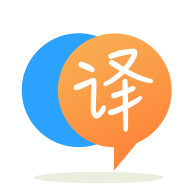
[英]Java How to split array into two different arrays, and then add a number to the arrays
[英]How to split file input into 2 different arrays java
如何將文件輸入文本拆分為 2 個不同的數組? 我想為姓名創建 n 個數組,並為電話號碼創建一個數組。 我設法進行文件輸入,但我嘗試了所有方法,但似乎無法拆分名稱和數字,然后將其放入 2 個不同的數組中。 我是菜鳥請幫忙
這是 phonebook.txt 文件的樣子
斌阿里,1110001111
亞歷克斯·卡德爾,8943257000
Poh Caimon,3247129843
迭戈·阿梅斯基塔,1001010000
泰麥舒,7776665555
喲瑪多,1110002233
Caup Sul,5252521551
這家伙,7776663333
我和我,0009991221
賈斯汀百里香,1113332222
嘿哦,3939399339
自由人,4533819911
彼得·派博,6480013966
威廉姆洛克,9059671045
下面是我的代碼
import java.io.*;
import java.util.Scanner;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.BufferedReader;
public class demos {
public static void main(String[] args){
FileInputStream Phonebook;
DataInputStream In;
int i = 0;
String fileInput;
try
{
Phonebook = new FileInputStream("phonebook.txt");
FileReader fr = new FileReader("phonebook.txt");
BufferedReader br = new BufferedReader(fr);
String buffer;
String fulltext="";
while ((buffer = br.readLine()) != null) {
fulltext += buffer;
// System.out.println(buffer);
String names = buffer;
char [] Y ;
Y = names.toCharArray();
System.out.println(Y);
}}
catch (FileNotFoundException e)
{
System.out.println("Error - this file does not exist");
}
catch (IOException e)
{
System.out.println("error=" + e.toString() );
}
如果您使用的是 Java 8,您可以簡化它:
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.util.ArrayList;
public class Test {
static ArrayList<String> names = new ArrayList<String>();
static ArrayList<String> numbers = new ArrayList<String>();
/**
* For each line, split it on the comma and send to splitNameAndNum()
*/
public static void main(String[] args) throws IOException {
Files.lines(new File("L:\\phonebook.txt").toPath())
.forEach(l -> splitNameAndNum(l.split(",")));
}
/**
* Accept an array of length 2 and put in the proper ArrayList
*/
public static void splitNameAndNum(String[] arr) {
names.add(arr[0]);
numbers.add(arr[1]);
}
}
在 Java 7 中:
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.ArrayList;
public class Test {
static ArrayList<String> names = new ArrayList<String>();
static ArrayList<String> numbers = new ArrayList<String>();
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream("L:\\phonebook.txt")));
String line;
while ((line = br.readLine()) != null) {
splitNameAndNum(line.split(","));
}
}
/**
* Accept an array of length 2 and put in the proper ArrayList
*/
public static void splitNameAndNum(String[] arr) {
names.add(arr[0]);
numbers.add(arr[1]);
}
}
對於完整的功能(而不是命令式)解決方案,我建議您使用以下解決方案:
public static void main(String[] args) throws IOException {
Object[] names = Files.lines(new File("phonebook.txt").toPath()).map(l -> l.split(",")[0]).toArray();
Object[] numbers = Files.lines(new File("phonebook.txt").toPath()).map(l -> l.split(",")[1]).toArray();
System.out.println("names in the file are : ");
Arrays.stream(names).forEach(System.out::println);
System.out.println("numbers in the file are : ");
Arrays.stream(numbers).forEach(System.out::println);
}
names in the file are : Bin Arry Alex Cadel Poh Caimon Diego Amezquita Tai Mai Shu Yo Madow Caup Sul This Guy Me And I Justin Thyme Hey Oh Free Man Peter Piper William Mulock numbers in the file are : 1110001111 8943257000 3247129843 1001010000 7776665555 1110002233 5252521551 7776663333 0009991221 1113332222 3939399339 4533819911 6480013966 9059671045
輸出names in the file are : Bin Arry Alex Cadel Poh Caimon Diego Amezquita Tai Mai Shu Yo Madow Caup Sul This Guy Me And I Justin Thyme Hey Oh Free Man Peter Piper William Mulock numbers in the file are : 1110001111 8943257000 3247129843 1001010000 7776665555 1110002233 5252521551 7776663333 0009991221 1113332222 3939399339 4533819911 6480013966 9059671045
正如你所看到的,函數式編程既簡短又聰明…… 當你習慣時很容易
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.