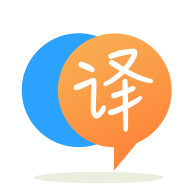
[英]React functional component TypeError: Cannot read property 'props' of undefined
[英]Cannot read property 'props' of undefined in functional component
所以我有以下反應功能組件:
import React from "react";
import LNSelect from "../LNSelect/LNSelect";
import { CountryRegionData } from "react-country-region-selector";
const parsed = CountryRegionData.map(
([country_name, country_code, cities]) => ({
country_name,
country_code,
cities: cities
.split("|")
.map(cityData => cityData.split("~"))
.map(([city_name, city_code]) => ({ city_name, city_code }))
})
);
const regions = parsed
.find(country => country.country_name === this.props.country)
.cities.map(({ city_name, city_code }) => ({
label: city_name,
value: city_code
}));
const LNSelectRegion = props => {
return <LNSelect options={regions} {...props} />;
};
export default LNSelectRegion;
基本上我使用 package 來獲取國家和他們的城市/城市代碼,然后找到一個作為支柱country
傳遞的特定國家並將其城市映射到label
和value
數組以用於我有的選擇器組件,這個代碼的問題是在.find
行上,我收到錯誤Cannot read property 'props' of undefined
,我想解決這個問題,我還想為 country 屬性設置一個默認值,以防它為空( if this.props.country === "" {this.props.country = "United States}
),我該怎么做?
1)在功能組件中,您可以使用props
而不是this.props
獲取道具。
2)您只能在功能組件LNSelectRegion
中獲取道具。
所以我只是在這個標准上重新編寫你的代碼,希望它會起作用
import React from "react";
import LNSelect from "../LNSelect/LNSelect";
import { CountryRegionData } from "react-country-region-selector";
const parsed = CountryRegionData.map(
([country_name, country_code, cities]) => ({
country_name,
country_code,
cities: cities
.split("|")
.map(cityData => cityData.split("~"))
.map(([city_name, city_code]) => ({ city_name, city_code }))
})
);
const LNSelectRegion = props => {
const regions = parsed
.find(country => country.country_name === props.country)
.cities.map(({ city_name, city_code }) => ({
label: city_name,
value: city_code
}));
return <LNSelect options={regions} {...props} />;
};
export default LNSelectRegion;
你不能在功能組件中使用this
,所以你的代碼應該是:
const regions = parsed
.find(country => country.country_name === props.country)
.cities.map(({ city_name, city_code }) => ({
label: city_name,
value: city_code
}));
您還需要 function 並在此塊周圍傳遞 props 參數
const YourComponent = (props) => {
// your code above
}
const regions
function 應該寫在傳遞 props 的功能組件內。
// Functional component
const Child = props => {
const regions = parsed
.find(country => country.country_name === props.country)
.cities.map(({ city_name, city_code }) => ({
label: city_name,
value: city_code
}));
return (
<div></div>
)
}
因為this.props.country
中的this
是未定義的。 嘗試通過 prop 獲取regions
const getRegions = (country) => parsed
.find(country => country.country_name === country)
.cities.map(({ city_name, city_code }) => ({
label: city_name,
value: city_code
}));
const LNSelectRegion = props => {
return <LNSelect options={getRegions(props.country)} {...props} />;
};
您的parsed
和regions
常量在實際組件之外,您無法在那里訪問道具。 功能組件中也沒有“this”。 看看下面的代碼:
import React from "react";
import LNSelect from "../LNSelect/LNSelect";
import { CountryRegionData } from "react-country-region-selector";
const parsed = CountryRegionData.map(
([country_name, country_code, cities]) => ({
country_name,
country_code,
cities: cities
.split("|")
.map(cityData => cityData.split("~"))
.map(([city_name, city_code]) => ({ city_name, city_code }))
})
);
const getRegions = (countryFromProps) => parsed
.find(country => country.country_name === countryFromProps)
.cities.map(({ city_name, city_code }) => ({
label: city_name,
value: city_code
}));
const LNSelectRegion = (props) => {
return <LNSelect options={getRegions(props.country)} {...props} />;
};
LNSelectRegion.defaultProps = {
country: "United States"
}
export default LNSelectRegion;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.