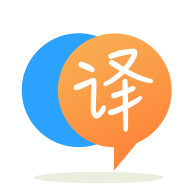
[英]How to update the state of badge counter in Tab Navigator in react-native
[英]How to add badge to tab-bar in react-native?
我正在使用 tabnavigator (createbottomBottomTabNavigator) 並且需要幫助使用 redux 計算百吉餅數量。
您始終可以創建自定義組件,在本例中為選項卡項 ( <TabBarItem />
)。
我創建了一個簡單的演示,這里是小吃鏈接: https : //snack.expo.io/@abranhe/tab-badge-count-redux
在此示例中,您有 3 個頁面/選項卡(應用程序、導航和配置文件),因此您需要管理每個頁面/選項卡的通知計數器。 所以我們從創建組件開始,我使用Native Base來預構建組件。 此外,我們有以下初始狀態。
export default {
apps: {
notificationCount: 7,
},
navigation: {
notificationCount: 0,
},
profile: {
notificationCount: 3,
},
};
TabBarItem.js
import React from 'react';
import { connect } from 'react-redux';
import { View, StyleSheet } from 'react-native';
import { Badge, Button, Icon, Text } from 'native-base';
const renderBadge = ({ badgeProps, counter }) => (
<Badge {...badgeProps} style={styles.badge}>
<Text style={styles.notificationText}>{counter}</Text>
</Badge>
);
function TabBarItem({ notificationCount, badgeProps, icon, label, color }) {
let counter = 0;
switch (icon) {
case 'apps':
counter = notificationCount.apps;
break;
case 'navigate':
counter = notificationCount.navigation;
break;
case 'person':
counter = notificationCount.profile;
break;
default:
counter = 0;
}
return (
<View style={styles.container}>
{counter > 0 && renderBadge({ counter, badgeProps })}
<View style={styles.iconContainer}>
<Icon name={icon} style={{ color }} />
<Text style={{ color, ...styles.label }}>{label}</Text>
</View>
</View>
);
}
const styles = ....;
const mapStateToProps = state => ({
notificationCount: {
apps: state.apps.notificationCount,
navigation: state.navigation.notificationCount,
profile: state.profile.notificationCount,
},
});
export default connect(mapStateToProps)(TabBarItem);
這只是一種解決方法,正如您所看到的,我正在創建一個連接到 Redux 的組件,並根據圖標名稱道具檢查應呈現哪個選項卡。 如果我們只有一個帶有通知的選項卡,我們只需要訪問 Redux 上的數據,並自動呈現。
我們需要創建一個 reducer 來處理 Increment 和 Decrement 動作
export default function reducer(state = initialState, action = {}) {
switch (action.type) {
case 'APPS.INCREMENT':
return {
...state,
apps: {
...state.apps,
notificationCount: state.apps.notificationCount + 1,
},
};
case 'APPS.DECREMENT':
return {
...state,
apps: {
...state.apps,
notificationCount: state.apps.notificationCount - 1,
},
};
...
}
我們還需要添加創建的組件:
import React from 'react';
import { connect } from 'react-redux';
import { StyleSheet } from 'react-native';
import { Container, Content, H1, H3 } from 'native-base';
import NotificationCounter from '../components/NotificationCounter';
const Apps = ({ notificationCount, dispatch }) => (
<Container>
<Content contentContainerStyle={styles.container}>
<H1>Apps Page</H1>
<NotificationCounter
handleIncrement={() => dispatch({ type: 'APPS.INCREMENT' })}
handleDecrement={() => dispatch({ type: 'APPS.DECREMENT' })}
/>
<H3>Notification Count: {notificationCount}</H3>
</Content>
</Container>
);
const styles = ...
const mapStateToProps = state => ({
notificationCount: state.apps.notificationCount,
});
export default connect(mapStateToProps)(Apps);
我們正在使用dispatch({ type: 'APPS.INCREMENT' })
來觸發增加應用程序選項卡通知的操作。
<NotificationCounter />
組件代碼:
import React from 'react';
import { View, StyleSheet } from 'react-native';
import { Button, Text } from 'native-base';
export default ({ handleDecrement, handleIncrement }) => (
<View style={styles.container}>
<Button onPress={handleDecrement}>
<Text>Decrement</Text>
</Button>
<Button onPress={handleIncrement}>
<Text>Increment</Text>
</Button>
</View>
);
const styles = ...
使用 redux 有一種自定義方法可以做到這一點,您可以使用相同的方法制作自定義組件:-
screen: NotificationScreen,
navigationOptions: {
tabBar: (state, acc) => ({
icon: ({ tintColor, focused }) => (
<BadgeTabIcon
iconName="notification"
size={26}
selected={focused}
/>
),
visible: (acc && acc.visible !== 'undefined') ? acc.visible : true,
}),
},
},
在哪里,
export default connect(state => ({
notificationCount: state.notifications.count,
}))(({ dispatch, nav }) => (
<View>
<TabIcon {...props} />
{
props.notificationCount > 0 ?
<View style={{ position: 'absolute', right: 10, top: 5, backgroundColor: 'red', borderRadius: 9, width: 18, height: 18, justifyContent: 'center', alignItems: 'center' }}>
<Text style={{ color: 'white' }}>{props.notificationCount}</Text>
</View> : null
}
</View>
));
在這里閱讀更多
此外,react native 中的官方 tabnavigation 也有同樣的支持,你可以在這里閱讀更多
我希望這會有所幫助...謝謝:)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.