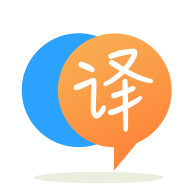
[英]Mockito InvalidUseOfMatchersException, when trying to unit test method with custom Callback as a parameter
[英]Getting an InvalidUseOfMatchersException when stubbing method
我在我的 Android 項目中使用 mockito 來測試將QuerySnapshot
轉換為List<Entry>
的函數。
這是我要測試的功能:
class EntriesMapper {
fun map(querySnapshot: QuerySnapshot): List<Entry> {
return querySnapshot.map { documentSnapshot ->
Entry(documentSnapshot["id"] as String)
}
}
}
這是測試類:
class EntriesMapperTest {
@Test
fun `map should convert query snapshot to entry`() {
val id = 1
val documentSnapshot = mock<DocumentSnapshot> {
on { this.id } doReturn id
}
val querySnapshot = mock<QuerySnapshot>()
val transform = any<(DocumentSnapshot) -> Entry>()
whenever(querySnapshot.map(transform)).thenAnswer { answer ->
(answer.arguments.first() as (DocumentSnapshot) -> Entry).invoke(documentSnapshot)
}
val testObject = EntriesMapper()
val entries = testObject.map(querySnapshot)
val entry = entries.first()
assertThat(entry.id, equalTo(id))
}
}
這是例外:
org.mockito.exceptions.misusing.InvalidUseOfMatchersException:
Invalid use of argument matchers!
0 matchers expected, 1 recorded:
-> at io.company.myapp.entries.EntriesMapperTest.map should convert query snapshot to entry(EntriesMapperTest.kt:147)
This exception may occur if matchers are combined with raw values:
//incorrect:
someMethod(anyObject(), "raw String");
When using matchers, all arguments have to be provided by matchers.
For example:
//correct:
someMethod(anyObject(), eq("String by matcher"));
我知道這是一個非常常見的例外,當您將匹配器與原始值結合使用時,但正如您所看到的,我沒有傳入原始值。
我決定模擬 QuerySnapshot 的原因是它的構造函數是私有的,並且使用 mockito 刪除 map 函數最終會產生比解決方案更多的問題。
QuerySnapshot 繼承自 Iterable,因此我建議使用 List 而不是使用模擬。
class EntriesMapper {
fun map(querySnapshot: Iterable<DocumentSnapshot>): List<Entry> {
return querySnapshot.map { documentSnapshot ->
Entry(documentSnapshot["id"] as String)
}
}
測試班
class EntriesMapperTest {
@Test
fun `map should convert query snapshot to entry`() {
val id = 1
val documentSnapshot = mock<DocumentSnapshot> {
on { this.id } doReturn id
}
val testObject = EntriesMapper()
val entries = testObject.map(listOf(documentSnapshot))
val entry = entries.first()
assertThat(entry.id, equalTo(id))
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.