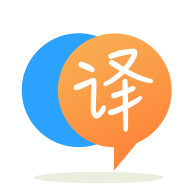
[英]Python Tkinter Canvas Many create_window() Items Not Scrolling with Scrollbar
[英]Tkinter scrollbar works only with create_window
我有一個窗格窗口,在右側窗格中,我在畫布中有一個要滾動的框架。 只有當我調用畫布create_window
函數時,我才能讓它滾動。 當我調用create_window
一切正常,除了內容框架沒有展開並且我已將網格設置為sticky=nsew
。 在示例代碼中有一個名為 x 的變量,如果 x 設置為 1,則框架展開並且滾動條正確顯示但它們不起作用,如果設置為 0 滾動條工作但框架不展開。 如果 x 設置為 1 或 0,我需要滾動條工作。您需要調整窗口大小以查看此問題,請注意分隔符小部件會擴展以填充框架,但當 x 設置為時滾動條不會滾動任何內容1.
我就在那里我讓它工作 99% 只是一個小故障。 當窗口高度小於內容且窗口寬度大於內容時,分隔符小部件不會擴展以填充內容框架。 我更新了代碼並添加了背景顏色以突出顯示問題。
import tkinter as tk
import tkinter.ttk as ttk
lorem_ipsum = 'Lorem ipsum dolor sit amet, luctus non. Litora viverra ligula'
class Scrollbar(ttk.Scrollbar):
def __init__(self, parent, canvas, **kwargs):
ttk.Scrollbar.__init__(self, parent, **kwargs)
command = canvas.xview if kwargs.get('orient', tk.VERTICAL) == tk.HORIZONTAL else canvas.yview
self.configure(command=command)
def set(self, low, high):
if float(low) > 0 or float(high) < 1:
self.grid()
else:
self.grid_remove()
ttk.Scrollbar.set(self, low, high)
class App(tk.Tk):
def __init__(self):
super().__init__()
self.rowconfigure(0, weight=1)
self.columnconfigure(0, weight=1)
self.title('Paned Window Demo')
self.geometry('420x200')
style = ttk.Style()
style.theme_use('clam')
style.configure('TPanedwindow', background='black')
pw = ttk.PanedWindow(self, orient=tk.HORIZONTAL)
left_frame = ttk.Frame(pw)
right_frame = ttk.Frame(pw)
ttk.Label(left_frame, text='Left Pane').grid()
left_frame.rowconfigure(0, weight=1)
left_frame.columnconfigure(0, weight=1)
left_frame.grid(sticky=tk.NSEW)
right_frame.rowconfigure(0, weight=1)
right_frame.columnconfigure(0, weight=1)
right_frame.grid(sticky=tk.NSEW)
pw.add(left_frame)
pw.add(right_frame)
pw.grid(sticky=tk.NSEW)
canvas = tk.Canvas(right_frame, bg=style.lookup('TFrame', 'background'))
canvas.frame = ttk.Frame(canvas)
canvas.rowconfigure(0, weight=1)
canvas.columnconfigure(0, weight=1)
canvas.grid(sticky=tk.NSEW)
canvas.frame.rowconfigure(990, weight=1)
canvas.frame.columnconfigure(0, weight=1)
canvas.frame.grid(sticky=tk.NSEW)
content = tk.Frame(canvas.frame, bg='blue')
content.rowconfigure(0, weight=1)
content.columnconfigure(0, weight=1)
content.grid(sticky=tk.NSEW)
xscroll = Scrollbar(right_frame, canvas, orient=tk.HORIZONTAL)
yscroll = Scrollbar(right_frame, canvas, orient=tk.VERTICAL)
xscroll.grid(row=990, column=0, sticky=tk.EW)
yscroll.grid(row=0, column=990, sticky=tk.NS)
for idx in range(1, 11):
tk.Label(content, bg='#aaaaaa', fg='#000000', text=f'{idx} {lorem_ipsum}').grid(sticky=tk.NW)
ttk.Separator(content, orient=tk.HORIZONTAL).grid(pady=10, sticky=tk.EW)
for idx in range(11, 21):
tk.Label(content, bg='#aaaaaa', fg='#000000', text=f'{idx} {lorem_ipsum}').grid(sticky=tk.NW)
self.window = canvas.create_window((0, 0), window=canvas.frame, anchor=tk.NW)
self.update_idletasks()
pw.sashpos(0, newpos=100)
def update_canvas(event):
content.update_idletasks()
_, _, width, height = content.bbox(tk.ALL)
if event.width < width or event.height < height:
if not self.window:
self.window = canvas.create_window((0, 0), window=canvas.frame, anchor=tk.NW)
else:
self.window = None
canvas.frame.grid(sticky=tk.NSEW)
canvas.bind('<Configure>', update_canvas)
canvas.configure(scrollregion=content.bbox(tk.ALL))
canvas.configure(xscrollcommand=xscroll.set, yscrollcommand=yscroll.set)
def main():
app = App()
app.mainloop()
if __name__ == '__main__':
main()
滾動條僅與canvas.create_window()
一起使用是完全正常的,因為這是在畫布內顯示小部件的適當方法,以便畫布知道小部件的大小。 否則,您只是將畫布用作框架。 但是, canvas.create_window()
方法沒有sticky
選項,因此您必須在畫布更改大小時手動更改小部件的寬度。
因此,在您的update_canvas
回調中,如果畫布的寬度大於內容所需的寬度,則每次畫布更改寬度時都需要更改窗口的寬度:
def update_canvas(event):
# if the new canvas's width (event.width) is larger than the content's
# minimum width (content.winfo_reqwidth()) then make canvas.frame the
# same width as the canvas
if event.width > content.winfo_reqwidth():
canvas.itemconfigure(self.window, width=event.width)
此外,您的代碼中有一些無用的行,特別是您不必網格canvas.frame
因為您使用canvas.create_window()
顯示它,見下文:
class App(tk.Tk):
def __init__(self):
super().__init__()
self.rowconfigure(0, weight=1)
self.columnconfigure(0, weight=1)
self.title('Paned Window Demo')
self.geometry('420x200')
style = ttk.Style()
style.theme_use('clam')
style.configure('TPanedwindow', background='black')
pw = ttk.PanedWindow(self, orient=tk.HORIZONTAL)
left_frame = ttk.Frame(pw)
right_frame = ttk.Frame(pw)
ttk.Label(left_frame, text='Left Pane').grid()
left_frame.rowconfigure(0, weight=1)
left_frame.columnconfigure(0, weight=1)
left_frame.grid(sticky=tk.NSEW)
right_frame.rowconfigure(0, weight=1)
right_frame.columnconfigure(0, weight=1)
right_frame.grid(sticky=tk.NSEW)
pw.add(left_frame)
pw.add(right_frame)
pw.grid(sticky=tk.NSEW)
canvas = tk.Canvas(right_frame, bg=style.lookup('TFrame', 'background'))
canvas.frame = ttk.Frame(canvas)
# canvas.rowconfigure(0, weight=1) -> useless since no widget will be gridded in the canvas
# canvas.columnconfigure(0, weight=1) -> useless since no widget will be gridded in the canvas
canvas.grid(sticky=tk.NSEW)
canvas.frame.rowconfigure(990, weight=1)
canvas.frame.columnconfigure(0, weight=1)
# canvas.frame.grid(sticky=tk.NSEW) -> no need to grid canvas.frame since it is displayed it with canvas.create_window()
content = tk.Frame(canvas.frame, bg='blue')
content.rowconfigure(0, weight=1)
content.columnconfigure(0, weight=1)
content.grid(sticky=tk.NSEW)
xscroll = Scrollbar(right_frame, canvas, orient=tk.HORIZONTAL)
yscroll = Scrollbar(right_frame, canvas, orient=tk.VERTICAL)
xscroll.grid(row=990, column=0, sticky=tk.EW)
yscroll.grid(row=0, column=990, sticky=tk.NS)
for idx in range(1, 11):
tk.Label(content, bg='#aaaaaa', fg='#000000', text=f'{idx} {lorem_ipsum}').grid(sticky=tk.NW)
ttk.Separator(content, orient=tk.HORIZONTAL).grid(pady=10, sticky=tk.EW)
for idx in range(11, 21):
tk.Label(content, bg='#aaaaaa', fg='#000000', text=f'{idx} {lorem_ipsum}').grid(sticky=tk.NW)
self.window = canvas.create_window((0, 0), window=canvas.frame, anchor=tk.NW)
self.update_idletasks()
pw.sashpos(0, newpos=100)
def update_canvas(event):
# if the new canvas's width (event.width) is larger than the content's
# minimum width (content.winfo_reqwidth()) then make canvas.frame the
# same width as the canvas
if event.width > content.winfo_reqwidth():
canvas.itemconfigure(self.window, width=event.width)
canvas.bind('<Configure>', update_canvas)
canvas.configure(scrollregion=content.bbox(tk.ALL))
canvas.configure(xscrollcommand=xscroll.set, yscrollcommand=yscroll.set)
def main():
app = App()
app.mainloop()
if __name__ == '__main__':
main()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.