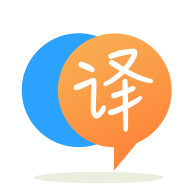
[英]How do I initialise a std::array field inside a struct with the contents of a variable length sequence container?
[英]How do I initialise a const array struct field with the output of a function?
我有一個包含 const 數組的結構,並希望在構造時將其初始化為特定值。 不幸的是,它的內容取決於作為參數傳遞給構造函數的幾個參數,並且需要一個函數來計算數組的內容。
我理想中想做的事情是這樣的:
struct SomeType {
const unsigned int listOfValues[32];
unsigned int[32] processParameters(unsigned int parameter) {
unsigned int arrayValues[32];
for(int i = 0; i < 32; i++) {
arrayValues[i] = i * parameter;
}
return arrayValues;
}
SomeType(unsigned int parameter) : listOfValues(processParameters(parameter)) {
}
};
當然這里有幾個問題(從函數返回數組是不可能的,數據類型不匹配等)。 然而,有沒有什么辦法,這是可能的嗎?
我已經看到其他類似的問題建議為此使用 std::vector,但是由此產生的堆分配是我的性能預算無法承受的。
正如 Nathan 建議的那樣,您應該使用std::array
更改原始std::array
。 這樣您仍然可以享受堆棧分配的好處,但現在您可以從副本進行初始化。
using MyArray = std::array<unsigned int, 32>;
const MyArray listOfValues;
MyArray processParameters(unsigned int parameter) {
MyArray arrayValues;
for(int i = 0; i < 32; i++) {
arrayValues[i] = i * parameter;
}
return arrayValues;
}
我從數組數據類型中刪除了 const ,因為它不是必需的,因為您的數組已經是 const ,而且使用 const unsigned int 您將無法在運行時設置arrayValues
的值。
這是否符合您的目的? 沒有我能看到的堆分配。
struct SomeType {
const unsigned int *listOfValues;
const unsigned int * processParameters(unsigned int parameter) {
for(int i = 0; i < 32; i++) {
_listOfValues[i] = i * parameter;
}
return _listOfValues;
}
SomeType(unsigned int parameter) :
listOfValues(processParameters(parameter))
{
}
private:
unsigned int _listOfValues[32];
};
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.