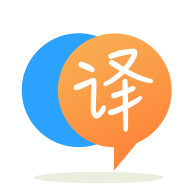
[英]What should happen when the return value from a C++ function that returns a reference of an undefined object type is not assigned?
[英]For a search algo with reference return type, what should be the default return value?
假設我有一個數組,我想檢索對該數組元素的引用。
struct A {
int n;
}
A& search_algo(A* AList, int len, int target) {
for (int i = 0; i < len; i++) {
if (AList[i].n == target) { return AList[i]; }
}
return what? //problem comes here, target not in array, what should I return
}
我想知道處理它的最常規方法是什么,或者什么返回值最有意義。 就像我怎樣才能最好地傳達“你的東西不在這里,走開”的信息。 類似於nullptr
東西會很棒。
我目前的解決方案是在堆棧上初始化一個對象A
並返回它。 雖然我可以編譯得很好,但是返回對局部變量的引用是不安全的。
我正在考慮使用new
在堆上初始化對象,但這會很麻煩,我將不得不處理內存釋放。 我不喜歡。
一個好的做法是返回找到元素的索引/位置,而不是返回找到的值。 這就是STL
所做的,它返回找到的元素的位置/迭代器,如果未找到該元素,則返回最后一個元素之前 1 個單位的位置,這表明在容器中找不到該元素。 如果在數組中找不到該元素,則可以返回len
。 例如,
#include <iostream>
#include <string>
#include <fstream>
using namespace std;
struct A {
int n;
};
int search_algo(A* AList, int len, int target) {
for (int i = 0; i < len; i++)
if (AList[i].n == target)
return i;
return len;
}
int main(){
int _len = 4;
A _list[_len] = {6,7,8,9};
int idx1 = search_algo(_list,_len,7);
int idx2 = search_algo(_list,_len,10);
if(idx1==_len)
cout<<"Element not found"<<endl;
else
cout<<"Element found at "<<idx1<<" index and it's value is "<<_list[idx1].n<<endl;
if(idx2==_len)
cout<<"Element not found"<<endl;
else
cout<<"Element found at "<<idx2<<" index and it's value is "<<_list[idx2].n<<endl;
}
輸出:
Element found at 1 index and it's value is 7
Element not found
返回索引將是一個很好的做法。 但是如果你堅持引用,我認為你可以在search_algo的末尾拋出異常。
返回容器的 last() 迭代器或len
以指示 find() 失敗。 這是 STL 的慣例,也是一個很好的做法。
template<typename InputIterator, typename T>
InputIterator find (InputIterator first, InputIterator last, const T& val)
{
while (first!=last) {
if (*first==val) return first;
++first;
}
return last;
}
如果您期望“未找到”作為有效結果,則不應返回對找到的對象的引用,因為 C++ 中沒有“空”引用。
您可以返回一個指針(nullptr 表示未找到),一個迭代器(最后一個表示未找到)。
在標准庫中,返回引用的函數通常不是用於搜索元素的,通常是沒有元素返回的例外情況。 所以它只會拋出一個異常,比如std::map::at()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.