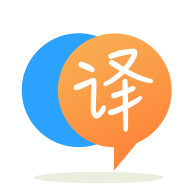
[英]C++ Reading a SPECIFIC line from a text file and comparing it with an input
[英]Reading specific data from a line in a file - C++
我有一個像這樣划分的.txt文件:
(P1, 3): (E10, E20, E1, E3)
(P2, 2): (E10, E20, E2, E5)
(P3, 2): (E10, E20)
我只想將每行的數字保存在一個數組中。 例如:第一個是 [1,3,10,20,1,3]。 我怎樣才能做到這一點?
使用ifstream逐行遍歷文件。
將字符串讀取到 std::string 后,使用正則表達式搜索來查找新讀取的字符串中出現的數字。
您要用於提取字符串中所有數字的正則表達式如下:
https://regex101.com/r/yWJp5p/3
使用示例:
#include <regex>
#include <iostream>
#include <string>
std::vector<std::string> fetchMatches(std::string str, const std::regex re) {
std::vector<std::string> matches;
std::smatch sm; // Use to get the matches as string
while (regex_search(str, sm, re)) {
matches.push_back(sm.str());
str = sm.suffix();
}
return matches;
}
int main() {
std::string example_input = "(P1, 3): (E10, E20, E1, E3)";
std::regex re{"\\d+"};
auto matches = fetchMatches(example_input, re);
for (const auto& match : matches) {
std::cout << match << std::endl;
}
return 0;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.