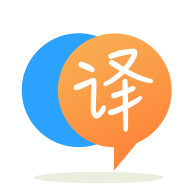
[英]How to call parameterized constructor of member object variable in a class' default constructor in C++?
[英]How to make object of a class with parameterized constructor in other class?
我想在Area
類中創建一個DataArea
類的對象並在main
函數中初始化數據。 但是我的代碼工作的唯一方法是在Area
類中初始化數據。
另外,我不知道我是否正確地制作了對象。 請指導我。 我的代碼如下:
#include<iostream>
using namespace std;
class DataArea
{
public:
int radius, length, width, base, heigth;
DataArea(int l, int w, int b, int h, int r)
{
length = l;
width = w;
radius = r;
heigth = h;
base = b;
}
};
class Area
{
public:
DataArea* s = new DataArea(3, 4, 5, 6, 7);
float AreaCirle()
{
return 3.142 * s->radius * s->radius;
}
float AreaRectangle()
{
return s->length * s->width;
}
float AreaTraingle()
{
return (s->base * s->heigth) / 2;
}
};
class print_data : public Area
{
public:
void print()
{
cout << "Area of Circle is: " << AreaCirle() << endl;
cout << "Area of Rectangle is: " << AreaRectangle() << endl;
cout << "Area of Traingle is: " << AreaTraingle() << endl;
}
};
int main()
{
//DataArea da(3, 4, 5, 6, 7);
print_data m;
m.print();
}
在我看來, Area
課程對於您想要實現的目標來說是多余的。 您可能應該將方法直接放在DataArea
類中。 然后,您可以根據需要創建任意數量的DataArea
對象...
像這樣:
class DataArea
{
public:
int radius, length, width, base, heigth;
DataArea(int l , int w , int b , int h , int r )
{
length = l;
width = w;
radius = r;
heigth = h;
base = b;
}
float AreaCirle()
{
return 3.142 * radius * radius;
}
float AreaRectangle()
{
return length * width ;
}
float AreaTraingle()
{
return (base * heigth)/2;
}
};
int main(int argc, char **argv)
{
DataArea area1 (1,2,3,4,5);
DataArea area2 (8,2,3,4,5);
std::cout << area1.AreaCirle() << std::endl;
std::cout << area2.AreaCirle() << std::endl;
}
您可能難以理解這個概念的原因是:您正在定義一個類並實例化一個對象。 有時這些術語可以互換使用,但在這種情況下,這是一個重要的區別。
如果您希望您的方法對某個其他類進行操作,那么您應該創建接受該類作為參數的方法。 否則,它是不必要的復雜。
如果您不在Area
類之外使用它,您的DataArea
基本上是絕對的。 同樣, print_data
類可以替換為operator<<
重載。
以下是更新后的代碼,其中的注釋將引導您完成。
#include <iostream>
// DataArea (optionally) can be the part of Area class
struct DataArea /* final */
{
float length, width, base, height, radius;
DataArea(float l, float w, float b, float h, float r)
: length{ l } // use member initializer lists to initlize the members
, width{ w }
, base{ b }
, height{ h }
, radius{ r }
{}
};
class Area /* final */
{
DataArea mDataArea; // DataArea as member
public:
// provide a constructor which initialize the `DataArea` member
Area(float l, float w, float b, float h, float r)
: mDataArea{ l, w, b, h, r } // member initializer
{}
// camelCase naming for the functions and variables
// mark it as const as the function does not change the member
float areaCirle() const /* noexcept */
{
return 3.142f * mDataArea.radius * mDataArea.radius;
}
float areaRectangle() const /* noexcept */
{
return mDataArea.length * mDataArea.width;
}
float areaTraingle() const /* noexcept */
{
return (mDataArea.base * mDataArea.height) / 2.f;
}
// provide a operator<< for printing the results
friend std::ostream& operator<<(std::ostream& out, const Area& areaObject) /* noexcept */;
};
std::ostream& operator<<(std::ostream& out, const Area& areaObject) /* noexcept */
{
out << "Area of Circle is: " << areaObject.areaCirle() << "\n";
out << "Area of Rectangle is: " << areaObject.areaRectangle() << "\n";
out << "Area of Traingle is: " << areaObject.areaTraingle() << "\n";
return out;
}
int main()
{
// now construct the Area object like this
Area obj{ 3, 4, 5, 6, 7 };
// simply print the result which uses the operator<< overload of the Area class
std::cout << obj;
}
輸出:
Area of Circle is: 153.958
Area of Rectangle is: 12
Area of Traingle is: 15
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.