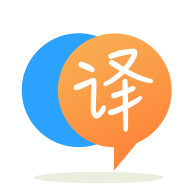
[英]How to print list elements (which are also lists) in separated lines in Python
[英]Efficiently remove elements from a list in python which are also in given lists
所以我有兩個列表和一個主列表。 如果主列表中的元素存在於其他兩個列表中的任何一個中,則應刪除它們。
例子:
s1 = [1,2,3,4,7]
s2 = [3,4,5,6,20]
mainlist = [6,7,8,9,10,11,12,13,14,15]
因此,由於 mainList 包含元素 6 和 7,它們也存在於 s1 或 s2 中,因此應將它們刪除,結果應如下所示。
resultList = [8,9,10,11,12,13,14,15]
我的代碼:
for j in mainlist[:]:
if j in s1 or j in s2:
mainlist.remove(j)
反正有沒有使用for循環? 我需要一種有效的方法來降低時間復雜度。 謝謝 !
您可以使用列表理解,它可以很好地解決這類問題:
result = [x for x in mainlist if x not in s1 and x not in s2]
使用list/set
操作,您可以執行以下操作之一
result = list(set(mainlist) - (set(s1) | set(s2))) # remove the concat of s1&s2
result = list(set(mainlist) - set(s1) - set(s2)) # remove s1 and s2
可能您可以使用列表推導創建另一個列表
res = [i for i in test_list if i not in s1 and i not in s2]
或使用filter() + lambda
res = filter(lambda i: i not in s1 and i not in s2, mainlist)
或使用for
循環
for elem in mainlist:
if elem in s1 or elem in s2:
mainlist.remove(elem)
嘗試這個:
mainlist = list(set(mainlist) - (set(s1)|set(s2)))
在這里,我假設所有列表都沒有重復元素。
您可以計時並與其他方法進行比較。
time_idx = time()
result = [x for x in mainlist if x not in s1 and x not in s2]
print(time() - time_idx)
0.00012612342834472656
time_idx = time()
mainlist = list(set(mainlist) - (set(s1)|set(s2)))
print(time() - time_idx)
0.00010609626770019531
改進是顯着的,因為這是一個小列表。
您可以將s1
和s2
轉換為dict
s1 = {i:i for i in s1}
s2 = {i:i for i in s2}
mainlist = [i for i in mainlist if i not in s1 or i not in s2]
將列表轉換為 dict 會浪費一些時間,但由於 python 字典基本上是 hash 表,因此搜索復雜度為O(1)
而不是O(n)
,就像列表一樣。
所以最終它不是O(n^2)
,而是O(n)
或類似的東西。
如果你試試這個,請告訴我。
只需使用 set 操作過濾掉
>>> list(set(mainlist) - set(s1+s2))
>>> [8, 9, 10, 11, 12, 13, 14, 15]
您還可以使用計數器來執行此操作:
from collections import Counter
print(list(Counter(mainlist) - Counter(s1) - Counter(s2)))
Out[309]: [8, 9, 10, 11, 12, 13, 14, 15]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.