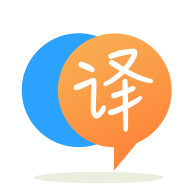
[英]How to quick-sort a 1D array based on 3rd column (index divided by 3) in C++
[英]How do I sort string vectors based on 2nd column/3rd column etc?
我有一個
vector<string>data
如此組織
//NAME ID AGE
//NAME ID AGE
//NAME ID AGE
//NAME ID AGE
我可以按字母順序按名稱排序,如何根據第 2 列/第 3 列按升序對其進行排序? 感謝您的任何幫助和建議。
std::sort
的第三個重載有第三個參數允許您提供一個函數來執行排序邏輯。
// get nth token from a string
std::string getnTh(const std::string & str, int n)
{
std::istringstream strm(str);
std::string result;
for (int count = 0; count < n; count++)
{
if (!(strm >> result))
{
throw std::out_of_range("ran out of tokens before n");
}
}
return result;
}
// get ID, second token, from string
std::string get_ID(const std::string str)
{
return getnTh(str, 2);
}
// compare the ID field, second token, in two strings
bool cmp_ID(const std::string &a, const std::string &b)
{
std::string tokena = get_ID(a);
std::string tokenb = get_ID(b);
return tokena < tokenb;
}
int main()
{
std::vector<std::string> data {"c c c ", "b b b " , "a a a"};
std::sort (data.begin(), data.end(), cmp_ID);
}
注意:此代碼可以稍微壓縮一下。 為了便於閱讀,我已將其逐步分解。
注意:這是殘酷的! 它不斷地一遍遍解析同一個string
,令人作嘔的白費力氣。
相反,您應該創建一個結構來存儲已經解析的字符串並將該結構存儲在std::vector
。
// stores a person
struct person
{
std::string name;
std::string ID;
std::string age;
// constructor to parse an input string into a new person
person(const std::string & in)
{
std::istringstream strm(in);
if (!(strm >> name >> ID >> age))
{
throw std::runtime_error("invalid person input");
}
}
};
// much simpler and faster compare function. All of the parsing is done once ahead of time.
bool cmp_ID(const person &a, const person &b)
{
return a.ID < b.ID;
}
int main()
{
// replaces vector<string> data
std::vector<person> data {{"c c c"}, {"b b b"} , {"a a a"}};
std::sort (data.begin(), data.end(), cmp_ID);
}
您可以按每個字符讀取這些字符串,直到遇到第一個/第二個空格。 然后你應該能夠“過濾”出第一個/第二個屬性。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.