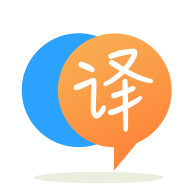
[英]Java, Count number of students who take the same course as a method in Student Class
[英]Java - How to find students with their highest marks writing a method in a Student class?
我是 Java 的初學者,並且陷入了一項任務。 如果有人能幫助我,我將不勝感激。 我有一個具有這些屬性的班級學生 - 姓名、化學分數、數學分數和物理分數。 在 Main 類中,我與幾個學生一起創建了一個 ArrayList。 我的任務是找出所有學生中化學分數最高、數學和物理分數最高的學生(他們的名字)。 我在 Main 類的 main 方法中這樣做了,但問題是我必須在 Student 的類中編寫它,而此時我沒有這樣做。
這是我的代碼:
public class Student {
protected String name;
private int chemistry;
private int mathematics;
private int physics;
public Student(String name, int chemistry, int mathematics, int physics) {
this.name = name;
this.chemistry = chemistry;
this.mathematics = mathematics;
this.physics = physics;
}
public int getChemistry() {
return chemistry;
}
public void setChemistry(int chemistry) {
this.chemistry = chemistry;
}
public int getMathematics() {
return mathematics;
}
public void setMathematics(int mathematics) {
this.mathematics = mathematics;
}
public int getPhysics() {
return physics;
}
public void setPhysics(int physics) {
this.physics = physics;
}
}
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
Student tom = new Student("Tom", 7, 6, 4);
Student rome = new Student("Rome", 9, 5, 8);
Student jack = new Student("Jack", 6, 9, 8);
Student simon = new Student("Simon", 10, 8, 5);
Student darek = new Student("Darek", 10, 9, 8);
ArrayList<Student> students = new ArrayList<>();
students.add(tom);
students.add(rome);
students.add(jack);
students.add(simon);
students.add(darek);
System.out.println("Student(s) with the highest Chemistry grade among all students:");
int max = 0;
String names = null;
for (int i = 0; i < students.size(); i++) {
if (max == students.get(i).getChemistry()) {
names += ", " + students.get(i).name;
} else if (max < students.get(i).getChemistry()) {
max = students.get(i).getChemistry();
names = students.get(i).name;
}
}
System.out.println(names);
System.out.println();
System.out.println("Student(s) with the highest Mathematics grade among all students:");
max = 0;
names = null;
for (int i = 0; i < students.size(); i++) {
if (max == students.get(i).getMathematics()) {
names += ", " + students.get(i).name;
} else if (max < students.get(i).getMathematics()) {
max = students.get(i).getMathematics();
names = students.get(i).name;
}
}
System.out.println(names);
System.out.println();
System.out.println("Student(s) with the highest Physics grade among all students:");
max = 0;
names = null;
for (int i = 0; i < students.size(); i++) {
if (max == students.get(i).getPhysics()) {
names += ", " + students.get(i).name;
} else if (max < students.get(i).getPhysics()) {
max = students.get(i).getPhysics();
names = students.get(i).name;
}
}
System.out.println(names);
}
}
我嘗試在 Student 類中編寫類似的方法:
public int bestOfChemistry(int max, String names) {
if (max == chemistry) {
names += ", " + name;
} else if (max < chemistry) {
max = chemistry;
names = name;
}
return max;
}
但是當我嘗試在 Main 類中使用此方法時,我只能獲得最高等級。 我知道,因為我只在方法 bestOfChemistry(..) 中返回了它,但我也不知道如何獲取它們的名字:
int max = 0;
String names = null;
for (int i = 0; i < students.size(); i++) {
max = students.get(i).bestOfChemistry(max, names);
}
System.out.println(max);
我也不知道如何為這三個課程編寫一個方法來避免使用幾種相同的方法。
您不應該在表示單個對象的類中創建引用多個對象的方法,這在語義上沒有意義。
編輯:由於您不允許在主課程中這樣做,您可以添加一個新課程,其功能是搜索最好的學生。 例如,我將其命名為“StudentsFinder”。 它看起來像這樣:
class StudentsFinder {
private final ArrayList<Student> students;
private ArrayList<String> bestStudents;
StudentsFinder(ArrayList<Student> students) {
this.students = students;
}
ArrayList<String> getBestChemistryStudents() {
bestStudents = new ArrayList<>();
int maxChemistryGrade = 0;
//We look for the best grade first.
for (Student student : students) {
if (student.getChemistry() > maxChemistryGrade) {
maxChemistryGrade = student.getChemistry();
}
}
//Now we can add those students with the best grade in the array
for (Student student : students) {
if (student.getChemistry() == maxChemistryGrade) {
bestStudents.add(student.getName());
}
}
//And we return the results
return bestStudents;
}
//The following methods do the same thing but with math and physics grades, respectively
ArrayList<String> getBestMathStudents() {
bestStudents = new ArrayList<>();
int maxMathGrade = 0;
for (Student student : students) {
if (student.getMathematics() > maxMathGrade) {
maxMathGrade = student.getMathematics();
}
}
for (Student student : students) {
if (student.getMathematics() == maxMathGrade) {
bestStudents.add(student.getName());
}
}
return bestStudents;
}
ArrayList<String> getBestPhysicsStudents() {
bestStudents = new ArrayList<>();
int maxPhysicsGrade = 0;
for (Student student : students) {
if (student.getPhysics() > maxPhysicsGrade) {
maxPhysicsGrade = student.getPhysics();
}
}
for (Student student : students) {
if (student.getPhysics() == maxPhysicsGrade) {
bestStudents.add(student.getName());
}
}
return bestStudents;
}
}
在 Student 類中,您將需要名稱的吸氣劑:
public String getName() {
return name;
}
然后,您可以在主類中添加一個新的 StudentsFinder 實例,在構造函數中將學生數組傳遞給它,並調用每個方法:
StudentsFinder finder = new StudentsFinder(students);
System.out.println("Student(s) with the highest Chemistry grade among all students:");
System.out.println(finder.getBestChemistryStudents());
System.out.println("Student(s) with the highest Mathematics grade among all students:");
System.out.println(finder.getBestMathStudents());
System.out.println("Student(s) with the highest Physics grade among all students:");
System.out.println(finder.getBestPhysicsStudents());
請注意,我們可以將結果直接傳遞給println()
方法:
System.out.println(finder.getBestChemistryStudents());
...因為它會自動調用ArrayList
中的toString()
,其實現是從AbstractCollection
類繼承的。 它以[value1, value2, ..., valueN]
格式打印所有值。
我猜你有一些設計問題。 Class 是一個藍圖,您將在其上初始化一個對象,在這種情況下,每個 Student 對象都是一個單獨的學生,它具有名稱、化學、物理、數學屬性。 在 Student 類中使用一種方法來為您提供具有最高分的學生姓名列表是沒有意義的。 因此,我的建議是創建一個 Classroom、Course 或 School 類,這些類將具有諸如學生列表之類的屬性。 在那個班級中,獲取最高分學生名單的功能是有意義的,因為每個教室都可以說你可以找到它。 希望它會幫助你。
public enum Subject{
CHEMISTRY,
MATH,
PHYSICS;
}
public String findNameOfTheBest(List<Student> students, Subject subject){
switch(subject){
case CHEMISTRY:
return students.stream().sorted(Comparator.comparingInt(Student::getChemistry).reversed()).findFirst().map(Student::getName).get();
case MATH:
return students.stream().sorted(Comparator.comparingInt(Student::getMathematics).reversed()).findFirst().map(Student::getName).get();
case PHYSICS:
return students.stream().sorted(Comparator.comparingInt(Student::getPhysics).reversed()).findFirst().map(Student::getName).get();
default:
throw new Exception("unknown subject type");
}
}
只需使用不同的主題類型調用此方法並將返回值分配給變量。 一個簡短的解釋:它按屬性之一對列表中的每個學生對象進行排序,根據您傳入的主題,可以是化學、數學或物理(例如 Subject.CHEMISTRY)。 然后顛倒順序,使最高標記位於列表的最開頭。 然后它調用 findFirst() ,它返回一個 Optional。 在這個 Optional 上調用了一個 map 方法,它基本上將元素從 Student 對象轉換為其屬性“名稱”。
編輯:這是考慮到具有最高成績的多個學生的可能性的調整方法。
public List<String> findNameOfTheBest(List<Student> students, Subject subject){
switch(subject){
case CHEMISTRY:
int highestMark = students.stream().max(Comparator.comparingInt(Student::getChemistry)).get();
return students.stream().filter(student -> student.getChemistry == highestMark).map(Student::getName).collect(Collectors.toList());
case MATH:
int highestMark = students.stream().max(Comparator.comparingInt(Student::getMathematics)).get();
return students.stream().filter(student -> student.getMathematics == highestMark).map(Student::getName).collect(Collectors.toList());
case PHYSICS:
int highestMark = students.stream().max(Comparator.comparingInt(Student::getPhysics)).get();
return students.stream().filter(student -> student.getPhysics == highestMark).map(Student::getName).collect(Collectors.toList());
default:
throw new Exception("unknown subject type");
}
}
最簡單的方法:
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
Student tom = new Student("Tom", 7, 6, 4);
Student rome = new Student("Rome", 9, 5, 8);
Student jack = new Student("Jack", 6, 9, 8);
Student simon = new Student("Simon", 10, 8, 5);
Student darek = new Student("Darek", 10, 9, 8);
derek.bestChem();
derek.bestPhys();
derek.bestMath();
}
public class Student {
private String name;
private int chemistry;
private int mathematics;
private int physics;
public static ArrayList<Student> students= new ArrayList<Student>();
public Student(String name, int chemistry, int mathematics, int physics) {
this.name = name;
this.chemistry = chemistry;
this.mathematics = mathematics;
this.physics = physics;
students.add(this);
}
public int getChemistry() {
return chemistry;
}
public void setChemistry(int chemistry) {
this.chemistry = chemistry;
}
public int getMathematics() {
return mathematics;
}
public void setMathematics(int mathematics) {
this.mathematics = mathematics;
}
public int getPhysics() {
return physics;
}
public void setPhysics(int physics) {
this.physics = physics;
}
public String getName(){
return name;
}
public void bestChem(){
int x=-2;
String y="";
for (int j=0; j< students.size(); j++){
if (students.get(j).getChemistry()>x) {
x=students.get(j).getChemistry();
y=students.get(j).getName();
}
}
System.out.println("Student(s) with the highest Chemistry grade among all students:" + y);
}
public void bestPhys(){
int x=-2;
String y="";
for (int j=0; j< students.size(); j++){
if (students.get(j).getPhysics()>x) {
x=students.get(j).getPhysics();
y=students.get(j).getName();
}
}
System.out.println("Student(s) with the highest Physics grade among all students:" + y);
}
public void bestMath(){
int x=-2;
String y="";
for (int j=0; j<students.size(); j++){
if (students.get(j).getMathematics()>x) {
x=students.get(j).getMathematics();
y=students.get(j).getName();
}
}
System.out.println("Student(s) with the highest Mathematics grade among all students:" + y);
}
}
我是Java的初學者,被困在一項任務中。 如果有人可以幫助我,我將非常感激。 我有一個具有以下屬性的班級學生-名稱,化學標記,數學標記和物理標記。 在主課程中,我創建了一個包含多個學生的ArrayList。 我的任務是尋找所有學生中化學得分最高,數學和物理得分最高的學生(他們的名字)。 我是在Main類main方法中完成此操作的,但問題是我必須在Student的班級中編寫此代碼,但此時我做不到。
這是我的代碼:
public class Student {
protected String name;
private int chemistry;
private int mathematics;
private int physics;
public Student(String name, int chemistry, int mathematics, int physics) {
this.name = name;
this.chemistry = chemistry;
this.mathematics = mathematics;
this.physics = physics;
}
public int getChemistry() {
return chemistry;
}
public void setChemistry(int chemistry) {
this.chemistry = chemistry;
}
public int getMathematics() {
return mathematics;
}
public void setMathematics(int mathematics) {
this.mathematics = mathematics;
}
public int getPhysics() {
return physics;
}
public void setPhysics(int physics) {
this.physics = physics;
}
}
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
Student tom = new Student("Tom", 7, 6, 4);
Student rome = new Student("Rome", 9, 5, 8);
Student jack = new Student("Jack", 6, 9, 8);
Student simon = new Student("Simon", 10, 8, 5);
Student darek = new Student("Darek", 10, 9, 8);
ArrayList<Student> students = new ArrayList<>();
students.add(tom);
students.add(rome);
students.add(jack);
students.add(simon);
students.add(darek);
System.out.println("Student(s) with the highest Chemistry grade among all students:");
int max = 0;
String names = null;
for (int i = 0; i < students.size(); i++) {
if (max == students.get(i).getChemistry()) {
names += ", " + students.get(i).name;
} else if (max < students.get(i).getChemistry()) {
max = students.get(i).getChemistry();
names = students.get(i).name;
}
}
System.out.println(names);
System.out.println();
System.out.println("Student(s) with the highest Mathematics grade among all students:");
max = 0;
names = null;
for (int i = 0; i < students.size(); i++) {
if (max == students.get(i).getMathematics()) {
names += ", " + students.get(i).name;
} else if (max < students.get(i).getMathematics()) {
max = students.get(i).getMathematics();
names = students.get(i).name;
}
}
System.out.println(names);
System.out.println();
System.out.println("Student(s) with the highest Physics grade among all students:");
max = 0;
names = null;
for (int i = 0; i < students.size(); i++) {
if (max == students.get(i).getPhysics()) {
names += ", " + students.get(i).name;
} else if (max < students.get(i).getPhysics()) {
max = students.get(i).getPhysics();
names = students.get(i).name;
}
}
System.out.println(names);
}
}
我試圖在Student類中編寫類似的方法:
public int bestOfChemistry(int max, String names) {
if (max == chemistry) {
names += ", " + name;
} else if (max < chemistry) {
max = chemistry;
names = name;
}
return max;
}
但是,當我嘗試在Main類中使用此方法時,我只能獲得最高成績。 我知道,因為我只在bestOfChemistry(..)方法中返回了它,但是我也不知道如何獲得它們的名字:
int max = 0;
String names = null;
for (int i = 0; i < students.size(); i++) {
max = students.get(i).bestOfChemistry(max, names);
}
System.out.println(max);
我也不知道如何為這三個課程編寫一種方法,以避免幾種相同的方法。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.