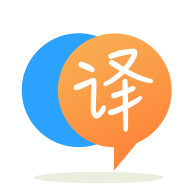
[英]Spring boot hibernate bidirectional mapping many to one can not established relationship
[英]Spring boot Hibernate one to Many Relationship
我有兩個實體產品和產品選項。 產品可以有多個產品選項,可以看出我已經將它與 oneToMany 關系進行了映射。
@Entity
public class Product {
@Id
@GeneratedValue(strategy=GenerationType.IDENTITY)
private int id;
private String description;
private String productCategory;
private String optionDescription;
private BigDecimal productBasePrice;
@OneToMany(mappedBy = "product",cascade = CascadeType.ALL)
private Set<ProductOption>productOptions=new HashSet<>();
public Product() {}
public Product(String description, ProductCategory productCategory,String optionDescription, BigDecimal productBasePrice) {
super();
this.description = description;
this.productCategory=productCategory.toString();
this.optionDescription = optionDescription;
this.productBasePrice = productBasePrice;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getDescription() {
return description;
}
public String getProductCategory() {
return productCategory;
}
public void setProductCategory(String productCategory) {
this.productCategory = productCategory;
}
public void setDescription(String description) {
this.description = description;
}
public String getOptionDescription() {
return optionDescription;
}
public void setOptionDescription(String optionDescription) {
this.optionDescription = optionDescription;
}
public BigDecimal getProductBasePrice() {
return productBasePrice;
}
public void setProductBasePrice(BigDecimal productBasePrice) {
this.productBasePrice = productBasePrice;
}
public Set<ProductOption> getProductOptions() {
return productOptions;
}
public void setProductOptions(Set<ProductOption> productOptions) {
this.productOptions = productOptions;
}
}
和產品選項
@Entity
public class ProductOption {
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
private Long id;
private String productOptionDescription;
private BigDecimal optionPrice;
private BigDecimal optionPriceForSmall;
private BigDecimal optionPriceForNormal;
private BigDecimal optionPriceForFamily;
private BigDecimal optionPriceForParty;
@ManyToOne
@JoinColumn
private Product product;
public ProductOption() {}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getProductOptionDescription() {
return productOptionDescription;
}
public void setProductOptionDescription(String productOptionDescription) {
this.productOptionDescription = productOptionDescription;
}
public BigDecimal getOptionPrice() {
return optionPrice;
}
public void setOptionPrice(BigDecimal optionPrice) {
this.optionPrice = optionPrice;
}
public BigDecimal getOptionPriceForSmall() {
return optionPriceForSmall;
}
public void setOptionPriceForSmall(BigDecimal optionPriceForSmall) {
this.optionPriceForSmall = optionPriceForSmall;
}
public BigDecimal getOptionPriceForNormal() {
return optionPriceForNormal;
}
public void setOptionPriceForNormal(BigDecimal optionPriceForNormal) {
this.optionPriceForNormal = optionPriceForNormal;
}
public BigDecimal getOptionPriceForFamily() {
return optionPriceForFamily;
}
public void setOptionPriceForFamily(BigDecimal optionPriceForFamily) {
this.optionPriceForFamily = optionPriceForFamily;
}
public BigDecimal getOptionPriceForParty() {
return optionPriceForParty;
}
public void setOptionPriceForParty(BigDecimal optionPriceForParty) {
this.optionPriceForParty = optionPriceForParty;
}
public ProductOption(String productOptionDescription, BigDecimal optionPrice, BigDecimal optionPriceForSmall,
BigDecimal optionPriceForNormal, BigDecimal optionPriceForFamily, BigDecimal optionPriceForParty) {
super();
this.productOptionDescription = productOptionDescription;
this.optionPrice = optionPrice;
this.optionPriceForSmall = optionPriceForSmall;
this.optionPriceForNormal = optionPriceForNormal;
this.optionPriceForFamily = optionPriceForFamily;
this.optionPriceForParty = optionPriceForParty;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((id == null) ? 0 : id.hashCode());
result = prime * result + ((optionPrice == null) ? 0 : optionPrice.hashCode());
result = prime * result + ((optionPriceForFamily == null) ? 0 : optionPriceForFamily.hashCode());
result = prime * result + ((optionPriceForNormal == null) ? 0 : optionPriceForNormal.hashCode());
result = prime * result + ((optionPriceForParty == null) ? 0 : optionPriceForParty.hashCode());
result = prime * result + ((optionPriceForSmall == null) ? 0 : optionPriceForSmall.hashCode());
result = prime * result + ((productOptionDescription == null) ? 0 : productOptionDescription.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
ProductOption other = (ProductOption) obj;
if (id == null) {
if (other.id != null)
return false;
} else if (!id.equals(other.id))
return false;
if (optionPrice == null) {
if (other.optionPrice != null)
return false;
} else if (!optionPrice.equals(other.optionPrice))
return false;
if (optionPriceForFamily == null) {
if (other.optionPriceForFamily != null)
return false;
} else if (!optionPriceForFamily.equals(other.optionPriceForFamily))
return false;
if (optionPriceForNormal == null) {
if (other.optionPriceForNormal != null)
return false;
} else if (!optionPriceForNormal.equals(other.optionPriceForNormal))
return false;
if (optionPriceForParty == null) {
if (other.optionPriceForParty != null)
return false;
} else if (!optionPriceForParty.equals(other.optionPriceForParty))
return false;
if (optionPriceForSmall == null) {
if (other.optionPriceForSmall != null)
return false;
} else if (!optionPriceForSmall.equals(other.optionPriceForSmall))
return false;
if (productOptionDescription == null) {
if (other.productOptionDescription != null)
return false;
} else if (!productOptionDescription.equals(other.productOptionDescription))
return false;
return true;
}
@Override
public String toString() {
return "ProductOption [id=" + id + ", productOptionDescription=" + productOptionDescription + ", optionPrice="
+ optionPrice + ", optionPriceForSmall=" + optionPriceForSmall + ", optionPriceForNormal="
+ optionPriceForNormal + ", optionPriceForFamily=" + optionPriceForFamily + ", optionPriceForParty="
+ optionPriceForParty + "]";
}
}
這就是我初始化數據的方式。
@Component
public class WebsiteBootstrap implements ApplicationListener<ContextRefreshedEvent>{
private ProductRepository productRepository;
@Override
public void onApplicationEvent(ContextRefreshedEvent arg0) {
initProducts();
}
public WebsiteBootstrap( ProductRepository productRepository,
ProductOptionRepository productOptionRepository) {
this.productRepository=productRepository;
}
private void initProducts() {
ProductOption productOpton1=new ProductOption("mit Cocktailsauce", new BigDecimal(0), null, null, null, null);
ProductOption productOpton2=new ProductOption("mit Joghurtsauce", new BigDecimal(0), null, null, null, null);
ProductOption productOpton3=new ProductOption("ohne Sauce", new BigDecimal(0), null, null, null, null);
Product product37= new Product("Falafel", ProductCategory.Vegatarische_Döner, "Wahl aus: mit Cocktailsauce, mit Joghurtsauce oder ohne Sauce.", new BigDecimal(5.00));
product37.getProductOptions().add(productOpton1);
product37.getProductOptions().add(productOpton2);
product37.getProductOptions().add(productOpton3);
this.productRepository.save(product37);
}
在數據庫中我找不到product_id
。
我的映射中缺少什么?
ProductOptions
在您的情況下是子實體(它包含帶有外鍵的列),因此它負責保存關系。 您必須在子端保存實體:
private void initProducts() {
ProductOption productOpton1=new ProductOption("mit Cocktailsauce", new BigDecimal(0), null, null, null, null);
ProductOption productOpton2=new ProductOption("mit Joghurtsauce", new BigDecimal(0), null, null, null, null);
ProductOption productOpton3=new ProductOption("ohne Sauce", new BigDecimal(0), null, null, null, null);
Product product37= new Product("Falafel", ProductCategory.Vegatarische_Döner, "Wahl aus: mit Cocktailsauce, mit Joghurtsauce oder ohne Sauce.", new BigDecimal(5.00));
product37.getProductOptions().add(productOpton1);
product37.getProductOptions().add(productOpton2);
product37.getProductOptions().add(productOpton3);
productOpton1.setProduct(product37);
productOpton2.setProduct(product37);
productOpton3.setProduct(product37);
this.productRepository.save(product37);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.