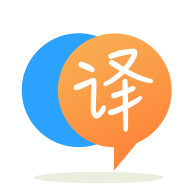
[英]Regarding structs in C, how to copy the data from a struct to a string array
[英]How do i copy a struct from an array of structs?
我意識到所有的printf
都會變得有點長,但我真的不知道如何更好地呈現我的問題。
我在 c 中正確初始化結構時遇到問題。 我試過用printf
調試來顯示我的問題。 本質上,我正在使用以下函數創建一個結構列表:
Vertex *Vertex_new(int n){
Vertex *vert = malloc(sizeof(Vertex));
if(!vert){return NULL;}
vert->id = n;
vert->outNeighbours = malloc(sizeof(LinkedList));
vert->outNeighbours = LinkedList_new();
vert->inNeighbours = malloc(sizeof(LinkedList));
vert->inNeighbours = LinkedList_new();
return vert;
}
struct Vertex {
int id; // a number in [0; numVertices[
LinkedList *outNeighbours; // A linked list of vertices.
LinkedList *inNeighbours; // A linked list of vertices
};
這用於創建以下結構,然后是函數。 之后的函數稱為Graph_new()
,我在保存Vertex
遇到問題,正確地在列表中。 我不知道我做錯了哪一部分,但我假設這與我如何初始化列表有關,因此做了一堆打印語句來嘗試理解問題:
struct Graph {
int numVertices;
int numEdges;
Vertex *vertices; // An array of numVertices vertices
};
Graph *Graph_new(int n){
Graph *grf = malloc(sizeof(Graph));
if(!grf){return NULL;}
grf->numEdges = 0;
grf->numVertices = n;
Vertex list[n];
grf->vertices = malloc(sizeof(Vertex*)*n);
for(int i = 0; i < n;i++){
list[i] = *Vertex_new(i);
}
grf->vertices = list;
// My attempt at debugging, `grf` is simply returned after these print statements:
printf("Inside graph_new for list:\n");
printf("%d ",list[0].id);
printf("%d ",list[1].id);
printf("%d ",list[2].id);
printf("%d ",list[3].id);
printf("%d \n",list[4].id);
// Det her virker herinde, men ikke uden for
// er der et problem i hvordan jeg gemmer listen?
printf("Inside graph_new for grf->vertices:\n");
printf("%d ",grf->vertices[0].id);
printf("%d ",grf->vertices[1].id);
printf("%d ",grf->vertices[2].id);
printf("%d ",grf->vertices[3].id);
printf("%d \n",grf->vertices[4].id);
printf("%d ",grf->vertices[0].id);
printf("%d ",grf->vertices[1].id);
printf("%d ",grf->vertices[2].id);
printf("%d ",grf->vertices[3].id);
printf("%d \n",grf->vertices[4].id);
return grf;
}
在另一個函數中,我調用graph_new(n)
。 n
是從文件中讀取的,在這種情況下n
是 5,我已經測試過,以確保它正確讀取它,所以它是有保證的 5。我測試了兩種打印方式,以了解發生了什么。 首先:
Graph *newG = Graph_new(n);
// outside of graph_new:
printf("outside of graph_new, newG->vertices:\n");
printf("%d ",newG->vertices[0].id);
printf("%d ",newG->vertices[1].id);
printf("%d ",newG->vertices[2].id);
printf("%d ",newG->vertices[3].id);
printf("%d \n",newG->vertices[4].id);
printf("%d ",newG->vertices[0].id);
printf("%d ",newG->vertices[1].id);
printf("%d ",newG->vertices[2].id);
printf("%d ",newG->vertices[3].id);
printf("%d \n",newG->vertices[4].id);
給出輸出:
Inside graph_new for list:
0 1 2 3 4
Inside graph_new for grf->vertices:
0 1 2 3 4
0 1 2 3 4
outside of graph_new, newG->vertices:
0 -285208794 -603392624 -603392624 0
0 -285208794 -603392624 -603392624 0
所以起初我認為這是因為列表沒有正確保存,或者列表中的頂點沒有正確保存。
但是,如果我然后在Graph_new
之外打印它:
Vertex vert1 = newG->vertices[0];
Vertex vert2 = newG->vertices[1];
Vertex vert3 = newG->vertices[2];
Vertex vert4 = newG->vertices[3];
Vertex vert5 = newG->vertices[4];
printf("works outside:\n");
printf("%d ", vert1.id);
printf("%d ", vert2.id);
printf("%d ", vert3.id);
printf("%d ", vert4.id);
printf("%d \n", vert5.id);
// Saving the vertices back in the array works
newG->vertices[0] = vert1;
newG->vertices[1] = vert2;
newG->vertices[2] = vert3;
newG->vertices[3] = vert4;
newG->vertices[4] = vert5;
Vertex vert11 = newG->vertices[0];
Vertex vert22 = newG->vertices[1];
Vertex vert33 = newG->vertices[2];
Vertex vert44 = newG->vertices[3];
Vertex vert55 = newG->vertices[4];
printf("Works again\n");
printf("%d ", vert11.id);
printf("%d ", vert22.id);
printf("%d ", vert33.id);
printf("%d ", vert44.id);
printf("%d \n", vert55.id);
// ------------------------------
printf("Doesn't work: \n");
Vertex vvert1 = newG->vertices[0];
Vertex vvert2 = newG->vertices[1];
Vertex vvert3 = newG->vertices[2];
Vertex vvert4 = newG->vertices[3];
Vertex vvert5 = newG->vertices[4];
printf("%d ", vvert1.id);
printf("%d ", vvert2.id);
printf("%d ", vvert3.id);
printf("%d ", vvert4.id);
printf("%d \n", vvert5.id);
我得到以下輸出:
Inside graph_new for list:
0 1 2 3 4
Inside graph_new for grf->vertices:
0 1 2 3 4
0 1 2 3 4
works outside:
0 1 2 3 4
Works again
0 1 2 3 4
Doesn't work:
0 -2035597530 519880720 -2031896736 -1398417392
如果有人有時間了解它並伸出援助之手,我將不勝感激。 我可能無法及時參加考試,但至少我知道我做錯了什么。
Graph *Graph_new(int n){
…
Vertex list[n];
grf->vertices = malloc(sizeof(Vertex*)*n);
for(int i = 0; i < n;i++){
list[i] = *Vertex_new(i);
}
grf->vertices = list;
…
return grf;
}
list
數組是Graph_new
函數的本地數組,一旦控制退出函數就會被銷毀,因此您不能從Graph_new
引用list
,這是未定義的行為。
還有*Vertex_new(i);
有內存泄漏,因為您正在失去對分配的引用。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.