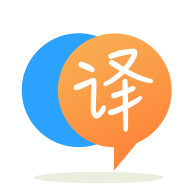
[英]Javascript setter - How to get the name of the setter inside of the setter?
[英]How to get getter/setter name in JavaScript/TypeScript?
獲取函數名稱非常簡單:
const func1 = function() {}
const object = {
func2: function() {}
}
console.log(func1.name);
// expected output: "func1"
console.log(object.func2.name);
// expected output: "func2"
但是,如何獲取 getter/setter 的字符串名稱?
class Example {
get hello() {
return 'world';
}
}
const obj = new Example();
重要的提示:
我不希望使用硬編碼字符串:
Object.getOwnPropertyDescriptor(Object.getPrototypeOf(obj), 'hello')
但是獲取名稱,例如:
console.log(getGetterName(obj.hello))
// expected output: "hello"
該語法設置了hello
屬性描述符的get
函數,因此函數的名稱將始終為get
您可以使用Object.getOwnPropertyDescriptor()
檢查hello
屬性是否在其屬性描述符上具有get
函數。
class Example { get hello() { return 'world'; } } /* compiles to / runs as var Example = (function () { function Example() { } Object.defineProperty(Example.prototype, "hello", { get: function () { return 'world'; }, enumerable: true, configurable: true }); return Example; }()); */ const des = Object.getOwnPropertyDescriptor(Example.prototype, 'hello'); console.log(des.get.name); // get (will always be 'get') // to check if 'hello' is a getter function isGetter(name) { const des = Object.getOwnPropertyDescriptor(Example.prototype, name); return !!des && !!des.get && typeof des.get === 'function'; } console.log(isGetter('hello')); // true
聽起來這不會解決您的最終問題,但是: Object.getOwnPropertyDescriptor(Example.prototype, 'hello').get.name
100% 回答了“如何在 JavaScript/TypeScript 中獲取 getter/setter 名稱?”的問題。 它永遠是“得到”
編輯:
一旦你調用obj.hello
,getter 就已經被調用了,你所擁有的只是原始結果,但是你可以在屬性值本身上使用元數據。
function stringPropertyName() { let _internal; return (target, key) => { Object.defineProperty(target, key, { get: () => { const newString = new String(_internal); Reflect.defineMetadata('name', key, newString); return newString; }, set: value => { _internal = value; } }); }; } class Example1 { @stringPropertyName() hello = 'world'; } const obj1 = new Example1(); console.log(Reflect.getMetadata('name', obj1.hello)); // hello class Example2 { _hello = 'world'; get hello() { const newString = new String(this._hello); Reflect.defineMetadata('name', 'hello', newString); return newString; } set hello(value) { this._hello = value; } } const obj2 = new Example2(); console.log(Reflect.getMetadata('name', obj2.hello)); // hello
<script src="https://cdnjs.cloudflare.com/ajax/libs/core-js/2.6.11/core.min.js"></script>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.