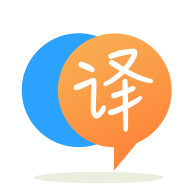
[英]How to translate a web page using selenium firefox and chrome driver in python?
[英]How to get status code in selenium chrome web driver in python
我在 selenium 中尋找 status_code 但找不到任何適合我需要的代碼。 我的另一個問題是,當我輸入一個不存在的域時,可以說https://gghgjeggeg.com 。 Selenium 不會引發任何錯誤。 它的頁面來源是這樣的:-
<html><head></head><body></body></html>
如何獲取狀態代碼(對於有效域,例如: https://twiitter.com/404errpage )以及為 Selenium 中不存在的域引發錯誤,或者是否有任何其他庫,例如 Selenium?
對於 Firefox 或 Chrome,您可以為此使用插件。 我們將狀態代碼保存在響應 cookie 中,並在 selenium 端讀取此 cookie。
您可以在此處閱讀有關瀏覽器擴展的更多信息:
鉻: https : //developer.chrome.com/extensions/getstarted
火狐: https : //developer.mozilla.org/en-US/docs/Web/Tutorials
注意:(未認證的插件僅適用於 Firefox Dev 版本,如果您想使用標准的 Firefox,您必須在 Firefox 站點上認證您的擴展。)
鉻版
//your_js_file_with_extension.js
var targetPage = "*://*/*";
function setStatusCodeDiv(e) {
chrome.cookies.set({
url: e.url,
name: 'status-code',
value: `${e.statusCode}`
});
}
chrome.webRequest.onCompleted.addListener(
setStatusCodeDiv,
{urls: [targetPage], types: ["main_frame"]}
);
顯現:
{
"description": "Save http status code in site cookies",
"manifest_version": 2,
"name": "StatusCodeInCookies",
"version": "1.0",
"permissions": [
"webRequest", "*://*/*", "cookies"
],
"background": {
"scripts": [ "your_js_file_with_extension.js" ]
}
}
Firefox 版本幾乎相同。
//your_js_file_with_extension.js
var targetPage = "*://*/*";
function setStatusCodeDiv(e) {
browser.cookies.set({
url: e.url,
name: 'status-code',
value: `${e.statusCode}`
});
}
browser.webRequest.onCompleted.addListener(
setStatusCodeDiv,
{urls: [targetPage], types: ["main_frame"]}
);
顯現:
{
"description": "Save http status code in site cookies",
"manifest_version": 2,
"name": "StatusCodeInCookies",
"version": "1.0",
"permissions": [
"webRequest", "*://*/*", "cookies"
],
"background": {
"scripts": [ "your_js_file_with_extension.js" ]
},
"applications": {
"gecko": {
"id": "some_id"
}
}
}
接下來你必須構建這個擴展:
對於 Chrome,您必須創建 *.pem 和 *.crx 文件(powershell 腳本):
start-Process -FilePath "C:\Program Files (x86)\Google\Chrome\Application\chrome.exe" -ArgumentList "--pack-extension=C:\Path\to\your\js\and\manifest"
Firefox(我們只需要 zip 文件):
[io.compression.zipfile]::CreateFromDirectory('C:\Path\to\your\js\and\manifest', 'destination\folder')
硒步驟
好的,當我們有擴展時,我們可以將它添加到我們的 selenium 應用程序中。 我用 C# 編寫我們的版本,但我認為很容易將其重寫為其他語言(在這里你可以找到 Python 版本: Using Extensions with Selenium (Python) )。
使用 Chrome 驅動器加載擴展程序:
var options = new ChromeOptions();
options.AddExtension(Path.Combine(System.Environment.CurrentDirectory,@"Selenium\BrowsersExtensions\Compiled\YOUR_CHROME_EXTENSION.crx"));
var chromeDriver = new ChromeDriver(ChromeDriverService.CreateDefaultService(), options);
使用 Firefox 加載(您必須使用配置文件):
var profile = new FirefoxProfile();
profile.AddExtension(Path.Combine(System.Environment.CurrentDirectory,@"Selenium\BrowsersExtensions\Compiled\YOUR_FIREFOX_EXTENSION.zip"));
var options = new FirefoxOptions
{
Profile = profile
};
var firefoxDriver = new FirefoxDriver(FirefoxDriverService.CreateDefaultService(), options);
好的,我們差不多完成了,現在我們需要從 cookie 中讀取狀態代碼,這應該類似於:
webDriver.Navigate().GoToUrl('your_url');
if (webDriver.Manage() is IOptions options
&& options.Cookies.GetCookieNamed("status-code") is Cookie cookie
&& int.TryParse(cookie.Value, out var statusCode))
{
//we delete cookies after we read status code but this is not necessary
options.Cookies.DeleteCookieNamed("status-code");
return statusCode;
}
logger.Warn($"Can't get http status code from {webDriver.Url}");
return 500;
這就是全部。 我在任何地方都沒有看到這樣的答案。 希望我有所幫助。
Selenium 不打算用於直接檢查 HTTP 狀態代碼。 Selenium 用於像用戶一樣與網站交互。 而一般用戶不會打開開發者工具查看HTTP狀態碼,而是查看頁面內容。
我什至看到頁面響應 HTTP 200 OK 向用戶傳遞“找不到資源”消息。
甚至 Selenium 開發人員也解決了這個問題:
瀏覽器將始終表示 HTTP 狀態代碼,例如 404 或 500 錯誤頁面。 當您遇到這些錯誤頁面之一時,“快速失敗”的一種簡單方法是在每個頁面加載后檢查頁面標題或可靠點(例如
<h1>
標簽)的內容。
來源: selenium.dev / 最糟糕的做法 / HTTP 響應代碼
如果您堅持使用 Selenium,您最好找到第一個h1
元素並尋找典型的 Chrome 404 簽名:
h1 = driver.find_element_by_css_selector('h1')
if h1.text == u"This site can’t be reached":
print("Not found")
雖然,如果你想抓取網站,你甚至可以使用 urllib,就像評論中建議的 Tek Nath:
import urllib.request
import urllib.request
import urllib.error
try:
with urllib.request.urlopen('http://www.safasdfsadfsadfdsf.org/') as f:
print(f.read())
print(f.status)
print(f.getheader("content-length"))
except urllib.error.URLError as e:
print(e.reason)
由於域不存在,代碼將運行到異常處理程序分支。
有關詳細信息和更多示例,請參閱 Python 文檔:
然后,您可能希望使用 DOM 解析器將 HTML 標記處理為 DOM 樹,以便於處理。 雖然這超出了這個問題 - 從這里開始:
xml.dom
(Python 文檔)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.