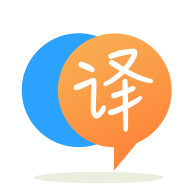
[英]My program crashes when I try to read a NULL value using sqlite in C
[英]my program crashes when i have read in all the scores
這個程序在我讀完所有評委的分數並且編譯器沒有給出任何警告后關閉/崩潰。 當它讀入所有分數時,它應該打印出所有分數以及最高分數、平均分數和最低分數。
#include <stdio.h>
/*
Prints out info to the user.
*/
void printInfo(){
printf("Program information\n");
printf("The program reads in the number of judges and the score from each judge.\n");
printf("Then it calculates the average score without regard to the lowest and\n");
printf("highest judge score. Finally it prints the results (the highest, the\n");
printf("lowest and the final average score).\n\n");
}
/*
Reads in how many judges the user wants to have.
returns the number of judges.
*/
int readJudge(){
int tempNumbJudge;
do{
printf("Number of judges (min 3 and max 10 judges)? ");
scanf("%d", &tempNumbJudge);
}while(3 > tempNumbJudge || tempNumbJudge > 10);
return tempNumbJudge;
}
/*
Reads in the score from each judge
Parameters:
arr - the array where to put the scores
judges - the number of judges
*/
void readJudgeScore(double arr[], int judges){
printf("\n");
double temp = 0;
int i = 0;
while(i <= judges - 1){
printf("Score from judge %d? ", i+1);
scanf("%lf", &temp);
arr[i] = temp;
i++;
}
}
/*
Prints of the score from each judge.
Parameters:
arr - the array with the scores
judges - the number of judges
*/
void printJudgeScore(double arr[], int judges){
printf("\n");
printf("Loaded scores:\n");
for (int i = 0; i < judges; i++) {
printf("Judge %d: %.1f\n", i+1 , arr[i]);
}
}
/*
Finds the highest score.
Parameters:
arr - the array with the scores
judges - the number of judges
returns the highest score
*/
double findMaxValue(double arr[], int judges){
double maxValue = arr[0];
for(int i = 0; i < judges; i++){
if(arr[i] > maxValue){
maxValue = arr[i];
}
}
return maxValue;
}
/*
Finds the lowest score.
Parameters:
arr - the array with the scores
judges - the number of judges
returns the lowest score
*/
double findMinValue(double arr[], int judges){
double minValue = arr[0];
for(int j = 0; j < judges; j++){
if(arr[j] < minValue){
minValue = arr[j];
}
}
return minValue;
}
/*
Calculates the average score.
Parameters:
arr - the array with the scores
judges - the number of judges
max - the highest score
min - the lowest score
returns the average score
*/
double findAverage(double arr[], int judges, double max, double min){
double sum = 0;
double average = 0;
for(int k = 0; k < judges; k++){
sum = sum + arr[k];
}
average = (sum - max - min) / (judges - 2);
return average;
}
/*
Prints out the final results
Parameters:
max - the highest score
min - the lowest score
average - the average score
*/
void printFinalResult(double max, double min, double average){
printf("\n");
printf("Final result:\n");
printf("Highest judge score: %.1f\n", max);
printf("Lowest judge score: %.1f\n", min);
printf("Final average score: %.1f", average);
}
int main(){
int numbJudge;
double maxValue;
double minValue;
double average;
printInfo();
numbJudge = readJudge();
double points[numbJudge];
readJudgeScore(points, numbJudge);
printJudgeScore(points, numbJudge);
maxValue = findMaxValue(points, numbJudge);
minValue = findMinValue(points, numbJudge);
average = findAverage(points, numbJudge, maxValue, minValue);
printFinalResult(maxValue, minValue, average);
return 0;
}
我使用的編譯器是 gcc 帶有標志 std=c99 和 -Wall
您需要傳遞變量的地址,否則scanf()
應該如何寫入它?
你需要:
scanf("%lf", &temp);
還要檢查返回值,I/O 可能會失敗。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.