = Ticket.PRICE * event.getPriceFactor();
return price;
}
/**
* Generates a String representation of the Ticket.
*/
@Override
public String toString() {
return "Ticket serialNumber = " + serialNumber + ", " + "price =" + this.getPrice();
}
/*
* Creates a serial number for the ticket.
*/
private static int computeSerialNumber() {
int i = counter;
counter++;
return i;
}
}
public class Test {
public static void main(String[] args) throws UnsupportedOperationException, NoSpaceException {
double a,b,c,d,e;
Play p = new Play("p1", 1.0);
Ticket tick = new Ticket(p);
Ticket tick2 = new Ticket(p);
Ticket tick3 = new Ticket(p);
Ticket tick4 = new Ticket(p);
Ticket tick5 = new Ticket(p);
p.addTicket(tick);
p.addTicket(tick2);
p.addTicket(tick3);
p.addTicket(tick4);
p.addTicket(tick5);
a = tick.getPrice();
b = tick2.getPrice();
c = tick3.getPrice();
d = tick4.getPrice();
e = tick5.getPrice();
System.out.println(a);
System.out.println(b);
System.out.println(c);
System.out.println(d);
System.out.println(e);
}
}
訂單的預期結果 [10.0, 10.0, 10.0, 12.0, 14.399]
實際結果 [14.399, 14.399, 14.399, 14.399, 14.399]
DerMolly 將 pricefactor 帶入 Ticket class 的答案的替代方法是僅計算創建時的價格,而不是每個 getPrice:
public Ticket(Event event) throws NoSpaceException, UnsupportedOperationException {
this.event = event;
event.addTicket(this);
this.price = Ticket.PRICE * event.getPriceFactor();
this.serialNumber = computeSerialNumber();
}
public double getPrice() {
return price;
}
目前,所有Ticket
對象都持有對其Event
的引用並從那里獲取priceFactor
。 然后,您有時會更改Event
中的priceFactor
,所有Ticket
對象都會從其Event
中獲取新的priceFactor
。
public double getPrice() {
price = Ticket.PRICE * event.getPriceFactor();
return price;
}
您可以將priceFactor
(通過構造函數或其他方式)放入您的Ticket
對象中。
public class Ticket {
private static int counter = 1;
private int serialNumber;
private double price;
private static double PRICE = 10.0;
private double priceFactor;
private Event event;
public Ticket(Event event, double priceFactor) throws NoSpaceException, UnsupportedOperationEtception {
this.event = event;
this.priceFactor = priceFactor;
event.addTicket(this);
this.serialNumber = computeSerialNumber();
}
public double getPrice() {
price = Ticket.PRICE * this.getPriceFactor();
return price;
}
}
如果您不想直接設置priceFactor
,您也可以在Ticket
的構造函數中復制它的值
public class Ticket {
private static int counter = 1;
private int serialNumber;
private double price;
private static double PRICE = 10.0;
private double priceFactor;
private Event event;
public Ticket(Event event) throws NoSpaceException, UnsupportedOperationEtception {
this.event = event;
this.priceFactor = event.getPriceFactor;
event.addTicket(this);
this.serialNumber = computeSerialNumber();
}
public double getPrice() {
price = Ticket.PRICE * this.getPriceFactor();
return price;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.