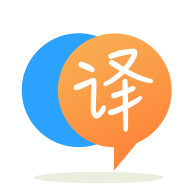
[英]Reading gzipped string in sqlite3 database (python write, Java Android read)
[英]How do I convert an image to a String to read/write it to a SQLite Database - Android Studio (Java)
我無法使用 Java 將圖像轉換為字符串以將其讀/寫到 Android Studio 上的 SQLite 數據庫。 我看了很多教程,似乎找不到我遺漏的東西。 任何幫助將不勝感激!
我的錯誤源於沒有從主活動中的 AddData 轉換它:
數據庫助手:
public class DatabaseHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "relove.db";
public static final String TABLE_NAME = "PLGarments_Table";
public static final String COL_ID = "ID";
public static final String COL_TITLE = "TITLE";
public static final String COL_DESCRIPTION = "DESCRIPTION";
public static final String COL_OWNER = "OWNER";
public static final String COL_IMAGE = "image";
public DatabaseHelper(@Nullable Context context) {
super(context, DATABASE_NAME , null, 1);
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL("create table " + TABLE_NAME + "(ID INTEGER PRIMARY KEY AUTOINCREMENT, TITLE TEXT, DESCRIPTION TEXT, OWNER TEXT, IMAGE BLOB)");
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL(" Drop table if exists " + TABLE_NAME );
onCreate(db);
}
public void queryData(String sql){
SQLiteDatabase database = getWritableDatabase();
database.execSQL(sql);
} //unnecessary?
public boolean insertData(String TITLE, String DESCRIPTION, String OWNER, byte[] image){ //CREATE
SQLiteDatabase db = this.getWritableDatabase();
ContentValues contentValues = new ContentValues();
contentValues.put(COL_TITLE,TITLE);
contentValues.put(COL_DESCRIPTION,DESCRIPTION);
contentValues.put(COL_OWNER,OWNER);
contentValues.put(COL_IMAGE, image);
long result = db.insert(TABLE_NAME, null,contentValues );
if (result == -1)
return false;
else
return true;
}
public Cursor getAllData(){ //RETRIEVE
SQLiteDatabase db = this.getWritableDatabase();
Cursor res = db.rawQuery("select * from " + TABLE_NAME, null);
return res;
}
public boolean updateData(String ID,String TITLE, String DESCRIPTION, String OWNER, byte[] image ){ //UPDATE
SQLiteDatabase db = this.getWritableDatabase(); //instance of database
ContentValues contentValues = new ContentValues();
contentValues.put(COL_ID, ID);
contentValues.put(COL_TITLE,TITLE);
contentValues.put(COL_DESCRIPTION,DESCRIPTION);
contentValues.put(COL_OWNER,OWNER);
contentValues.put(COL_IMAGE, image);
db.update(TABLE_NAME, contentValues, "ID = ?", new String[] {ID});
return true;
}
public Integer deleteData (String ID){ //DELETE
SQLiteDatabase db = this.getWritableDatabase();
return db.delete(TABLE_NAME, "ID = ?", new String[] {ID});
}
}
主要活動:
public class MainActivity extends AppCompatActivity {
DatabaseHelper myDb;
EditText editTitle, editDescription, editOwner, editTextID;
Button btnAddData, btnviewAll, btnviewUpdate, btnDelete, btnLogOut;
ImageView mImageView;
final int REQUEST_CODE_GALLERY = 999;
//use lines 37 - 40 when starting a new activity
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
myDb = new DatabaseHelper(this); /*calling constructor*/
editTitle = (EditText)findViewById(R.id.editText_title);
editDescription = (EditText)findViewById(R.id.editText_description);
editOwner = (EditText)findViewById(R.id.editText_owner);
editTextID = (EditText)findViewById(R.id.editText_id);
btnAddData = (Button)findViewById(R.id.button_add);
btnviewAll = (Button)findViewById(R.id.button_viewAll);
btnviewUpdate = (Button)findViewById(R.id.button_update);
btnDelete = (Button)findViewById(R.id.button_delete);
btnLogOut = (Button)findViewById(R.id.button_logout);
mImageView = (ImageView)findViewById(R.id.imageView);
AddData();
viewAll();
UpdateGarment();
DeleteGarment();
btnLogOut.setOnClickListener( new View.OnClickListener(){
@Override
public void onClick(View view){
launchLoginActivity();
}
}
); // Learn2Crack - Android Switching Between Activities
mImageView.setOnClickListener(
new View.OnClickListener() {
@Override
public void onClick(View v) {
//read external storage permissions to select image from gallery
// Runtime permission for devices Android 6.0 and above
ActivityCompat.requestPermissions(
MainActivity.this, new String[]{Manifest.permission.READ_EXTERNAL_STORAGE},
REQUEST_CODE_GALLERY
);
}
});
}
//back to login
public void launchLoginActivity(){
Intent intent = new Intent (this, LoginActivity.class);
startActivity(intent);
} // Learn2Crack - Android Switching Between Activities
//Create
public void AddData(){
btnAddData.setOnClickListener(
new View.OnClickListener(){
@Override
public void onClick(View v){
boolean isInserted = myDb.insertData(editTitle.getText().toString(), editDescription.getText().toString(),
editOwner.getText().toString(), *mImageView.*);
if (isInserted == true)
Toast.makeText(MainActivity.this, "Garment Inserted", Toast.LENGTH_LONG).show();
else
Toast.makeText(MainActivity.this, "Garment Not Inserted", Toast.LENGTH_LONG).show(); }
});
} /*end of add button*/
//Retrieve
public void viewAll(){
btnviewAll.setOnClickListener(
new View.OnClickListener() {
@Override
public void onClick(View v) { /*the actual action goes in here */
Cursor res = myDb.getAllData();
if (res.getCount() == 0){
//show message
showMessage("Error", "No Garments Found");
return;
}
StringBuffer buffer = new StringBuffer();
while ( res.moveToNext()){
buffer.append("ID :" + res.getString(0)+ "\n");
buffer.append("TITLE :" + res.getString(1)+ "\n");
buffer.append("DESCRIPTION :" + res.getString(2)+ "\n");
buffer.append("OWNER :" + res.getString(3)+ "\n\n");
}
//show all data
showMessage("Garments", buffer.toString());
}
}
);
}
//Update
public void UpdateGarment(){
btnviewUpdate.setOnClickListener(
new View.OnClickListener(){
@Override
public void onClick(View v){
boolean isUpdate = myDb.updateData(editTextID.getText().toString(), editTitle.getText().toString(),
editDescription.getText().toString(), editOwner.getText().toString() );
if (isUpdate == true)
Toast.makeText(MainActivity.this, "Garment Updated", Toast.LENGTH_LONG).show();
else
Toast.makeText(MainActivity.this, "Garment Not Updated", Toast.LENGTH_LONG).show();
}
}
);
}
//Delete
public void DeleteGarment(){
btnDelete.setOnClickListener(
new View.OnClickListener() {
@Override
public void onClick(View v) {
Integer deletedRows = myDb.deleteData(editTextID.getText().toString());
if (deletedRows > 0)
Toast.makeText(MainActivity.this, "Garment Deleted", Toast.LENGTH_LONG).show();
else
Toast.makeText(MainActivity.this, "Garment Not Deleted", Toast.LENGTH_LONG).show();
}
}
);
}
public void showMessage(String title, String message){
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setCancelable(true);
builder.setTitle(title);
builder.setMessage(message);
builder.show();
}
//Gallary permission
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
if (requestCode == REQUEST_CODE_GALLERY){
if (grantResults.length>0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
//gallery intent
Intent galleryIntent = new Intent(Intent.ACTION_GET_CONTENT);
galleryIntent.setType("image/*");
startActivityForResult(galleryIntent, REQUEST_CODE_GALLERY);
}
else {
Toast.makeText(this, "Don't have permission to access file location", Toast.LENGTH_SHORT).show();
}
return;
}
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
}
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
if(requestCode == REQUEST_CODE_GALLERY && resultCode == RESULT_OK) {
Uri imageUri = data.getData();
CropImage.activity(imageUri)
.setGuidelines(CropImageView.Guidelines.ON)
.setAspectRatio(1,1)
.start(this);
}
if (requestCode == CropImage.CROP_IMAGE_ACTIVITY_REQUEST_CODE){
CropImage.ActivityResult result = CropImage.getActivityResult(data);
if (resultCode == RESULT_OK){
Uri resultUri = result.getUri();
//set image chosen from gallery to image view
mImageView.setImageURI(resultUri);
}
else if (resultCode == CropImage.CROP_IMAGE_ACTIVITY_RESULT_ERROR_CODE){
Exception error = result.getError();
}
}
super.onActivityResult(requestCode, resultCode, data);
}
}
您需要轉換mImageView。 到一個字節[](位圖)。
所以
BitmapDrawable d = (BitmapDrawable) mImageView.getDrawable();
Bitmap b = d.getBitmap();
boolean isInserted = myDb.insertData(editTitle.getText().toString(), editDescription.getText().toString(),
editOwner.getText().toString(), b);
如果位圖大於 2Mb,則無法從 Cursor 獲取。 不建議將位圖存儲在數據庫中,而是將位圖存儲為文件並將路徑存儲在數據庫中。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.