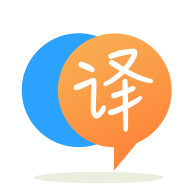
[英]Converting Async/Await function to normal ES5 targetting IE 11
[英]Converting async/await function to ES5 equivalent
我正在重寫一個分批執行 REST API 調用的應用程序,例如一次執行 500 個總調用 10 個。 我需要幫助將使用 ES6+ 函數的 js 函數降級為等效於 ES5 的函數(基本上沒有箭頭函數或 async/await)。
在支持 ES6+ 函數(箭頭函數、async/await 等)的環境中使用的原始應用程序中,我的工作函數如下:
原函數:
// Async function to process rest calls in batches
const searchIssues = async (restCalls, batchSize, loadingText) => {
const restCallsLength = restCalls.length;
var issues = [];
for (let i = 0; i < restCallsLength; i += batchSize) {
//create batch of requests
var requests = restCalls.slice(i, i + batchSize).map((restCall) => {
return fetch(restCall)
.then(function(fieldResponse) {
return fieldResponse.json()
})
.then(d => {
response = d.issues;
//for each issue in respose, push to issues array
response.forEach(issue => {
issue.fields.key = issue.key
issues.push(issue.fields)
});
})
})
// await will force current batch to resolve, then start the next iteration.
await Promise.all(requests)
.catch(e => console.log(`Error in processing batch ${i} - ${e}`)) // Catch the error.
//update loading text
d3.selectAll(".loading-text")
.text(loadingText + ": " + parseInt((i / restCallsLength) * 100) + "%")
}
//loading is done, set to 100%
d3.selectAll(".loading-text")
.text(loadingText + ": 100%")
return issues
}
例如,到目前為止,我編寫的代碼正確地對第一組 10 個中的其余調用進行了批量處理,但我似乎在解析 Promise 時遇到了困難,因此 for 循環可以繼續迭代。
我正在進行的重寫功能:
//Async function process rest calls in batches
function searchIssues(restCalls, batchSize, loadingText) {
const restCallsLength = restCalls.length;
var issues = [];
for (var i = 0; i < restCallsLength; i += batchSize) {
//create batch of requests
var requests = restCalls.slice(i, i + batchSize).map(function(restCall) {
return fetch(restCall)
.then(function(fieldResponse) {
return fieldResponse.json()
})
.then(function(data) {
console.log(data)
response = data.issues;
//for each issue in respose, push to issues array
response.forEach(function(issue) {
issue.fields.key = issue.key
issues.push(issue.fields)
});
})
})
//await will force current batch to resolve, then start the next iteration.
return Promise.resolve().then(function() {
console.log(i)
return Promise.all(requests);
}).then(function() {
d3.selectAll(".loading-text")
.text(loadingText + ": " + parseInt((i / restCallsLength) * 100) + "%")
});
//.catch(e => console.log(`Error in processing batch ${i} - ${e}`)) // Catch the error.
}
//loading is done, set to 100%
d3.selectAll(".loading-text")
.text(loadingText + ": 100%")
return issues
}
我的問題是,一旦我的 10 個 restCalls 完成,我該如何正確解析 Promise 並繼續遍歷 for 循環?
作為參考,我嘗試使用 Babel 編譯原始函數,但它不會在我的 Maven 應用程序中編譯,因此從頭開始重寫。
沒有async/await
,你不能暫停你的for
循環。 但是你可以通過使用遞歸函數來重現這種行為,在每批 10 個之后調用自己。沿着這些路線的東西(未經測試) :
// Async function to process rest calls in batches
function searchIssues(restCalls, batchSize, loadingText) {
var restCallsLength = restCalls.length,
issues = [],
i = 0;
return new Promise(function(resolve, reject) {
(function loop() {
if (i < restCallsLength) {
var requests = restCalls
.slice(i, i + batchSize)
.map(function(restCall) {
return fetch(restCall)
.then(function(fieldResponse) {
return fieldResponse.json();
})
.then(function(d) {
var response = d.issues;
//for each issue in respose, push to issues array
response.forEach(issue => {
issue.fields.key = issue.key;
issues.push(issue.fields);
});
});
});
return Promise.all(requests)
.catch(function(e) {
console.log(`Error in processing batch ${i} - ${e}`);
})
.then(function() {
// No matter if it failed or not, go to next iteration
d3.selectAll(".loading-text").text(
loadingText + ": " + parseInt((i / restCallsLength) * 100) + "%"
);
i += batchSize;
loop();
});
} else {
// loading is done, set to 100%
d3.selectAll(".loading-text").text(loadingText + ": 100%");
resolve(issues); // Resolve the outer promise
}
})();
});
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.