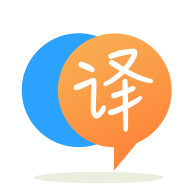
[英]how to show the hidden <dl> elements one by one or all at the same time?
[英]Show all the routes at the same time
我有 2 分,即 A & B
我正在 Google 地圖上繪制位於 A 點和 B 點之間的所有交付點。
這里實現的主要目標是在地圖上同時顯示所有這些多條路線,縮放應該足夠好以顯示地圖上的所有路線。
這是一個工作代碼:
<!DOCTYPE html>
<html>
<head>
<title>Google Map</title>
<script src="https://maps.googleapis.com/maps/api/js?key=API_KEY&libraries=places"></script>
<script type="text/javascript">
var map;
var origin = { lat: 40.6159134, lng: -74.0234863 };
var destination = { lat: 40.9132885, lng: -73.8340076 };
var locations = [
{ loadingLat: 40.8499301, loadingLng: -73.8449108, deliveryLat: 40.887934, deliveryLng: -73.828483 },
{ loadingLat: 40.7702993, loadingLng: -73.8792667, deliveryLat: 40.8246079, deliveryLng: -73.8713595 },
{ loadingLat: 40.7246827, loadingLng: -73.9347472, deliveryLat: 40.7567285, deliveryLng: -73.9142167 }
];
function addMarker(lat, lng, type, map) {
var coordinates = { lat: lat, lng: lng };
//var icon = 'AA.png';
var infoWindowContent = '<div><b>Pickup Point:</b> '+type+'</div>';
if(type == 'delivery'){
//icon = 'BB.png';
infoWindowContent = '<div><b>Delivery Point:</b> '+type+'</div>';
}
var marker = new google.maps.Marker({
position: coordinates,
map: map,
animation:google.maps.Animation.DROP
});
var infowindow = new google.maps.InfoWindow({
content: infoWindowContent
});
marker.addListener('click', function() {
infowindow.open(map, marker);
});
//infowindw.open(map, marker);
};
function initialize(){
var map = new google.maps.Map(document.getElementById("map_canvas"),{
//zoom: 14,
center: { lat: origin.lat, lng: origin.lng }
});
function renderDirections(result) {
var directionsRenderer = new google.maps.DirectionsRenderer;
directionsRenderer.setOptions({suppressMarkers: true});
directionsRenderer.setMap(map);
directionsRenderer.setDirections(result);
}
var directionsService = new google.maps.DirectionsService;
function requestDirections(loadingLat, loadingLng, deliveryLat, deliveryLng) {
directionsService.route({
origin: { lat: loadingLat, lng: loadingLng },
destination: { lat: deliveryLat, lng: deliveryLng },
travelMode: google.maps.DirectionsTravelMode.DRIVING
},
function(result) {
addMarker(loadingLat, loadingLng, 'pickup', map);
addMarker(deliveryLat, deliveryLng, 'delivery', map);
renderDirections(result);
});
}
requestDirections(origin.lat, origin.lng, destination.lat, destination.lng);
for(var i = 0; i < locations.length; i++){
requestDirections(locations[i].loadingLat, locations[i].loadingLng, locations[i].deliveryLat, locations[i].deliveryLng);
}
}
</script>
</head>
<body onLoad="initialize();">
<div id="map_canvas" style="width:1000px; height: 900px; margin:auto; background-color: #09F;"></div>
</body>
</html>
這里唯一的問題是地圖縮放是完全隨機的,它不會同時顯示所有路線。
DirectionsRenderer
自動縮放地圖以適應邊界。 這就是導致您看到的“隨機”縮放行為的原因。 路線服務是異步的,地圖左縮放到最后渲染的地圖,這並不總是相同的。
如果您發出多個路線請求,並且希望最終結果顯示所有路線,請將地圖縮放到路線邊界的聯合。
function renderDirections(result) {
var directionsRenderer = new google.maps.DirectionsRenderer;
directionsRenderer.setOptions({
suppressMarkers: true,
preserveViewport: true // <============================== prevent the "auto" zoom
});
directionsRenderer.setMap(map);
directionsRenderer.setDirections(result);
// calculate the union of bounds
if (bounds.isEmpty()) bounds=result.routes[0].bounds;
else bounds.union(result.routes[0].bounds);
map.fitBounds(bounds); // zoom the map to fit the union of the bounds
}
代碼片段:
/* Always set the map height explicitly to define the size of the div * element that contains the map. */ #map_canvas { height: 100%; background-color: #09F; } /* Optional: Makes the sample page fill the window. */ html, body { height: 100%; margin: 0; padding: 0; }
<script async defer src="https://maps.googleapis.com/maps/api/js?key=AIzaSyCkUOdZ5y7hMm0yrcCQoCvLwzdM6M8s5qk&libraries=places&callback=initialize"></script> <script type="text/javascript"> var map; var bounds; var origin = { lat: 40.6159134, lng: -74.0234863 }; var destination = { lat: 40.9132885, lng: -73.8340076 }; var locations = [ {loadingLat: 40.8499301, loadingLng: -73.8449108, deliveryLat: 40.887934, deliveryLng: -73.828483 }, {loadingLat: 40.7702993, loadingLng: -73.8792667, deliveryLat: 40.8246079, deliveryLng: -73.8713595 }, {loadingLat: 40.7246827, loadingLng: -73.9347472, deliveryLat: 40.7567285, deliveryLng: -73.9142167 } ]; function addMarker(lat, lng, type, map) { var coordinates = { lat: lat, lng: lng }; var infoWindowContent = '<div><b>Pickup Point:</b> ' + type + '</div>'; if (type == 'delivery') { infoWindowContent = '<div><b>Delivery Point:</b> ' + type + '</div>'; } var marker = new google.maps.Marker({ position: coordinates, map: map, animation: google.maps.Animation.DROP }); var infowindow = new google.maps.InfoWindow({ content: infoWindowContent }); marker.addListener('click', function() { infowindow.open(map, marker); }); }; function initialize() { var map = new google.maps.Map(document.getElementById("map_canvas"), { center: { lat: origin.lat, lng: origin.lng } }); bounds = new google.maps.LatLngBounds(); function renderDirections(result) { var directionsRenderer = new google.maps.DirectionsRenderer; directionsRenderer.setOptions({ suppressMarkers: true, preserveViewport: true }); directionsRenderer.setMap(map); directionsRenderer.setDirections(result); if (bounds.isEmpty()) bounds = result.routes[0].bounds; else bounds.union(result.routes[0].bounds); map.fitBounds(bounds); } var directionsService = new google.maps.DirectionsService(); function requestDirections(loadingLat, loadingLng, deliveryLat, deliveryLng) { directionsService.route({ origin: { lat: loadingLat, lng: loadingLng }, destination: { lat: deliveryLat, lng: deliveryLng }, travelMode: google.maps.DirectionsTravelMode.DRIVING }, function(result) { addMarker(loadingLat, loadingLng, 'pickup', map); addMarker(deliveryLat, deliveryLng, 'delivery', map); renderDirections(result); }); } requestDirections(origin.lat, origin.lng, destination.lat, destination.lng); for (var i = 0; i < locations.length; i++) { requestDirections(locations[i].loadingLat, locations[i].loadingLng, locations[i].deliveryLat, locations[i].deliveryLng); } } </script> <div id="map_canvas"></div>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.