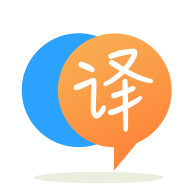
[英]How to create array and store values in it without knowing its size in Arduino
[英]How to read a text file and store into an array without knowing the size?
我剛來這地方。
如何在不知道文本文件中數據數量的情況下讀取文本文件並將其存儲到數組中?
#include <iostream>
#include <fstream>
using namespace std;
int main(){
ifstream data;
int a[100];
int i=0;
data.open("test.txt");
while(data>>a[i]){
cout << a[i] << endl;
i++;
}
data.close();
}
在我的代碼中,數組大小是固定的。 有沒有不使用<vector>
庫的解決方案? 我可以盡可能大地增加數組的大小,但這似乎不是一個好的解決方案。
最簡單的方法是只使用std::vector
。
如果您不願意這樣做,那么從概念上講,您必須
X
X
數據項。像下面這樣的東西應該可以完成這項工作(注意:我沒有測試代碼)
#include <iostream>
#include <fstream>
using namespace std;
size_t data_size(const string name)
{
size_t c = 0;
ifstream data;
data.open(name);
while(data>>a[i])
c++;
data.close();
return c;
}
int main(){
string name = "test.txt"
int* a = new int [data_size(name)] ;
int i=0;
ifstream data;
data.open("test.txt");
while(data>>a[i]){
cout << a[i] << endl;
i++;
}
data.close();
}
使用std::basic_string
:
#include <iostream>
#include <fstream>
#include <string>
int main(){
ifstream data;
std::string s = "";
data.open("test.txt");
while(data>>s);
data.close();
}
當陣列太小時,您可以擴大陣列。 使用您認為最合適的尺寸,並且只有在給出更多數據時才會放大。
#include <iostream>
#include <fstream>
int main() {
std::ifstream data;
int size = 100;
int* a = new int[size];
int i = 0;
data.open("test.txt");
if (data.is_open()) // check if file is open
return -1;
while (data.peek() != EOF) {
if (i >= size) {
int old_size = size;
size *= 2; // double the size, or what ever. just make it bigger
int* new_array = new int[size]; // create new array with new size
std::copy(a, a + old_size, new_array); // copy data to new array
int* tmp1 = a; // assign old array to a tmp pointer, to not lose the memory
a = new_array; // assign the newly allocated memory to the pointer
delete[] a; a = nullptr; // delete the old memory
}
data >> a[i];
std::cout << a[i] << '\n';
i++;
}
data.close();
// after you don't need the data anymore; delete[]
delete[] a; a = nullptr;
return 0;
}
我學到的一件事是,永遠不要使用using namespace std;
. 您打開了一個非常大的命名空間。 如果你不小心定義了一個 function ,它與std
中的一個同名,你會得到一個編譯器錯誤,這很難得到,它是關於什么的。
另一個忠告,你真的應該看看std::vector
和所有其他 STL 容器。 他們會減輕很多痛苦。
最簡單的方法是計算文件中的元素。 然后分配正確數量的memory。 然后將數據復制到 memory 中。
#include <iterator>
#include <algorithm>
int main()
{
std::ifstream data("test.txt");
std::size_t count = std::distance(std::istream_iterator<int>(data),
std::istream_iterator<int>{});
data.fseek(0); // Post C++11 this works as expected in older version you
// will need to reset the flags as well.
int data = new int[count];
std::copy(std::istream_iterator<int>(data), std::istream_iterator<int>{},
data);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.