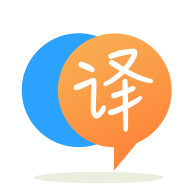
[英]java.net.UnknownHostException: Unable to resolve host "api.themoviedb.org
[英]Error: java.net.UnknownHostException: Unable to resolve host "api.themoviedb.org
編輯:模擬器沒有互聯網:/如果您遇到此問題,請嘗試轉到 AVD 管理器並擦除模擬器。
在添加適配器之前,我的代碼可以正常工作,但我已經對其進行了三次檢查,但我的 asynhttprequest 仍然無法正常工作(在它返回狀態代碼 200 以及結果和電影變量之前)。 任何想法都值得贊賞,我已經嘗試了模擬器和電話,所以我知道互聯網不是問題。
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.flixster">
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
MainActivity.java:
public class MainActivity extends AppCompatActivity {
public static final String NOW_PLAYING_URL = "https://api.themoviedb.org/3/movie/now_playing?api_key=a07e22bc18f5cb106bfe4cc1f83ad8ed";
public static final String TAG = "MainActivity";
List<Movie> movies;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
RecyclerView rvMovies = findViewById(R.id.rvMovies);
movies = new ArrayList<>();
// Create the adapter
final MovieAdapter movieAdapter = new MovieAdapter(this, movies);
// Set the adapter on the recycler view
rvMovies.setAdapter(movieAdapter);
// Set a Layout Manager on the recycler view
rvMovies.setLayoutManager(new LinearLayoutManager(this));
AsyncHttpClient client = new AsyncHttpClient();
client.get(NOW_PLAYING_URL, new JsonHttpResponseHandler() {
@Override
public void onSuccess(int statusCode, Headers headers, JSON json) {
Log.d(TAG, "onSuccess");
JSONObject jsonObject = json.jsonObject;
try {
JSONArray results = jsonObject.getJSONArray("results");
Log.i(TAG, "Results: " + results.toString());
movies.addAll(Movie.fromJsonArray(results));
// whenever data changes, rerender recycler view
//movieAdapter.notifyDataSetChanged();
Log.i(TAG, "Movies: " + movies.size());
} catch (JSONException e) {
Log.e(TAG, "Hit json exception", e);
e.printStackTrace();
}
}
@Override
public void onFailure(int statusCode, Headers headers, String response, Throwable throwable) {
Log.d(TAG, "onFailure");
}
});
}}
Movie.java
public class Movie {
String posterPath;
String title;
String overview;
public Movie (JSONObject jsonObject) throws JSONException {
posterPath = jsonObject.getString("poster_path");
title = jsonObject.getString("title");
overview = jsonObject.getString("overview");
}
// Iterates through data from results array returned by MainActivity.java into individual movie objects
public static List<Movie> fromJsonArray(JSONArray movieJsonArray) throws JSONException {
List<Movie> movies = new ArrayList<>();
for (int i = 0; i < movieJsonArray.length(); i++){
movies.add(new Movie(movieJsonArray.getJSONObject(i)));
}
return movies;
}
// TODO: figure out what sizes are available and then append to url before posterPath
public String getPosterPath() {
return String.format("https://image.tmdb.org/t/p/w342/%s", posterPath);
}
public String getTitle() {
return title;
}
public String getOverview() {
return overview;
}
}
MovieAdapter.java
public class MovieAdapter extends RecyclerView.Adapter<MovieAdapter.ViewHolder> {
Context context;
List<Movie> movies;
public MovieAdapter (Context context, List<Movie>movies) {
this.context = context;
this.movies = movies;
}
// Usually involves inflating a layout from XML and returning the holder
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View movieView = LayoutInflater.from(context).inflate(R.layout.item_movie, parent, false);
return new ViewHolder(movieView);
}
// Involves populating data into the item through holder
@Override
public void onBindViewHolder(@NonNull ViewHolder holder, int position) {
// Get the movie at the passed in position
Movie movie = movies.get(position);
// Bind the movie data into the viewholder
holder.bind(movie);
}
// Returns the total count of items in the list
@Override
public int getItemCount() {
return movies.size();
}
public class ViewHolder extends RecyclerView.ViewHolder {
TextView tvTitle;
TextView tvOverview;
ImageView ivPoster;
public ViewHolder(@NonNull View itemView) {
super(itemView);
tvTitle = itemView.findViewById(R.id.tvTitle);
tvOverview = itemView.findViewById(R.id.tvOverview);
ivPoster= itemView.findViewById(R.id.ivPoster);
}
public void bind(Movie movie) {
tvTitle.setText(movie.getTitle());
tvOverview.setText(movie.getOverview());
Glide.with(context).load(movie.getPosterPath()).into(ivPoster);
}
}
}
StackTrace
2020-02-11 22:47:53.186 28424-28424/? I/xample.flixste: Not late-enabling -Xcheck:jni (already on)
2020-02-11 22:47:53.444 28424-28424/? E/xample.flixste: Unknown bits set in runtime_flags: 0x8000
2020-02-11 22:47:53.539 28424-28424/? W/xample.flixste: Unexpected CPU variant for X86 using defaults: x86
2020-02-11 22:47:55.018 28424-28462/com.example.flixster D/libEGL: Emulator has host GPU support, qemu.gles is set to 1.
2020-02-11 22:47:55.012 28424-28424/com.example.flixster W/RenderThread: type=1400 audit(0.0:168): avc: denied { write } for name="property_service" dev="tmpfs" ino=274 scontext=u:r:untrusted_app:s0:c134,c256,c512,c768 tcontext=u:object_r:property_socket:s0 tclass=sock_file permissive=0
2020-02-11 22:47:55.022 28424-28462/com.example.flixster W/libc: Unable to set property "qemu.gles" to "1": connection failed; errno=13 (Permission denied)
2020-02-11 22:47:55.082 28424-28462/com.example.flixster D/libEGL: loaded /vendor/lib/egl/libEGL_emulation.so
2020-02-11 22:47:55.114 28424-28462/com.example.flixster D/libEGL: loaded /vendor/lib/egl/libGLESv1_CM_emulation.so
2020-02-11 22:47:55.133 28424-28462/com.example.flixster D/libEGL: loaded /vendor/lib/egl/libGLESv2_emulation.so
2020-02-11 22:47:55.769 28424-28424/com.example.flixster W/xample.flixste: Accessing hidden method Landroid/view/View;->computeFitSystemWindows(Landroid/graphics/Rect;Landroid/graphics/Rect;)Z (greylist, reflection, allowed)
2020-02-11 22:47:55.770 28424-28424/com.example.flixster W/xample.flixste: Accessing hidden method Landroid/view/ViewGroup;->makeOptionalFitsSystemWindows()V (greylist, reflection, allowed)
2020-02-11 22:47:56.241 28424-28424/com.example.flixster W/xample.flixste: Accessing hidden method Lcom/android/org/conscrypt/OpenSSLSocketImpl;->setUseSessionTickets(Z)V (greylist,core-platform-api, reflection, allowed)
2020-02-11 22:47:56.241 28424-28424/com.example.flixster W/xample.flixste: Accessing hidden method Lcom/android/org/conscrypt/OpenSSLSocketImpl;->setHostname(Ljava/lang/String;)V (greylist,core-platform-api, reflection, allowed)
2020-02-11 22:47:56.241 28424-28424/com.example.flixster W/xample.flixste: Accessing hidden method Lcom/android/org/conscrypt/OpenSSLSocketImpl;->getAlpnSelectedProtocol()[B (greylist,core-platform-api, reflection, allowed)
2020-02-11 22:47:56.241 28424-28424/com.example.flixster W/xample.flixste: Accessing hidden method Lcom/android/org/conscrypt/OpenSSLSocketImpl;->setAlpnProtocols([B)V (greylist,core-platform-api, reflection, allowed)
2020-02-11 22:47:56.241 28424-28424/com.example.flixster W/xample.flixste: Accessing hidden method Ldalvik/system/CloseGuard;->get()Ldalvik/system/CloseGuard; (greylist,core-platform-api, reflection, allowed)
2020-02-11 22:47:56.241 28424-28424/com.example.flixster W/xample.flixste: Accessing hidden method Ldalvik/system/CloseGuard;->open(Ljava/lang/String;)V (greylist,core-platform-api, reflection, allowed)
2020-02-11 22:47:56.241 28424-28424/com.example.flixster W/xample.flixste: Accessing hidden method Ldalvik/system/CloseGuard;->warnIfOpen()V (greylist,core-platform-api, reflection, allowed)
2020-02-11 22:47:56.242 28424-28424/com.example.flixster D/NetworkSecurityConfig: No Network Security Config specified, using platform default
2020-02-11 22:47:56.473 28424-28459/com.example.flixster D/HostConnection: HostConnection::get() New Host Connection established 0xe1632190, tid 28459
2020-02-11 22:47:56.491 28424-28459/com.example.flixster D/HostConnection: HostComposition ext ANDROID_EMU_CHECKSUM_HELPER_v1 ANDROID_EMU_native_sync_v2 ANDROID_EMU_native_sync_v3 ANDROID_EMU_native_sync_v4 ANDROID_EMU_dma_v1 ANDROID_EMU_direct_mem ANDROID_EMU_host_composition_v1 ANDROID_EMU_host_composition_v2 ANDROID_EMU_YUV420_888_to_NV21 ANDROID_EMU_YUV_Cache ANDROID_EMU_async_unmap_buffer GL_OES_EGL_image_external_essl3 GL_OES_vertex_array_object GL_KHR_texture_compression_astc_ldr ANDROID_EMU_gles_max_version_3_0
2020-02-11 22:47:56.597 28424-28459/com.example.flixster W/OpenGLRenderer: Failed to choose config with EGL_SWAP_BEHAVIOR_PRESERVED, retrying without...
2020-02-11 22:47:56.604 28424-28459/com.example.flixster D/eglCodecCommon: setVertexArrayObject: set vao to 0 (0) 0 0
2020-02-11 22:47:56.604 28424-28459/com.example.flixster D/EGL_emulation: eglCreateContext: 0xe161a120: maj 3 min 0 rcv 3
2020-02-11 22:47:56.609 28424-28459/com.example.flixster D/EGL_emulation: eglMakeCurrent: 0xe161a120: ver 3 0 (tinfo 0xe160f700)
2020-02-11 22:47:56.629 28424-28459/com.example.flixster W/Gralloc3: mapper 3.x is not supported
2020-02-11 22:47:56.633 28424-28459/com.example.flixster D/HostConnection: createUnique: call
2020-02-11 22:47:56.638 28424-28459/com.example.flixster D/HostConnection: HostConnection::get() New Host Connection established 0xe16322d0, tid 28459
2020-02-11 22:47:56.640 28424-28459/com.example.flixster D/HostConnection: HostComposition ext ANDROID_EMU_CHECKSUM_HELPER_v1 ANDROID_EMU_native_sync_v2 ANDROID_EMU_native_sync_v3 ANDROID_EMU_native_sync_v4 ANDROID_EMU_dma_v1 ANDROID_EMU_direct_mem ANDROID_EMU_host_composition_v1 ANDROID_EMU_host_composition_v2 ANDROID_EMU_YUV420_888_to_NV21 ANDROID_EMU_YUV_Cache ANDROID_EMU_async_unmap_buffer GL_OES_EGL_image_external_essl3 GL_OES_vertex_array_object GL_KHR_texture_compression_astc_ldr ANDROID_EMU_gles_max_version_3_0
2020-02-11 22:47:56.641 28424-28459/com.example.flixster D/eglCodecCommon: allocate: Ask for block of size 0x1000
2020-02-11 22:47:56.641 28424-28459/com.
example.flixster D/eglCodecCommon: allocate: ioctl allocate returned offset 0x3ff801000 size 0x2000
2020-02-11 22:47:56.666 28424-28459/com.example.flixster D/EGL_emulation: eglMakeCurrent: 0xe161a120: ver 3 0 (tinfo 0xe160f700)
2020-02-11 22:47:56.669 28424-28459/com.example.flixster D/eglCodecCommon: setVertexArrayObject: set vao to 0 (0) 1 0
2020-02-11 22:47:56.872 28424-28464/com.example.flixster D/MainActivity: onFailure
有兩種方式
1.將以下屬性添加到您的 AndroidManifest.xml 文件中。
android:usesCleartextTraffic="true"
原因
打算僅使用安全連接連接到目標的應用程序可以選擇不支持這些目標的明文(使用未加密的 HTTP 協議而不是 HTTPS)。 此選項有助於防止由於后端服務器等外部來源提供的 URL 發生變化而導致應用程序意外回歸
2.將 DNS 添加到您的 okhttp 客戶端。
return OkHttpClient.Builder()
.dns(Dns.SYSTEM)
.connectTimeout(60, TimeUnit.SECONDS)
.readTimeout(60, TimeUnit.SECONDS)
.build()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.