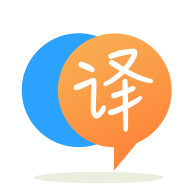
[英]Get public download URL from uploaded file in firebase storage with Java rest API
[英]Trouble getting download Url of uploaded file in Android Firebase
我嘗試將個人資料圖片上傳到 Firebase 存儲,然后將下載的 URL 保存到數據庫。 上傳工作完美,但我在下載 URL 時遇到問題。 我已經在 Stack Overflow 上嘗試了幾乎所有東西。 我正在分享相關代碼。
private String user_Name, user_Email, user_Password, user_Age, user_Phone, imageUri;
Uri imagePath;
選擇圖像
userProfilePic.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent();
intent.setType("image/*"); //Specify the type of intent
intent.setAction(Intent.ACTION_GET_CONTENT); //What action needs to be performed.
startActivityForResult(Intent.createChooser(intent, "Select Image"),
}
});
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { //Here we get the result from startActivityForResult().
if(requestCode == PICK_IMAGE && resultCode == RESULT_OK && data.getData() != null){
imagePath = data.getData(); //data.getData() holds the path of the file.
try {
Bitmap bitmap = MediaStore.Images.Media.getBitmap(getContentResolver(), imagePath); //this converts the Uri to an image.
userProfilePic.setImageBitmap(bitmap);
imageTrue = 1;
} catch (IOException e) {
e.printStackTrace();
}
}
super.onActivityResult(requestCode, resultCode, data);
}
上傳數據
private void sendUserData (){
FirebaseDatabase firebaseDatabase = FirebaseDatabase.getInstance();
DatabaseReference myRef = firebaseDatabase.getReference("Users").child(firebaseAuth.getUid());
final StorageReference imageReference = storageReference.child(firebaseAuth.getUid()).child("Images").child("Profile Pic");
//Here the root storage reference of our app storage is is "storageReference".
//.child(firebaseAuth.getUid()) creates a folder for every user. .child("images")
//creates another subfolder Images and the last child() function
//.child("Profile Pic") always gives the name of the file.
//User id/Images/profile_pic.png
//We can follow the same process for all other file types.
if(imageTrue==1){
UploadTask uploadTask = imageReference.putFile(imagePath); //Now we need to upload the file.
uploadTask.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception e) {
Toast.makeText(getApplicationContext(), "File Upload Failed", Toast.LENGTH_SHORT).show();
}
}).addOnSuccessListener(new OnSuccessListener<UploadTask.TaskSnapshot>() {
@Override
public void onSuccess(UploadTask.TaskSnapshot taskSnapshot) {
imageReference.getDownloadUrl().addOnSuccessListener(new OnSuccessListener<Uri>() {
@Override
public void onSuccess(Uri uri) {
Uri downloadUri = uri;
imageUri = downloadUri.toString();
}
});
Toast.makeText(getApplicationContext(), "File Uploaded Successfully", Toast.LENGTH_SHORT).show();
}
});
}
UserProfile userProfile = new UserProfile(user_Name, user_Age, user_Email, user_Phone, imageUri);
myRef.setValue(userProfile);
Toast.makeText(getApplicationContext(), "User Data Sent.", Toast.LENGTH_SHORT).show();
}
你的代碼是對的。 您只需要在sendUserData() function 中的代碼中進行一些更正。 您將在 UploadTask 的onSuccess
中獲取您的imageUrl
DatabaseReference myRef;
private void sendUserData (){
FirebaseDatabase firebaseDatabase = FirebaseDatabase.getInstance();
myRef = firebaseDatabase.getReference("Users").child(firebaseAuth.getUid());
final StorageReference imageReference = storageReference.child(firebaseAuth.getUid()).child("Images").child("Profile Pic");
//Here the root storage reference of our app storage is is "storageReference".
//.child(firebaseAuth.getUid()) creates a folder for every user. .child("images")
//creates another subfolder Images and the last child() function
//.child("Profile Pic") always gives the name of the file.
//User id/Images/profile_pic.png
//We can follow the same process for all other file types.
if(imageTrue==1){
UploadTask uploadTask = imageReference.putFile(imagePath); //Now we need to upload the file.
uploadTask.addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception e) {
Toast.makeText(getApplicationContext(), "File Upload Failed", Toast.LENGTH_SHORT).show();
}
}).addOnSuccessListener(new OnSuccessListener<UploadTask.TaskSnapshot>() {
@Override
public void onSuccess(UploadTask.TaskSnapshot taskSnapshot) {
imageReference.getDownloadUrl().addOnSuccessListener(new OnSuccessListener<Uri>() {
@Override
public void onSuccess(Uri uri) {
Uri downloadUri = uri;
imageUri = downloadUri.toString();
saveUserDetails(imageUri); // Image uploaded
}
});
Toast.makeText(getApplicationContext(), "File Uploaded Successfully", Toast.LENGTH_SHORT).show();
}
});
}else{
saveUserDetails(""); // Image not uploaded
}
}
常見的 function 用於saveUserDetails
:
public void saveUserDetails(String imageUri){
UserProfile userProfile = new UserProfile(user_Name, user_Age, user_Email, user_Phone, imageUri);
myRef.setValue(userProfile);
Toast.makeText(getApplicationContext(), "User Data Sent.", Toast.LENGTH_SHORT).show();
}
根據Firebase 官方文檔,您可以在addOnCompleteListener
方法上使用UploadTask
下載 URl。
UploadTask uploadTask =null;
final StorageReference ref = storageReference.child(firebaseAuth.getUid()).child("Images").child("Profile Pic").child(imagePath);
uploadTask = ref.putFile(file);
Task<Uri> urlTask = uploadTask.continueWithTask(new Continuation<UploadTask.TaskSnapshot, Task<Uri>>() {
@Override
public Task<Uri> then(@NonNull Task<UploadTask.TaskSnapshot> task) throws Exception {
if (!task.isSuccessful()) {
throw task.getException();
}
// Continue with the task to get the download URL
return ref.getDownloadUrl();
}
}).addOnCompleteListener(new OnCompleteListener<Uri>() {
@Override
public void onComplete(@NonNull Task<Uri> task) {
if (task.isSuccessful()) {
Uri downloadUri = task.getResult();
saveUserDetails(uri);
} else {
// Handle failures
// ...
}
}
});
另一種替代方法,在成功上傳圖片后,使用 get downloadUrl 查詢並獲取您的圖片 url。希望這可能對您有所幫助!
private void getImageUrl(){
storageReference.child(firebaseAuth.getUid()).child("Images").child("Profile Pic").child(imagePath).getDownloadUrl().addOnSuccessListener(new OnSuccessListener<Uri>() {
@Override
public void onSuccess(Uri uri) {
saveUserDetails(uri);
}
}).addOnFailureListener(new OnFailureListener() {
@Override
public void onFailure(@NonNull Exception exception) {
// Handle any errors
}
});
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.