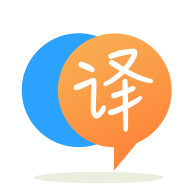
[英]Is it possible to create a type alias that has trait bounds on a generic type for a function?
[英]Is it possible to create a generic impl for a trait that works with all but one subset of types?
我正在嘗試編寫一個通用方法,該方法接受一個返回Serialize
值或Arc<Serialize>
值的函數。 我的解決方案是創建一個特征來在需要時解開Arc
並生成對基礎值的引用:
use serde::Serialize;
use std::sync::Arc;
pub trait Unwrapper {
type Inner: Serialize;
fn unwrap(&self) -> &Self::Inner;
}
impl<T> Unwrapper for T
where
T: Serialize,
{
type Inner = T;
fn unwrap(&self) -> &Self::Inner {
self
}
}
impl<T> Unwrapper for Arc<T>
where
T: Serialize,
{
type Inner = T;
fn unwrap(&self) -> &Self::Inner {
self
}
}
fn use_processor<F, O>(processor: F)
where
O: Unwrapper,
F: Fn() -> O,
{
// do something useful processor
}
由於Arc
將來可能會實現Serialize
,我收到了E0119
錯誤,就像我啟用 serde crate 的功能一樣:
error[E0119]: conflicting implementations of trait `Unwrapper` for type `std::sync::Arc<_>`:
--> src/lib.rs:20:1
|
10 | / impl<T> Unwrapper for T
11 | | where
12 | | T: Serialize,
13 | | {
... |
17 | | }
18 | | }
| |_- first implementation here
19 |
20 | / impl<T> Unwrapper for Arc<T>
21 | | where
22 | | T: Serialize,
23 | | {
... |
27 | | }
28 | | }
| |_^ conflicting implementation for `std::sync::Arc<_>`
我不想這樣做,因為我只想允許Arc
在頂層而不是在值內(出於同樣的原因,默認情況下該功能未啟用)。 鑒於此,有沒有辦法僅針對Arc
禁用我的第一個impl
? 或者有沒有更好的方法來解決問題?
您的嘗試不起作用,因為不可能有一個特征的重疊實現。
下面試圖編寫接受泛型方法Serialize
值或Arc
一的Serialize
值。
它利用了Borrow
特性及其對任何 T 的全面實現。
請注意在泛型方法的調用站點上使用 turbo fish 語法。
use std::sync::Arc;
use std::borrow::Borrow;
use serde::Serialize;
#[derive(Serialize, Debug)]
struct Point {
x: i32,
y: i32,
}
fn myserialize<T: Borrow<I>, I: Serialize>(value: T) {
let value = value.borrow();
let serialized = serde_json::to_string(value).unwrap();
println!("serialized = {}", serialized);
}
fn main() {
let point = Point { x: 1, y: 2 };
myserialize(point);
let arc_point = Arc::new(Point { x: 1, y: 2 });
myserialize::<_, Point>(arc_point);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.