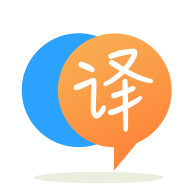
[英]How to re-render Formik values after updated it - React Native?
[英]How to re-render updated values in react
我正在嘗試以固定間隔無限循環通過 Rainbow colors 並在瀏覽器 DOM 中更新該顏色。
顏色值每半秒改變一次。 但它不是在 DOM 中重新渲染。
import React from 'react'
const Rainbow = (WrapedComponent) =>{
const colors = ['violet', 'indigo', 'blue', 'green', 'yellow', 'orange', 'red'];
let i=0;
let newColor;
setInterval(() => {
newColor = colors[i];
if (i === (colors.length - 1)) {
i = 0;
} else {
i++;
}
}, 500);
let newStyle = {
color: newColor
}
return (props) => {
return (
<div style={newStyle}>
<h1>Hello World</h1>
<WrapedComponent {...props}/>
</div>
)
}
}
export default Rainbow
您正在更新newColor
就好了; 問題是newStyle
(和newStyle.color
)只分配給一次。 盡管newStyle.color
最初引用newColor
的第一個值,但它與newStyle.color
不斷引用newColor
的未來值不同。
只需將超時中的 newColor = colors[i newColor = colors[i]
更改為newStyle.color = colors[i]
即可更新 object。 如果您希望newStyle
應用於 DOM 中的某些內容,您當然需要確保代碼也已到位。
編輯:
現在已經明確這是 React,您需要進行一些更改。 React 不會監控局部變量的變化並將這些變化應用到 DOM。 相反,您必須專門觸發 React 中的更新。 最直接的方法是通過state 。 對 state 的更改會導致重新渲染。 您還需要將計時器間隔設置為可以在卸載組件時關閉的效果。
import React from 'react'
const { useEffect, useState } = React;
const colors = ['violet', 'indigo', 'blue', 'green', 'yellow', 'orange', 'red'];
const Rainbow = (WrappedComponent) => {
return (props) => {
const [color, setColor] = useState(0);
useEffect(() => {
const timeout = setTimeout(() => {
setColor((color + 1) % colors.length);
}, 500);
return () => clearTimeout(timeout);
}, [color]);
return (
<div style={{ color: colors[color] }}>
<h1>Hello World</h1>
<WrappedComponent {...props}/>
</div>
);
}
}
export default Rainbow
與其創建一個新的 scope 並遞歸調用 loopforver ... 永遠 ... 並每次創建一個新的 setTimeout,只需使用 setInterval。 我還將 newStyle.color 添加到循環中,否則您將永遠不會設置任何內容。 newstyle.color = newColor 只發生一次,似乎您希望它以某種方式被綁定。
const colors = ['violet', 'indigo', 'blue', 'green', 'yellow',
'orange', 'red'
];
let i = 0;
let newColor;
let newStyle = {};
let intervalId = window.setInterval(() => {
newStyle.color = colors[i];
//Apply newStyle to some dom element here if need be
i++
if (i === 6) {
i = 0;
}
}, 500);
//When you're ready to be done
window.clearInterval(intervalId);
你的問題最初被標記為reactjs
之前被刪除,因為不清楚你的示例代碼中的反應在哪里起作用。 但是,您只需調用this.setState()
代替console.log()
即可更新組件 state 中的color
屬性。
然后還有一些其他的事情需要注意:
setTimeout()
調用 function,不如使用setInterval()
。"red"
,因為之前已重置索引。 我還更改了代碼以動態考慮數組的長度,而無需對其進行硬編碼。 const colors = ['violet', 'indigo', 'blue', 'green', 'yellow', 'orange', 'red']; let i = 0; let newColor; setInterval(() => { newColor = colors[i]; document.documentElement.style.backgroundColor = newColor; // this is where you would call // this.setState({color: newColor}); if (i === (colors.length - 1)) { i = 0; } else { i++; } }, 500);
setTimeout 方法超時 500 毫秒,並且一直在執行,到執行 setTimeout 時,
let newStyle = {
color: newColor
}
已經執行。 這就是您無法獲得新顏色的原因。
可能是這樣嗎?
const testElement = document.querySelector('#divTest'), btStop = document.querySelector('#Bt-Stop'), colors = ['violet', 'indigo', 'blue', 'green', 'yellow', 'orange', 'red'] let indexColor = 0 testElement.style.backgroundColor = colors[indexColor] let refInterval = setInterval(_=>{ indexColor = ++indexColor % colors.length testElement.style.backgroundColor = colors[indexColor] }, 1500) btStop.onclick=_=>{ clearInterval(refInterval) }
#divTest { width: 300px; height: 200px; } #Bt-Stop { margin-top: 1em; }
<div id="divTest"></div> <button id="Bt-Stop">stop</button>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.