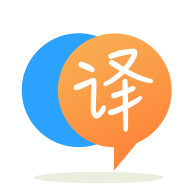
[英]How to save generated PDF files to MySQL db using spring boot?
[英]How to send generated PDF document to frontend in Restfull way in Spring Boot?
我已經通過指南 pdf 生成實現了。 我的類PdfView
有一個方法:
@Override
protected void buildPdfDocument(Map<String, Object> model, Document document, PdfWriter writer, HttpServletRequest request, HttpServletResponse response) {
response.setHeader("Content-Disposition", "attachment; filename=\"animal-profile.pdf\"");
List<Animal> animals = (List<Animal>) model.get("animals");
try {
document.add(new Paragraph("Generated animals: " + LocalDate.now()));
} catch (DocumentException e) {
e.printStackTrace();
}
PdfPTable table = new PdfPTable(animals.stream().findAny().get().getColumnCount());
table.setWidthPercentage(100.0f);
table.setSpacingBefore(10);
Font font = FontFactory.getFont(FontFactory.TIMES);
font.setColor(BaseColor.WHITE);
PdfPCell cell = new PdfPCell();
cell.setBackgroundColor(BaseColor.DARK_GRAY);
cell.setPadding(5);
cell.setPhrase(new Phrase("Animal Id", font));
table.addCell(cell);
cell.setPhrase(new Phrase("Animal name", font));
for (Animal animal : animals) {
table.addCell(animal.getId().toString());
table.addCell(animal.getName());
}
document.add(table);
}
我應該如何使用 GET 方法實現控制器來下載 PDF?
示例代碼如下所示,使用 API 下載圖像 -
@RequestMapping("/api/download/{fileName:.+}")
public void downloadPDFResource(HttpServletRequest request, HttpServletResponse response,@PathVariable("fileName") String fileName) throws IOException {
String path = somePath + fileName;
File file = new File(path);
if (file.exists()) {
String mimeType = URLConnection.guessContentTypeFromName(file.getName()); // for you it would be application/pdf
if (mimeType == null) mimeType = "application/octet-stream";
response.setContentType(mimeType);
response.setHeader("Content-Disposition", String.format("inline; filename=\"" + file.getName() + "\""));
response.setContentLength((int) file.length());
InputStream inputStream = new BufferedInputStream(new FileInputStream(file));
FileCopyUtils.copy(inputStream, response.getOutputStream());
}
}
轉到 url - http://localhost:8080/download/api/download/fileName.pdf假設您的服務部署在端口 8080
您應該能夠預覽文件。
注意:如果要下載文件,請將 Content-Disposition 設置為附件
AbstractPdfView
本身就是一個View
它的實例可以簡單地從處理程序方法返回以呈現文檔。
來自Spring 參考。 PDF 視圖:
控制器可以從外部視圖定義(通過名稱引用它)或作為處理程序方法的
View
實例返回這樣的視圖。
這是一個最小的例子:
SimplePdfView.kt
:
class SimplePdfView : AbstractPdfView() {
override fun buildPdfDocument(
model: MutableMap<String, Any>,
document: Document, writer: PdfWriter,
request: HttpServletRequest, response: HttpServletResponse
) {
document.add(Paragraph("My paragraph"))
}
}
SimpleController.kt
:
@Controller
class SimpleController {
@GetMapping
fun getPdf() = SimplePdfView()
}
當/
端點被擊中時,pdf 將在瀏覽器中呈現。
如果你需要下載已經存在的pdf,你可以看看這個答案
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.