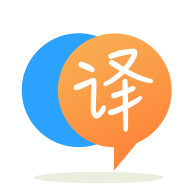
[英]Why is React functional component state not retaining state value?
[英]Why callbacks in react functional component is not reading the updated state value
我試圖在反應中使用交叉點觀察器實現無限滾動,但我面臨的問題是在交叉點觀察器的回調中,我無法讀取當前“頁面”和“列表”的最新值,以便我可以獲取數據下一頁。
import ReactDOM from "react-dom";
import "./styles.css";
require("intersection-observer");
const pageSize = 30;
const threshold = 5;
const generateList = (page, size) => {
let arr = [];
for (let i = 1; i <= size; i++) {
arr.push(`${(page - 1) * size + i}`);
}
return arr;
};
const fetchList = page => {
return new Promise(resolve => {
setTimeout(() => {
return resolve(generateList(page, pageSize));
}, 1000);
});
};
let options = {
root: null,
threshold: 0
};
function App() {
const [page, setPage] = useState(1);
const [fetching, setFetching] = useState(false);
const [list, setlist] = useState(generateList(page, pageSize));
const callback = entries => {
if (entries[0].isIntersecting) {
observerRef.current.unobserve(
document.getElementById(`item_${list.length - threshold}`)
);
setFetching(true);
/* at this point neither the 'page' is latest nor the 'list'
*they both have the initial states.
*/
fetchList(page + 1).then(res => {
setFetching(false);
setPage(page + 1);
setlist([...list, ...res]);
});
}
};
const observerRef = useRef(new IntersectionObserver(callback, options));
useEffect(() => {
if (observerRef.current) {
observerRef.current.observe(
document.getElementById(`item_${list.length - threshold}`)
);
}
}, [list]);
return (
<div className="App">
{list.map(l => (
<p key={l} id={`item_${l}`}>
{l}
</p>
))}
{fetching && <p>loading...</p>}
</div>
);
}
const rootElement = document.getElementById("root");
ReactDOM.render(<App />, rootElement);
當前行為: “頁面”和“列表”的值始終等於初始狀態而不是最新值。 第 2 頁后無限滾動不起作用
預期行為:在回調函數中,它應該讀取狀態“頁面”和“列表”的更新值。
這是這個演示的工作沙箱https://codesandbox.io/s/sweet-sun-rbcml?fontsize=14&hidenavigation=1&theme=dark
這里主要有兩個問題,閉包和直接查詢 DOM。
要解決閉包問題,請使用函數式useState
和引用:
const listLengthRef = useRef(list.length);
const pageRef = useRef(page);
const callback = useCallback(entries => {
if (entries[0].isIntersecting) {
observerRef.current.unobserve(
document.getElementById(`item_${listLengthRef.current - threshold}`)
);
setFetching(true);
fetchList(pageRef.current + 1).then(res => {
setFetching(false);
setPage(page => page + 1);
setlist(list => [...list, ...res]);
});
}
}, []);
const observerRef = useRef(new IntersectionObserver(callback, options));
useEffect(() => {
listLengthRef.current = list.length;
}, [list]);
useEffect(() => {
pageRef.current = page;
}, [page]);
盡管此代碼有效,但您應該將document.getElementById
替換為引用,在這種情況下,它將是對頁面最后一個元素的引用。
您可以使用 React setState
回調方法來保證您將收到以前的值。
如下更新您的callback
函數,它應該可以工作。
const callback = entries => {
if (entries[0].isIntersecting) {
setFetching(true);
setPage(prevPage => {
fetchList(prevPage + 1).then(res => {
setFetching(false);
setlist(prevList => {
observerRef.current.unobserve(document.getElementById(`item_${prevList.length - threshold}`));
return ([...prevList, ...res]);
});
})
return prevPage + 1;
})
}
};
我認為問題是由於 ref 一直引用舊觀察者。 每次更新依賴項時都需要刷新觀察者。 它與js中的閉包有關。 我會更新您的應用程序以將回調移動到 useEffect 中
function App() {
const [page, setPage] = useState(1);
const [fetching, setFetching] = useState(false);
const [list, setlist] = useState(generateList(page, pageSize));
const observerRef = useRef(null);
useEffect(() => {
const callback = entries => {
if (entries[0].isIntersecting) {
observerRef.current.unobserve(
document.getElementById(`item_${list.length - threshold}`)
);
setFetching(true);
/* at this point neither the 'page' is latest nor the 'list'
*they both have the initial states.
*/
console.log(page, list);
fetchList(page + 1).then(res => {
setFetching(false);
setPage(page + 1);
setlist([...list, ...res]);
});
}
};
observerRef.current = new IntersectionObserver(callback, options);
if (observerRef.current) {
observerRef.current.observe(
document.getElementById(`item_${list.length - threshold}`)
);
}
}, [list]);
return (
<div className="App">
{list.map(l => (
<p key={l} id={`item_${l}`}>
{l}
</p>
))}
{fetching && <p>loading...</p>}
</div>
);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.