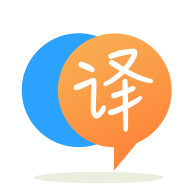
[英]Java recursive method to print one word in a string at a time without loops
[英]How to print out longest word in string WITHOUT using arrays and only loops in Java
目前,我能夠弄清楚如何顯示最長的字長。 但是,有沒有辦法使用循環來確定最長的單詞並將其打印出來?
public static void longestWordCalculator(String rawText)
{
int lengthCounter = 0;
int finalCounter = 0;
int textLength = rawText.length();
for (int i = 0; i < textLength ; i++)
{
String indexValue = Character.toString(rawText.charAt(i));
if(!" ".equals(indexValue))
{
lengthCounter++;
}
else
{
finalCounter = Math.max(finalCounter, lengthCounter);
lengthCounter = 0;
}
}
System.out.println("Final Value: " + Math.max(finalCounter, lengthCounter));
}
這是一個沒有拆分的解決方案:
public class LongestWord {
public static void longestWordCalculator(String rawText)
{
int lastSeparator = -1;
int maxLength = 0;
int solPosition = 0;
int textLength = rawText.length();
for (int i = 0; i < textLength ; i++)
{
//here you may check for other separators
if (rawText.charAt(i) == ' ') {
lastSeparator = i;
}
//assuming no separator is part of a word
else {
if (i - lastSeparator > maxLength) {
maxLength = i - lastSeparator;
solPosition = lastSeparator;
}
}
}
if (maxLength > 0) {
String solution = rawText.substring(solPosition+1, solPosition+maxLength+1);
System.out.println("Longest word is: " + solution);
}
else {
System.out.println("No solution finded!");
}
}
public static void main (String args[]) {
longestWordCalculator("la casa de mi amigo");
longestWordCalculator("quiero agua");
longestWordCalculator("bblablabla");
longestWordCalculator("");
}
}
輸出:
Longest word is: amigo
Longest word is: quiero
Longest word is: bblablabla
No solution finded!
這個解決方案很巧妙,它實際上會打印出所有長度最長的單詞,而不是一個。
public class Main {
public static void main(String[] args) {
String string = "one two three four five six seven eight nine";
String currentWord = "";
String largestWords = "";
int longestLength = 0;
for (int i = 0; i < string.length(); i++) {
char iteratedLetter = string.charAt(i);
if (iteratedLetter == ' ') { // previous word just ended.
if (currentWord.length() > longestLength) {
largestWords = "";
longestLength = currentWord.length();
}
else if (currentWord.length() == longestLength) {
largestWords += currentWord + " ";
}
currentWord = "";
}
else {
currentWord += iteratedLetter;
}
}
System.out.println("Largest words: " + largestWords);
}
}
我鼓勵理解代碼,盡管正如您所說,這是一項任務。
可以使用split()
將字符串拆分為字符串數組,然后循環遍歷每個元素來檢查單詞的長度。 在此示例中,我們假設沒有特殊字符且單詞由空格分隔。
該函數將找到第一個最長的單詞,即如果有平局,它將輸出長度最長的第一個單詞。 在下面的示例中,您可以看到looking
和longest
都有相同數量的字符,但它只會輸出looking
。
public static void longestWordCalculator(String rawText)
{
int textLength = rawText.length();
String longestWord = "";
String[] words = rawText.split("\\s");
for (int i = 0; i < words.length; i++)
{
if (words[i].length() > longestWord.length()) {
longestWord = words[i];
}
}
System.out.println("Longest word: " + longestWord);
System.out.println("With length of: " + longestWord.length());
}
用法: longestWordCalculator("Hello I am looking for the longest word");
輸出
最長的詞:看
長度為:7
編輯:
不使用數組:
public static void longestWordCalculator(String rawText)
{
int nextSpaceIndex = rawText.indexOf(" ") + 1;
String longestWord = "";
do {
String word = rawText.substring(0, nextSpaceIndex);
rawText = rawText.substring(nextSpaceIndex); // trim string
if (word.length() > longestWord.length()) {
longestWord = word;
}
int tempNextIndex = rawText.indexOf(" ") + 1;
nextSpaceIndex = tempNextIndex == 0 ? rawText.length() : tempNextIndex;
} while (rawText.length() > 0);
System.out.println("Longest word: " + longestWord);
System.out.println("With length of: " + longestWord.length());
}
按空格字符拆分文本並找到最長的單詞。 試試這個代碼。
public class Test {
public static void longestWordCalculator(String rawText) {
String[] words = rawText.trim().replaceAll(" +", " ").split(" ");
int foundIndex = -1;
int maxLenght = 0;
String foundWord = "";
for (int i = 0; i < words.length; i++) {
if (words[i].length() > maxLenght) {
maxLenght = words[i].length();
foundWord = words[i];
foundIndex = i;
}
}
System.out.println(String.format("Longest word is [Word=%s, WordLength=%s, WordIndex=%s]", foundWord, maxLenght, foundIndex));
}
public static void main(String args[]) {
longestWordCalculator("It looks good");
}
}
輸入:“看起來不錯”
輸出:最長的單詞是 [Word=looks, WordLength=5, WordIndex=1]
使用Pattern
和Matcher
以及while
循環。 就像是,
public static void longestWordCalculator(String rawText) {
Pattern p = Pattern.compile("(\\S+)\\b");
Matcher m = p.matcher(rawText);
String found = null;
while (m.find()) {
String s = m.group(1);
if (found == null || s.length() > found.length()) {
found = s;
}
}
if (found == null) {
System.out.println("No words found");
} else {
System.out.printf("The longest word in \"%s\" is %s which is %d characters.%n",
rawText, found, found.length());
}
}
發布的兩個答案很好,我會使用它們,但是,如果您想保留當前的實現並避免使用數組等,則可以在使用longestIndex = i - lengthCounter
保存新的最長長度時保存當前最長字符串的開始位置longestIndex = i - lengthCounter
。 最后,打印出rawText
從longestIndex
到longestIndex + finalCounter
的子字符串。
編輯 - 嘗試這樣的事情
int lengthCounter = 0;
int finalCounter = 0;
int textLength = rawText.length();
int longestIndex = 0;
for (int i = 0; i < textLength ; i++)
{
String indexValue = Character.toString(rawText.charAt(i));
if(!" ".equals(indexValue))
{
lengthCounter++;
}
else
{
if (lengthCounter > finalCounter) {
longestIndex = i - lengthCounter;
finalCounter = lengthCounter;
}
lengthCounter = 0;
}
}
System.out.println("Final Value: " + finalCounter);
System.out.println("Longest Word: " + rawText.substring(longestIndex, longestIndex + finalCounter));
我保留您的代碼,而不是使用數組。 這是更新的代碼:
public class Test {
public static void longestWordCalculator(String rawText) {
int lengthCounter = 0;
int finalCounter = 0;
int textLength = rawText.length();
StringBuffer processingWord = new StringBuffer();
String foundWord = null;
for (int i = 0; i < textLength; i++) {
String indexValue = Character.toString(rawText.charAt(i));
if (!" ".equals(indexValue)) {
processingWord.append(rawText.charAt(i));
lengthCounter++;
} else {
if (finalCounter < lengthCounter) {
finalCounter = lengthCounter;
foundWord = processingWord.toString();
processingWord = new StringBuffer();
}
lengthCounter = 0;
}
}
System.out.println("Final Value: " + finalCounter + ", Word: " + foundWord);
}
public static void main(String args[]) {
longestWordCalculator("It looks good");
}
}
希望它能有所幫助。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.