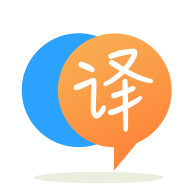
[英]Modifying javascript to maintain HTML dropdown menu selection in Flask
[英]ReactJS - JavaScript - HTML: How to update a dropdown menu after selection
問題:選擇后如何更新下拉菜單?
對不起,如果這個問題很簡單,但我一直在嘗試許多不同的方法來使它起作用。 我正在使用AISHub API構建一個船可視化器。 在查詢 API 后,我可以獲得一個包含我感興趣的船只的 json 文件,並將這些船只注入表格中,並在google-map
上顯示它們的標記。 現在一些船只做一些功能,其他人做其他事情,我正在嘗試使用dropdown
菜單過濾它。
下面是代碼最重要的部分:
谷歌地圖.js :
class BoatMap extends Component {
constructor(props) {
super(props);
this.state = {
buttonEnabled: true,
buttonClickedAt: null,
progress: 0,
ships: []
};
this.updateRequest = this.updateRequest.bind(this);
this.countDownInterval = null;
}
componentDidMount() {
this.countDownInterval = setInterval(() => {
if (!this.state.buttonClickedAt) return;
const date = new Date();
const diff = Math.floor((date.getTime() - this.state.buttonClickedAt.getTime()) / 1000);
if (diff < 90) {
this.setState({
progress: diff,
buttonEnabled: false
});
} else {
this.setState({
progress: 0,
buttonClickedAt: null,
buttonEnabled: true
});
}
}, 500);
}
componentWillUnmount() {
clearInterval(this.countdownInterval);
}
async updateRequest() {
const url = 'http://localhost:3001/hello';
console.log(url);
const fetchingData = await fetch(url);
const ships = await fetchingData.json();
console.log(ships);
this.setState({
buttonEnabled: false,
buttonClickedAt: new Date(),
progress: 0,
ships
});
setTimeout(() => {
this.setState({ buttonEnabled: true });
});
}
render() {
return (
<div className="google-map">
<GoogleMapReact
bootstrapURLKeys={{ key: 'My_KEY' }}
center={{
lat: 42.4,
lng: -71.1
}}
zoom={8}
>
// This will render the customized marker on Google-Map
{this.state.ships.map((ship) => (
<Ship ship={ship} key={ship.CALLSIGN} lat={ship.LATITUDE} lng={ship.LONGITUDE} />
))}
// This is the dropdown I am trying to update
<select className="combo-companies">
<option value="All">All</option>
<option value="research">research</option>
<option value="exploration">exploration</option>
<option value="navigation">navigation</option>
<option value="drilling">drilling</option>
</select>
</GoogleMapReact>
</div>
);
}
}
ShipTracker.js
const Ship = ({ ship }) => {
const shipName = ship.NAME;
const company = shipCompanyMap[shipName];
const shipImage = companyImageMap[company];
return (
<div>
{/* Render shipImage image */}
<img src={shipImage} alt="Logo" />
</div>
);
};
export { Ship };
const ShipTracker = ({ ships }) => {
const handleRowClick = (rowValue) => {
console.log(rowValue);
};
return (
<div className="ship-tracker">
<Table className="flags-table" responsive hover>
<thead>
<tr>
<th>#</th>
<th>Name</th>
<th>Callsign</th>
<th>Heading</th>
<th>SOG</th>
<th>IMO</th>
<th>MMSI</th>
<th>Longitude</th>
<th>Latitudee</th>
</tr>
</thead>
<tbody>
{ships.map((ship, index) => {
const { IMO, NAME, CALLSIGN, HEADING, SOG, MMSI, LONGITUDE, LATITUDE } = ship;
const cells = [ NAME, CALLSIGN, HEADING, SOG, IMO, MMSI, LONGITUDE, LATITUDE ];
return (
<tr onClick={() => handleRowClick(ship)} key={index}>
<th scope="row">{index}</th>
{cells.map((cell) => <td>{cell}</td>)}
</tr>
);
})}
</tbody>
</Table>
</div>
);
};
export default ShipTracker;
到目前為止我做了什么:
1)經過調查,我發現了這個來源,它有助於理解選擇背后的概念。 這個問題仍然沒有答案,想知道是否必須實現componentWillMount
和componentWillReceiveProps
函數,或者是否有其他方法。
2) 這篇文章也很有用,但讓我有點困惑,因為似乎需要將setState
作為實現的附加函數。
3) 從這里看來,必須在處理程序函數中提供response
。 雖然有用,但我認為這篇文章是 1) + 2) 點的總和。
我知道這是一個簡單的例子,但我試圖理解在傳遞到更中級或高級的內容之前如何更新簡單dropdown
的基本概念。 感謝您指出解決此問題的正確方向。
好的,所以您想根據用戶在下拉列表中選擇的選項過濾地圖上顯示的制造商。
您可以根據需要更改type
名稱。 下拉值之一的類型(全部、研究、探索、導航或鑽探)。
我還沒有測試以下代碼,但它應該可以工作!
class BoatMap extends Component {
constructor(props) {
super(props);
this.state = {
buttonEnabled: true,
buttonClickedAt: null,
progress: 0,
ships: [],
type: 'All'
};
this.updateRequest = this.updateRequest.bind(this);
this.countDownInterval = null;
}
componentDidUpdate(prevProps, prevState) {
if (this.state.type !== prevState.type) {
// The user has selected a different value from the dropdown
// To get this value: this.state.type
// do anything you want here...
console.log('dropdown value changed for ' + this.state.type);
}
}
handleChange = e => {
this.setState({
type: e.target.value
});
};
render() {
return (
<div className="google-map">
<GoogleMapReact
bootstrapURLKeys={{key: 'My_KEY'}}
center={{
lat: 42.4,
lng: -71.1
}}
zoom={8}
>
{this.state.ships.filter(ship => ship.type === this.state.type).map((ship) => (
<Ship ship={ship} key={ship.CALLSIGN} lat={ship.LATITUDE} lng={ship.LONGITUDE}/>
))}
<select className="combo-companies" value={this.state.type} onChange={this.handleChange}>
<option value="All">All</option>
<option value="research">research</option>
<option value="exploration">exploration</option>
<option value="navigation">navigation</option>
<option value="drilling">drilling</option>
</select>
</GoogleMapReact>
</div>
);
}
}
filter(ship => ship.type === this.state.type)
我以 ship.type 為例。 你的 Ship 實體有 type 屬性嗎?
編輯:
render() {
// All the ships
const ships = this.state.ships;
// Debug
console.log('this.state.type: ' + this.state.type);
// Only the ships matching the dropdown selected value
const filteredShips = ships.filter(ship => {
console.log('ship.shipImage: ' + ship.shipImage);
if (ship.shipImage !== this.state.type) {
console.log('ship.shipImage does not match the filtered value');
}
return ship.shipImage === this.state.type;
});
// Debug
console.log(ships);
console.log(filteredShips);
return (
<div className="google-map">
<GoogleMapReact
bootstrapURLKeys={{key: 'My_KEY'}}
center={{
lat: 42.4,
lng: -71.1
}}
zoom={8}
>
{filteredShips.map((ship) => (
<Ship ship={ship} key={ship.CALLSIGN} lat={ship.LATITUDE} lng={ship.LONGITUDE}/>
))}
<select className="combo-companies" value={this.state.type} onChange={this.handleChange}>
<option value="All">All</option>
<option value="research">research</option>
<option value="exploration">exploration</option>
<option value="navigation">navigation</option>
<option value="drilling">drilling</option>
</select>
</GoogleMapReact>
</div>
);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.