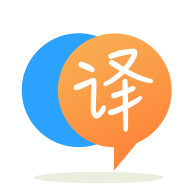
[英]How to toggle class when user selects a list item coming from api in react.s?
[英]How to show an error message to the user in React when no data coming from API
在我的React APP中,我實現了一條錯誤消息,以便在從 API 獲取數據時出現問題。 目標是顯示一條錯誤消息,而不是通知用戶有問題並稍后返回的項目列表。
不幸的是,我無法讓它工作,因為它沒有被稱為showError()
並且控制台中也沒有錯誤,所以我不知道是什么導致問題無法正常工作。
我嘗試這樣做的代碼如下:
import React, {Component} from "react";
import PropTypes from "prop-types";
import {ListGroup, ListGroupItem, ListGroupItemHeading, Container} from "reactstrap";
import {getMoviesInfo} from "../../../Apis/MovieApi";
import {
MdBook,
MdVoiceChat,
MdRecentActors,
MdFlag,
MdMovie,
MdChildCare,
} from "react-icons/md";
import {FaAward, FaCalendarAlt, FaLanguage} from "react-icons/fa";
import {
GiSandsOfTime,
GiFountainPen,
GiDirectorChair,
} from "react-icons/gi";
import Error from "../../../Components/Alert/Error";
export default class MovieDetails extends Component {
state = {
movieInfo: [],
hasErrors: false,
message: "Something went wrong, please refresh yours page or come back later",
};
async componentDidMount() {
await this.onFetchInfo(this.props.movieID);
}
onFetchInfo = async movieID => {
try {
const info = await getMoviesInfo(movieID);
console.log("GETTING IN DETAIL", info);
this.setState({
movieInfo: info,
});
return [];
} catch (err) {
console.log("onFetchInfo err: ", err);
this.onShowErrorMessage(); // here is my error
}
};
onShowErrorMessage = () => {
this.setState({hasErrors: true, loading: false});
setTimeout(this.onClearMessage, 5000);
};
// movieInfo && Object.keys(movieInfo).length !== 0 ?
/* : (
<div>{hasErrors && <Error message={message} />}</div>
)*/
render() {
const {movieInfo, hasErrors, message} = this.state;
return (
<>
<Container>
{hasErrors && <Error message={message} />}
</Container>
<ListGroup className="list-info">
<ListGroupItemHeading>{movieInfo.Title}</ListGroupItemHeading>
<ListGroupItem>
<MdBook />
<span>{movieInfo.Plot}</span>
</ListGroupItem>
<ListGroupItem>
<MdVoiceChat />
<span>{movieInfo.Genre}</span>
</ListGroupItem>
<ListGroupItem>
<GiDirectorChair />
<span>{movieInfo.Director}</span>
</ListGroupItem>
<ListGroupItem>
<MdRecentActors />
<span>{movieInfo.Actors}</span>
</ListGroupItem>
<ListGroupItem>
<GiFountainPen />
<span>{movieInfo.Writer}</span>
</ListGroupItem>
<ListGroupItem>
<MdFlag />
<span>{movieInfo.Country}</span>
</ListGroupItem>
<ListGroupItem>
<FaAward />
<span>{movieInfo.Awards}</span>
</ListGroupItem>
<ListGroupItem>
<FaCalendarAlt />
<span>{movieInfo.Year}</span>
</ListGroupItem>
<ListGroupItem>
<FaLanguage />
<span>{movieInfo.Language}</span>
</ListGroupItem>
<ListGroupItem>
<GiSandsOfTime />
<span>{movieInfo.Runtime}</span>
</ListGroupItem>
<ListGroupItem>
<MdMovie />
<span>{movieInfo.totalSeasons}</span>
</ListGroupItem>
<ListGroupItem>
<MdChildCare />
<span>{movieInfo.Rated}</span>
</ListGroupItem>
</ListGroup>
</>
);
}
}
MovieDetails.propTypes = {
history: PropTypes.any,
info: PropTypes.shape({
Title: PropTypes.string,
Actors: PropTypes.string,
Awards: PropTypes.string,
Country: PropTypes.string,
Genre: PropTypes.string,
Language: PropTypes.string,
Plot: PropTypes.string,
Year: PropTypes.string,
Runtime: PropTypes.string,
totalSeasons: PropTypes.string,
Rated: PropTypes.string,
Writer: PropTypes.string,
Director: PropTypes.string,
}),
movieID: PropTypes.string,
};
當catch()
出現錯誤時,它會向我顯示console.log()
但不會調用該函數來顯示它忽略的錯誤消息,並且無法弄清楚原因。
您可以有條件地使用 JSX 詢問數組或對象是否為空 - console.log("what ever you want") or render( <WhatEverYouWant /> )
問候
如果我的問題是正確的,那是因為 try..catch 是同步工作的,所以你的 catch 塊中的函數沒有運行。 如果異常發生在“預定”代碼中,比如在 setTimeout 中,那么 try..catch 將不會捕獲它:
try {
} catch (e) {
alert( "won't work" );
setTimeout(function() {
noSuchVariable; // script will die here
}, 1000);
}
那是因為當引擎已經離開 try..catch 構造時,函數本身會在稍后執行。
要在預定函數內捕獲異常,try..catch 必須在該函數內:
setTimeout(function() {
try {
noSuchVariable; // try..catch handles the error!
} catch {
alert( "error is caught here!" );
}
}, 1000);
您可以在此博客上獲得有關 try..catch.. 工作的詳細信息
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.