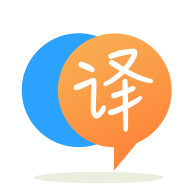
[英]Retrieved data from API into an array changes it's value when attempting to console.log in render function (react tsx)
[英]How to console.log data retrieved from asynchronous api call?
我正在對服務器進行 API 調用並發送到 React 組件。 我正在嘗試確定數據是否已成功加載以及加載后的外觀。
但是,當我console.log
記錄數據時,我顯然只收到promise
object。 有誰知道如何確保console.log
僅在 API 調用完成后觸發? 我嘗試使用async
和await
雖然我一直在努力讓它工作。
import React from 'react'
import { Field, reduxForm } from 'redux-form'
import { connect } from 'react-redux'
// actions
import { getPlayers, deletePlayer } from '../actions'
// components
import RenderPlayer from './RenderPlayer'
class DeletePlayer extends React.Component {
constructor (props) {
super(props)
this.players = null
this.renderPlayerList = null
}
componentDidMount () {
this.players = this.props.getPlayers()
this.renderPlayerList = () => {
//map over each player entry in database and send all details as props to rednderPlayer
this.players = this.players.map((player) => { return <RenderPlayer {...player} /> })
return this.players
}
console.log(this.players)
return this.players
}
render () {
return (
<div>
{this.players}
</div>
)
}
}
export default connect(null, { getPlayers, deletePlayer })(DeletePlayer)
如果this.players
返回 promise,您可以使用 .then .then()
對其響應進行處理。
this.players.then(res => console.log(res)).catch(err => console.log(err))
這是您的代碼,帶有async
和await
並進行了一些清理:
import React from 'react'
import { connect } from 'react-redux'
import { getPlayers, deletePlayer } from '../actions'
import RenderPlayer from './RenderPlayer'
class DeletePlayer extends React.Component {
state = {
players: [],
}
async componentDidMount () {
const { getPlayers } = this.props
try {
const players = await getPlayers()
console.log(players)
this.setState({ players })
}
catch (error) {
console.error(error)
}
}
render () {
const { players } = this.state
return (
<div>
{players.map(player => <RenderPlayer {...player} />)}
</div>
)
}
}
export default connect(null, { getPlayers, deletePlayer })(DeletePlayer)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.