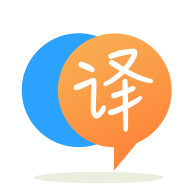
[英]Can I use istream_iterator<char> to copy some istream content into std::string?
[英]How could I use istream_iterator to convert unsigned char vector to a string?
我可以用普通的 for 循環來做到這一點:
vector<unsigned char> v{3, 13, 23, 83};
stringstream ss;
for (auto &el : v)
ss << std::hex << std::setw(2) << std::setfill('0') << static_cast<int>(el);
cout << ss.str() << endl;
我正在尋找允許我使用 std::copy 的方法:
std::copy(
std::begin(v),
std::end(v),
std::ostream_iterator<int>(ss << std::hex << std::setw(2) << std::setfill('0'))
);
這不會給我與 for 循環方法相同的輸出值。 對此我有兩個問題:
您使用 std::copy 的方法與
ss << std::hex << std::setw(2) << std::setfill('0');
std::copy(
std::begin(v),
std::end(v),
std::ostream_iterator<int>(ss)
);
由 std::setw 設置的寬度在第一次輸出操作后消失,因此只有第一個字符的輸出才會有它。
要使用 std::copy,您必須編寫自定義迭代器而不是使用 std::ostream_iterator。
您的第一種方法更好,因為它更短且更易於理解。
為了使用標准算法和輸出迭代器,我們可以定義一個新類並重載流插入運算符<<
。 std::copy
為我們想要流式傳輸的每個項目調用運算符<<
。
template <class T>
class HexOut
{
public:
HexOut(T val) : value(val)
{
}
friend std::ostream& operator<<(std::ostream& os, const HexOut<T>& elem)
{
os << std::hex << std::setw(2) << std::setfill('0') << static_cast<int>(elem.value) << std::dec;
return os;
}
private:
T value;
};
int main()
{
std::vector<unsigned char> v{ 3, 13, 23, 83 };
std::copy(v.begin(), v.end(), std::ostream_iterator<HexOut<unsigned char>>(std::cout));
return 0;
}
這里的操作符<<
每次都設置正確的輸出標志並將無符號字符轉換為 int。 如您所見,我們不得不編寫更多代碼並定義一個新類。 對於這樣的簡單任務來說,這可能是矯枉過正。 但是,使用迭代器使其可跨不同類型的流移植,並且我們利用了許多算法函數,例如std:copy
。 此外,所有格式都包含在運算符<<
,這使其更易於維護。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.