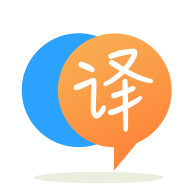
[英]Why doesn't scanf need an ampersand for strings and also works fine in printf (in C)?
[英]Why does an integer need an ampersand when formatting a pointer in printf while a character array doesn't in C?
具體來說,當我想格式化所述整數的指針時。 我正在研究 The Art of Exploitation,它在以下代碼片段中讓我印象深刻。
#include <stdio.h>
#include <string.h>
int main(int argc, char *argv[]) {
int value = 5;
char buffer_one[8], buffer_two[8];
strcpy(buffer_one, "one"); /* Put "one" into buffer_one. */
strcpy(buffer_two, "two"); /* Put "two" into buffer_two. */
printf("[BEFORE] buffer_two is at %p and contains \'%s\'\n", buffer_two, buffer_two);
printf("[BEFORE] buffer_one is at %p and contains \'%s\'\n", buffer_one, buffer_one);
printf("[BEFORE] value is at %p and is %d (0x%08x)\n", &value, value, value);
printf("\n[STRCPY] copying %d bytes into buffer_two\n\n", strlen(argv[1]));
strcpy(buffer_two, argv[1]); /* Copy first argument into buffer_two. */
printf("[AFTER] buffer_two is at %p and contains \'%s\'\n", buffer_two, buffer_two);
printf("[AFTER] buffer_one is at %p and contains \'%s\'\n", buffer_one, buffer_one);
printf("[AFTER] value is at %p and is %d (0x%08x)\n", &value, value, value);
}
盡管buffer_one
是char[8]
類型,但在某些情況下,例如作為函數參數傳遞時,它會衰減為設置為數組第一個元素的指針。 該指針將是char*
類型。
由於期望strcpy
的第一個參數是char*
類型,編譯成功。
&
將產生指向該值的指針(引用它)。 在該上下文中,字符數組已被視為指針。
我敢於嘗試比較buffer_one
和&buffer_one
值。 它們是不同的類型,編譯器應該警告你,但是實際值應該是相同的。
char buffer_one[8];
assert(buffer_one == &buffer_one); // should pass
請注意,這與也可以用作字符串變量的字符指針不同(從實際角度來看)。 引用一個指針會產生一個指向該指針的地址:
char *test = "lorem";
assert(test != &test); // should pass
為什么在 printf 中格式化指針時整數需要一個&符號而字符數組不需要?
要打印像int
或char []
這樣的對象的指針,代碼使用&
來獲取對象的地址。
int value = 5;
char buffer_one[8];
printf("Address of an int: %p\n", (void *) &value);
printf("Address of an array: %p\n", (void *) &buffer_one);
如果代碼不使用&
,則對象必須是或轉換為指針。 下面將數組buffer_one
轉換為其第一個元素的地址。
printf("Address of initial array element: %p\n", (void *) buffer_one);
結論&value
是必需的,因為int
不會自動轉換為指針。 buffer_one
不需要&
因為它在此用法中轉換為指針。 buffer_one
也可以使用&
,就像任何對象一樣。
數組buffer_one
的地址與其第一個元素的地址相同。 雖然它很常見,但上面的最后 2 個打印將打印相同的指針,C 不需要這個。 數組的地址和char
的地址是等價的,但不一定編碼相同。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.