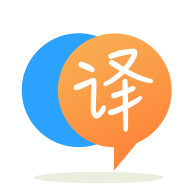
[英]how to display one row from SQLite database to textview/edittext
[英]How to get data from SQLite database and display it in EditText
如何從 SQLite 數據庫中獲取數據並在 EditText 中顯示
我需要幫助從 SQLite 獲取數據並在 EditText 中顯示它我為 getdata 編碼是正確的,如何在 EditText 中顯示部分請幫助我。 我需要確切的代碼來將數據從數據庫顯示到 EditText 中
提前致謝
```
SQLite databse code
public class DatabaseStudent extends SQLiteOpenHelper {
public static final String databasename="Student";
public static final String Student_table="Student_table";
public static final String Student_name="Name";
public static final String Student_rollnumber="RollNumber";
public static final String Student_College="College";
public static final String Student_Branch="Branch";
public static final String Student_Year="Year";
public static final String Parent_name="ParentName";
public static final String Parent_address="ParentAddress";
public static final String Parent_Phone_number="Phone";
public static final String Student_Email_ID="Email";
public static final String Student_Password="Password";
public static final int versioncode=1;
public DatabaseStudent(Context context){
super(
context,
databasename,
null,
versioncode);
}
@Override
public void onCreate(SQLiteDatabase database) {
String student_query;
student_query="CREATE TABLE IF NOT EXISTS "+Student_table+"(Name TEXT,RollNumber NUMBER PRIMARY KEY,College TEXT,Branch TEXT,Year TEXT,ParentName TEXT,ParentAddress TEXT,Phone NUMBER, Email Text, Password TEXT)";
database.execSQL(student_query);
}
@Override
public void onUpgrade(SQLiteDatabase database, int oldVersion, int newVersion) {
String student_query;
student_query= "DROP TABLE IF EXISTS " + Student_table;
database.execSQL(student_query);
}
public boolean Student_Data(String name,String rollnumber,String college,String branch,String year,String parentname,String parentaddress,String phone,String email,String password){
SQLiteDatabase db1=this.getWritableDatabase();
ContentValues cv=new ContentValues();
cv.put(Student_name,name);
cv.put(Student_rollnumber,rollnumber);
cv.put(Student_College,college);
cv.put(Student_Branch,branch);
cv.put(Student_Year,year);
cv.put(Parent_name,parentname);
cv.put(Parent_address,parentaddress);
cv.put(Parent_Phone_number,phone);
cv.put(Student_Email_ID,email);
cv.put(Student_Password,password);
long result=db1.insert(Student_table,null,cv);
if(result==-1)
return false;
else
return true;
}
public Cursor StudentData()
{
SQLiteDatabase db1=this.getWritableDatabase();
Cursor res = db1.rawQuery("select * from "+Student_table,null);
return res;
}
```
```
**java code**
public class StudentProfileActivity extends AppCompatActivity {
EditText editname,editroll,editcollege,editbranch,edityear,editparentname,editparentaddress,editphone,editemail,editpassword;
SQLiteDatabase sqLiteDatabase;
DatabaseStudent dbh;
Cursor res;
Student_Listadapter la;
Button btnupadte;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_student_profile);
editname=findViewById(R.id.editname);
editroll=findViewById(R.id.editrollnumber);
editcollege=findViewById(R.id.editcollege);
editbranch=findViewById(R.id.editbranch);
edityear=findViewById(R.id.edityear);
editparentaddress=findViewById(R.id.editparentaddress);
editparentname=findViewById(R.id.editparentname);
editphone=findViewById(R.id.editphone);
editemail=findViewById(R.id.editemail);
editpassword=findViewById(R.id.editpassword);
btnupadte=findViewById(R.id.btnupdate);
dbh = new DatabaseStudent(getApplicationContext());
sqLiteDatabase=dbh.getReadableDatabase();
res=dbh.StudentData();
if(res.moveToFirst())
{
do{
String name,rollnumber,college,branch,year,parentname,parentaddress,phone,email,password;
name= res.getString(0);
rollnumber=res.getString(1);
college=res.getString(2);
branch=res.getString(3);
year=res.getString(4);
parentname=res.getString(5);
parentaddress=res.getString(6);
phone=res.getString(7);
email=res.getString(8);
password=res.getString(9);
Dataprovider_Student DPC= new Dataprovider_Student(name,rollnumber,college,branch,year,parentname,parentaddress,phone,email,password);
la.add(DPC);
}while(res.moveToNext());
}
btnupadte.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
}
});
```
```
**xml code**
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:gravity="center"
android:background="@drawable/studentlist"
android:padding="10dp"
tools:context=".StudentProfileActivity">
<EditText
android:id="@+id/editname"
android:hint="Name"
android:ems="10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<EditText
android:id="@+id/editrollnumber"
android:hint="Roll number"
android:ems="10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<EditText
android:id="@+id/editcollege"
android:hint="college"
android:ems="10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<EditText
android:id="@+id/editbranch"
android:hint="branch"
android:ems="10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<EditText
android:id="@+id/edityear"
android:hint="year"
android:ems="10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<EditText
android:id="@+id/editparentname"
android:hint="parentname"
android:ems="10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<EditText
android:id="@+id/editparentaddress"
android:hint="parentaddress"
android:ems="10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<EditText
android:id="@+id/editphone"
android:hint="phone"
android:ems="10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<EditText
android:id="@+id/editemail"
android:hint="email"
android:ems="10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<EditText
android:id="@+id/editpassword"
android:hint="password"
android:ems="10"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btnupdate"
android:text="UPDATE"
android:textColor="#fff"
android:background="#009688"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
```
設置檢索到的文本(來自 SQLite DB)以在布局中編輯文本視圖,類似於下面的代碼
在 StudentProfileActivity.java 中,在 do-while 循環中,在“la.add(DPC);”行之后
editname.setText(name);
editroll.setText(rollnumber);
editcollege.setText(college);
.
.
.
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.