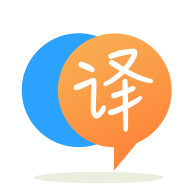
[英]Using std::unique_ptr.reset() to change the object under pointer
[英]Why std::move() of a unique_ptr to a void function to reset the pointer takes less time than call explicit unique_ptr.reset()
我制作了這些 PoC 來找出釋放 unique_ptr 內存的最快方法:
#include <iostream>
#include <ctime>
#include <ratio>
#include <chrono>
#include <cstdlib>
#include <memory>
void freePointer(std::unique_ptr<int> aPointer){
// The unique ptr memory is released here
}
int main ()
{
using namespace std::chrono;
// POC1
std::unique_ptr<int> pointer (new int(3));
high_resolution_clock::time_point t1 = high_resolution_clock::now();
pointer.reset();
high_resolution_clock::time_point t2 = high_resolution_clock::now();
duration<double> time_span = duration_cast<duration<double>>(t2 - t1);
std::cout << "POC1: It took me " << time_span.count() << " seconds." << std::endl;
// POC2
std::unique_ptr<int> pointer2 (new int(3));
high_resolution_clock::time_point t3 = high_resolution_clock::now();
freePointer(std::move(pointer2));
high_resolution_clock::time_point t4 = high_resolution_clock::now();
duration<double> time_span2 = duration_cast<duration<double>>(t4 - t3);
std::cout << "POC2: It took me " << time_span2.count() << " seconds." << std::endl;
return 0;
}
輸出是:
POC1:我花了 2.573e-06 秒。
POC2:我花了 5.07e-07 秒。
PoX 之間有一個數量級的差異,我不明白原因。
有人能給我一盞燈嗎?
#include <iostream>
#include <ctime>
#include <ratio>
#include <chrono>
#include <cstdlib>
#include <memory>
#include <vector>
void freePointer(std::unique_ptr<int> aPointer){
// The unique ptr memory is released here
}
int main ()
{
using namespace std::chrono;
// POC1
std::vector<std::unique_ptr<int>> pointers;
for(int i=0;i<10000;++i) {
pointers.emplace_back(new int(3));
}
high_resolution_clock::time_point t1 = high_resolution_clock::now();
for(int i=0;i<10000;++i) {
pointers[i].reset();
}
high_resolution_clock::time_point t2 = high_resolution_clock::now();
duration<double> time_span = duration_cast<duration<double>>(t2 - t1);
std::cout << "POC1: It took me " << time_span.count() << " seconds." << std::endl;
// POC2
std::vector<std::unique_ptr<int>> pointers2;
for(int i=0;i<10000;++i) {
pointers2.emplace_back(new int(3));
}
high_resolution_clock::time_point t3 = high_resolution_clock::now();
for(int i=0;i<10000;++i) {
freePointer(std::move(pointers2[i]));
}
high_resolution_clock::time_point t4 = high_resolution_clock::now();
duration<double> time_span2 = duration_cast<duration<double>>(t4 - t3);
std::cout << "POC2: It took me " << time_span2.count() << " seconds." << std::endl;
return 0;
}
在在線編譯器中對其進行測試后,結果顯示 POC2 較慢但結果尚無定論,因為在某些情況下 POC1 較慢。
POC1:我花了 0.000578603 秒。
POC2:我花了 0.00146163 秒。
對於單次運行,您替換順序,首先是 POC2,然后是 POC1,第二個將是最慢的。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.