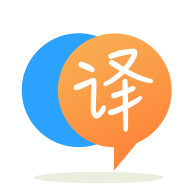
[英]How to filter a list of objects by matching properties with objects from another list
[英]How to create a list of matching objects from multiple lists and delete objects in the matching list such that it reflects in the original list
很抱歉,如果有人問過這個問題,但我在谷歌搜索中找不到類似的東西,所以就到這里。 假設我有兩個對象
筆記本
public class NoteBook {
private String name;
private String description;
public NoteBook(String name, String description) {
this.name = name;
this.description = description;
}
}
和筆記
public class Note {
private String sourceNoteBook
private String name;
private String category;
private String details;
public Note(String sourceNoteBook,String name, String category, String details) {
this.sourceNoteBook = sourceNoteBook;
this.name = name;
this.category = category;
this.details = details;
}
}
在程序中,用戶可以創建多個 NoteBook 對象,每個 NoteBook 存儲可變數量的 Notes。 最終我想添加一個搜索 function 可以按類別或名稱搜索筆記並返回找到的筆記列表。
通常我會使用 2 個 For 循環來遍歷筆記本列表,然后遍歷每個筆記本的筆記列表並比較字符串。 像這樣的東西:
For (NoteBook noteBook: noteBooks) {
For(Note note :noteBooks.getNoteList){
if (note.getCategory().contains(someString)) {
matchingNotes.add(notes);
}
}
}
但是,我現在希望能夠從 matchingNotes 列表中刪除筆記,這樣原始筆記本中的筆記也會被刪除。
存儲和搜索這兩個 class 的最佳方法是什么,以便我可以實現這樣的 function。
編輯:
只是為了澄清,最終結果是我希望用戶能夠在所有筆記本中搜索筆記類別,然后程序將返回與該類別匹配的筆記列表。 然后他/她可以從該列表中刪除一條筆記,這樣它也可以在原始筆記本中刪除。 例如完全從程序中刪除。
迭代器:
可能是最簡單的解決方案。 並且由於 java 在foreach
循環的底層使用迭代器,因此性能是相同的。
For (NoteBook noteBook: noteBooks) {
Iterator<Note> it = noteBooks.getNoteList().iterator();
while (it.hasNext()) {
Note note = it.next();
if (note.getCategory().equals(someString)) {
it.remove();
}
}
}
SQL:
這將是最佳的。 然而,即使使用輕量級的東西,例如 H2 或 SQLite,也需要重構。 而且在非常輕量級的應用程序中也不是一個選項。
高效的:
如果您只按類別或名稱搜索,您可以使用 2 個地圖:
Map<String, Note> notesByCategory;
Map<String, Note> notesBytName
這將需要O(n)
memory 來存儲地圖,但會在O(1)
時間內進行非常有效的查找(與當前O(n)相比)。 我會避免這種解決方案,因為在筆記內容和地圖之間很容易實現不一致的 state。
編輯:
var newNoteNames = newList.stream().map(Note::getName).collect(Collectors.toSet());
var oldNoteNames = noteBooks.stream().flatMap(Notebook::getNodeList).map(Note::getName).collect(Collectors.toSet());
var removedNames = oldNoteNames.removeAll(newNoteNames);
for (var removedName : removedNames) {
for (NoteBook noteBook: noteBooks) {
Iterator<Note> it = noteBooks.getNoteList().iterator();
while (it.hasNext()) {
Note note = it.next();
if (note.getName().contains(removedName)) {
it.remove();
}
}
}
}
為什么不將筆記本信息存儲在筆記 class 中?
public class Note {
private NoteBook sourceNoteBook;
private String name;
private String category;
private String details;
public Note(NoteBook sourceNoteBook,String name, String category, String details) {
this.sourceNoteBook = sourceNoteBook;
this.name = name;
this.category = category;
this.details = details;
}
}
每次要記錄的數據操作都會影響存儲它的筆記本
編輯我的答案
您可以創建一個 map 當鍵是筆記並且值是筆記本時 keySet 將返回給用戶,一旦他選擇了鍵,您就知道從哪個筆記本中刪除的值並且您擁有可以刪除的鍵筆記。 這是你的意思嗎?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.